What is Child Action Calls? How to in ASP.NET MVC 5?
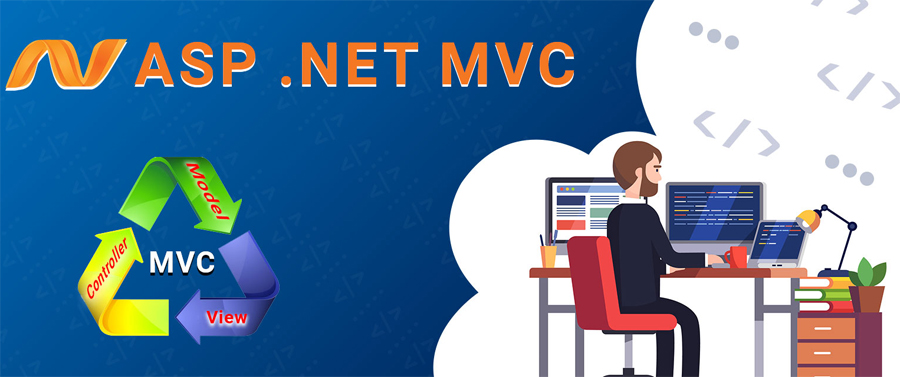
Example Source Code: https://drive.google.com/file/d/1dkgkEq9WR1b1hlDks-pEy8Fx6YBdEEgX/view?usp=sharing
What is Child Action Call?
When we call any action and that action returns view so that action execution will be completed when a view is returned but before completion of view processing if we call any action in between from the view that is called child action call whoes result can be loaded in a view before rendering the original view.
To make the child action call in ASP.NET MVC we have a helper called –
@Html.Action("ActionName",["ControllerName"],[RouteValue Parameters])
Let us understand it step by step how to create it –
Step 1: Create ASP.NET MVC web application as previously created.
Step 2 : Create a action with a name contact which returns a view as follows.
public class TestController : Controller
{
public ActionResult Contact()
{
return View();
}
}
Step 3: Also create two actions called SayHello() and SayBye() which returns string as follows –
public string SayHello()
{
return "Say Hello method Called!";
}
public string SayBye()
{
return "This is Say Bye Action Called!";
}
Step 4: Now call the SayHello and SayBye action using child action call from contact.cshtml view as follows –
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Contact</title>
</head>
<body>
<div>
<h2> Contact view used to demo </h2>
<p> this is contact view used to demo </p>
<h2> Call Say Hello </h2>
@Html.Action("SayHello")
<h2>Call Say Bye </h2>
@Html.Action("SayBye")
</div>
</body>
</html>
You can also return a partial view from action using a method PartialView.
PartialView() : – returns partial view having name exactly similar to action name.
Partialview(model) :- returns a strongly typed partial view having same name as that of action name.
PartialView(“viewname”) : – returns a partial view having a name passed as parameter.
PartialView(“viename”,Model):- returns a strongly typed partial view having a name passed as parameter.
Now create three models to create three strongly typed partial view to be returned from three child action calls as follows –
public class Customer
{
public Int64 CustomerID { get; set; }
public string CustomerName { get; set; }
public string Address { get; set; }
public string EmailID { get; set; }
public decimal CreditLimit { get; set; }
}
public class Emp
{
public Int64 EmpID { get; set; }
public string EmpName { get; set; }
public string DeptName { get; set; }
public decimal Salary { get; set; }
}
public class Product
{
public Int64 ProductID { get; set; }
public string ProductName { get; set; }
public string MfgName { get; set; }
public decimal Price { get; set; }
}
Now create three strongly typed partial view for every model as follows –
_Customre.cshtml
@model PartialViewExampleChildActionCalls.Models.Customer
<table border="1">
<tr>
<td>@Html.LabelFor(p=>p.CustomerID)</td>
<td>@Html.DisplayFor(p=>p.CustomerID)</td>
</tr>
<tr>
<td>@Html.LabelFor(p=>p.CustomerName)</td>
<td>@Html.DisplayFor(p=>p.CustomerName)</td>
</tr>
<tr>
<td>@Html.LabelFor(p=>p.Address)</td>
<td>@Html.DisplayFor(p => p.Address)</td>
</tr>
<tr>
<td>@Html.LabelFor(p=>p.EmailID)</td>
<td>@Html.DisplayFor(p=>p.EmailID)</td>
</tr>
<tr>
<td>@Html.LabelFor(p=>p.CreditLimit)</td>
<td>@Html.DisplayFor(p=>p.CreditLimit)</td>
</tr>
</table>
_Emp.cshtml
@model PartialViewExampleChildActionCalls.Models.Emp
<ol>
<li>Emp ID:@Html.DisplayFor(p => p.EmpID)</li>
<li>Emp ID:@Html.DisplayFor(p => p.EmpName)</li>
<li>Emp ID:@Html.DisplayFor(p => p.DeptName)</li>
<li>Emp ID:@Html.DisplayFor(p => p.Salary)</li>
</ol>
_Product.cshtml
@model PartialViewExampleChildActionCalls.Models.Product
<div style="width:400px;background-color:orange">
Product ID: @Model.ProductID <br />
Product Name: @Model.ProductName <br />
Mfg Name:@Model.MfgName <br />
Price :@Model.Price <br />
</div>
Create three child action actions those returns those partial views from child actions –
public ActionResult GetCust()
{
Customer c = new Customer() {CustomerID=123,CustomerName="Akash",Address="Nigdi",CreditLimit=56000,EmailID="Akash@hotmail.com"};
return PartialView("_Customer",c);
}
public ActionResult GetEmp()
{
Emp e = new Emp() { EmpID = 1, EmpName = "Suresh", DeptName = "Computer", Salary = 56000 };
return PartialView("_Emp", e);
}
public ActionResult GetProduct()
{
Product p = new Product() {ProductID=121,ProductName="Mouse",Price=56000,MfgName="Intex"};
return PartialView("_Product", p);
}
No create the action to get main view where from we can make the child action calls to render these partial views as follows –
public ActionResult CombineData()
{
return View();
}
Now create the CombineData View as follows –
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>CombineData</title>
</head>
<body>
<div>
<h2> Emp information </h2>
@Html.Action("GetEmp")
<hr />
<h2> Customer Information </h2>
@Html.Action("GetCust")
<hr />
<h2> Product information</h2>
@Html.Action("GetProduct")
</div>
</body>
</html>
535 Comments
Comments are closed.
web site
July 7, 2024 at 5:47 amMy spouse and I absolutely love your blog and find many
of your post’s to be exactly what I’m looking for. can you offer guest writers to
write content in your case? I wouldn’t mind producing a post or elaborating on some of the subjects you write about here.
Again, awesome website!
Romeo Newmeyer
September 11, 2024 at 8:26 amWould you be interested in exchanging links?
Leland Ried
September 18, 2024 at 11:01 amSuperb and well-thought-out content! If you need some information about SEO, then have a look at Article World
Warner Gifford
October 1, 2024 at 11:02 amHey there, I love all the points you made on that topic. There is definitely a great deal to know about this subject, and with that said, feel free to visit my blog FQ6 to learn more about Airport Transfer.
Raina Cornejo
October 8, 2024 at 2:58 pmIt is always great to come across a page where the admin take an actual effort to generate a really good article. Check out my website FQ5 concerning about Adsense.
Angel Weyer
October 13, 2024 at 10:46 pmThis website was… how do you say it? Relevant!! Finally I’ve found something that helped me. Thanks.
카지노추천
October 16, 2024 at 4:35 amWay cool! Some extremely valid points! I appreciate you penning this write-up and the rest of the site is also very good.
blue dream
October 16, 2024 at 10:35 amSpot on with this write-up, I really think this web site needs a great deal more attention. I’ll probably be returning to read through more, thanks for the information!
Heraldberg
October 16, 2024 at 1:18 pmWhen I initially commented I appear to have clicked the -Notify me when new comments are added- checkbox and from now on each time a comment is added I receive four emails with the exact same comment. There has to be a means you are able to remove me from that service? Cheers.
Herald America News
October 16, 2024 at 3:53 pmHaving read this I believed it was very enlightening. I appreciate you finding the time and energy to put this content together. I once again find myself personally spending a lot of time both reading and commenting. But so what, it was still worth it!
supplyhouse plumbing
October 16, 2024 at 6:27 pmGreetings, I do think your web site could be having web browser compatibility issues. When I take a look at your site in Safari, it looks fine however, when opening in I.E., it’s got some overlapping issues. I just wanted to give you a quick heads up! Aside from that, great site!
Dispensary Near Me
October 16, 2024 at 8:46 pmI want to to thank you for this excellent read!! I definitely loved every little bit of it. I have you book-marked to check out new things you post…
ücretsiz bonus veren siteler
October 17, 2024 at 12:38 amDeneme bonusu ile oyunlara sıfır yatırımla başlamak hiç bu kadar kazançlı olmamıştı!
daftar slot online
October 17, 2024 at 2:17 amI blog often and I truly thank you for your information. Your article has truly peaked my interest. I am going to take a note of your website and keep checking for new information about once a week. I subscribed to your Feed as well.
additional hints
October 17, 2024 at 7:52 amCasino’da bahis seçeneklerinin çeşitliliği sayesinde her oyunda farklı stratejiler deneyebiliyorum.
toptan poşet baskı
October 17, 2024 at 6:07 pmI was able to find good information from your articles.
인천오피
October 19, 2024 at 9:23 amYou’ve made some really good points there. I checked on the net to learn more about the issue and found most people will go along with your views on this website.
free sex chat
October 19, 2024 at 8:06 pmThis is the right web site for anyone who hopes to find out about this topic. You realize a whole lot its almost hard to argue with you (not that I really would want to…HaHa). You certainly put a brand new spin on a subject that has been written about for a long time. Wonderful stuff, just great.
rulo boru naylon
October 20, 2024 at 3:19 amThere is definately a lot to know about this subject. I love all of the points you have made.
toptan poşet imalatı
October 20, 2024 at 10:25 amI could not resist commenting. Perfectly written!
online dispensary that ships to all states
October 21, 2024 at 1:58 amThere’s definately a great deal to learn about this subject. I like all the points you made.
LAZER 888
October 21, 2024 at 7:14 amAn interesting discussion is worth comment. I do think that you ought to publish more about this issue, it might not be a taboo subject but usually people do not talk about such subjects. To the next! Best wishes!
tubidy
October 21, 2024 at 12:32 pmThis is a topic which is close to my heart… Take care! Exactly where are your contact details though?
슬롯사이트
October 21, 2024 at 11:57 pmHi there! This blog post couldn’t be written any better! Looking at this post reminds me of my previous roommate! He always kept preaching about this. I will send this article to him. Pretty sure he’ll have a very good read. Thank you for sharing!
토토사이트
October 24, 2024 at 3:35 amGreetings! Very helpful advice within this post! It is the little changes that produce the biggest changes. Many thanks for sharing!
online dispensary that ships to all states
October 24, 2024 at 6:07 pmI’m amazed, I must say. Rarely do I come across a blog that’s both equally educative and interesting, and let me tell you, you have hit the nail on the head. The problem is an issue that too few people are speaking intelligently about. I’m very happy that I stumbled across this during my search for something relating to this.
packman v4
October 26, 2024 at 2:34 amGreat info. Lucky me I found your blog by chance (stumbleupon). I have saved it for later.
Download MetaMask
October 26, 2024 at 5:37 amDownload and install MetaMask extension with this beginner guide. Securely set up MetaMask for Ethereum and Web3 applications.
조이슬롯
October 26, 2024 at 9:36 amIt’s nearly impossible to find experienced people on this topic, but you sound like you know what you’re talking about! Thanks
MetaMask Website
October 27, 2024 at 9:32 pmMetaMask stands out as one of the most popular wallet solutions, especially for interacting with Ethereum-based applications. This guide covers everything you need to know about downloading and installing the MetaMask Extension, empowering you to manage your digital assets with ease.
J88
October 27, 2024 at 10:35 pmHi there! This post could not be written much better! Going through this post reminds me of my previous roommate! He always kept talking about this. I’ll forward this article to him. Pretty sure he’ll have a good read. Thank you for sharing!
Kek Tarifi
October 28, 2024 at 5:34 amAmazing experience! Thank you so much for going above and beyond. Will definitely recommend it to my friends!
Revani Tarifi
October 28, 2024 at 6:01 amSuper happy with the service! You guys rock! Thanks for making everything so easy.
Browni Tarifi
October 28, 2024 at 6:47 amFrom start to finish, everything was perfect. Thank you for being so awesome and helpful!
Muhallebi Tarifi
October 28, 2024 at 7:05 amHuge shoutout to the staff for being incredible! Thanks for everything, really appreciate it!
Sütlaç Tarifi
October 28, 2024 at 7:50 amHuge shoutout to the staff for being incredible! Thanks for everything, really appreciate it!
Doğum Günü Mesajları
October 28, 2024 at 9:50 amHuge shoutout to the staff for being incredible! Thanks for everything, really appreciate it!
Günaydın Mesajı
October 28, 2024 at 6:42 pmYou made my day! Thank you for the amazing service – couldn’t be happier!
Burç Özellikleri
October 29, 2024 at 4:23 pmValuable information, thanks!
zeynepkoza39827
October 30, 2024 at 7:52 amVery helpful, thank you!
Metamask Extension
October 30, 2024 at 7:52 amVery helpful, thank you!
Metamask Download
October 30, 2024 at 10:30 pmMuch appreciated!
streamate cam
October 31, 2024 at 1:04 pmI seriously love your site.. Pleasant colors & theme. Did you build this amazing site yourself? Please reply back as I’m looking to create my own blog and want to find out where you got this from or what the theme is named. Many thanks.
Metamask Extension
October 31, 2024 at 3:38 pmSuper helpful, thank you!
메타마스크
October 31, 2024 at 9:59 pmThanks for the inspiration!
tubidy mp3
November 1, 2024 at 5:10 amSpot on with this write-up, I actually feel this web site needs much more attention. I’ll probably be returning to see more, thanks for the information.
metamask
November 1, 2024 at 6:14 amAwesome post, thanks!
MetaMask Extension
November 1, 2024 at 7:26 amThanks for the insights!
Ollie Gigantino
November 1, 2024 at 8:52 pmAfter I originally commented I appear to have clicked on the -Notify me when new comments are added- checkbox and now each time a comment is added I get 4 emails with the exact same comment. Perhaps there is a way you can remove me from that service? Many thanks.
Blue Dream
November 2, 2024 at 2:09 amHello there! This blog post could not be written any better! Going through this post reminds me of my previous roommate! He continually kept talking about this. I most certainly will send this post to him. Pretty sure he’s going to have a great read. I appreciate you for sharing!
MetaMask Wallet
November 2, 2024 at 6:52 amThanks for enlightening us!
Download MetaMask
November 2, 2024 at 6:18 pmAppreciate the share!
ragnarok online servers 2024
November 3, 2024 at 12:52 pmOh my goodness! Impressive article dude! Thank you, However I am going through problems with your RSS. I don’t know why I am unable to join it. Is there anyone else having identical RSS problems? Anyone that knows the answer will you kindly respond? Thanx!!
MetaMask Extension
November 3, 2024 at 4:19 pmThank you so much for your help! I truly appreciate your support and guidance. https://metamask-extension-1059.blogspot.com/2024/11/how-to-download-and-set-up-metamask.html
online dispensary that ships to all states
November 3, 2024 at 7:42 pmI blog frequently and I seriously appreciate your content. This article has truly peaked my interest. I am going to bookmark your site and keep checking for new information about once per week. I opted in for your Feed too.
MetaMask Extension
November 3, 2024 at 11:56 pmNoted. Thanks.
Download MetaMask Extension
November 4, 2024 at 12:10 amThat’ll do. Thanks.
tubidy mp3 download
November 4, 2024 at 2:27 amYou need to be a part of a contest for one of the most useful websites on the net. I am going to recommend this blog!
Download MetaMask Extension
November 4, 2024 at 7:39 amFine, thanks. Moving on.
Download MetaMask Extension
November 4, 2024 at 3:30 pmMuch obliged. Don’t expect a parade.
슬롯플랫폼
November 4, 2024 at 3:41 pmGood post. I am going through a few of these issues as well..
Motherboard
November 5, 2024 at 12:08 amI was pretty pleased to find this site. I wanted to thank you for ones time due to this fantastic read!! I definitely savored every little bit of it and I have you bookmarked to see new things in your website.
Download MetaMask Extension
November 5, 2024 at 2:36 amThat’ll do. Thanks.
Extension
November 5, 2024 at 3:15 amGrateful, but let’s not make a big deal out of it.
gift ideas for him
November 5, 2024 at 6:11 amI blog often and I really thank you for your information. This article has really peaked my interest. I am going to take a note of your website and keep checking for new details about once per week. I opted in for your Feed too.
MetaMask Extension
November 5, 2024 at 9:43 pmThat’ll do. Thanks.
바카라사이트
November 6, 2024 at 2:44 pmMay I just say what a comfort to find someone who truly understands what they’re talking about online. You definitely understand how to bring an issue to light and make it important. More and more people should read this and understand this side of the story. It’s surprising you are not more popular because you certainly possess the gift.
MetaMask Extension
November 7, 2024 at 8:02 amBig thanks for the assistance! You can always find more details at https://docs.extension.support/.
MetaMask Extension
November 7, 2024 at 8:20 pmMuch appreciated, this was exactly what I needed! More at https://download.extension.support/
Sunwin
November 7, 2024 at 8:56 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
sugar defender reviews
November 8, 2024 at 1:43 amAdding Sugar Defender to my everyday routine was one of the most effective decisions I
have actually created my wellness. I’m careful about
what I eat, yet this supplement adds an additional layer of assistance.
I really feel a lot more stable throughout the day, and
my desires have actually decreased substantially.
It behaves to have something so easy that makes such
a big difference!
sugar defender reviews
November 8, 2024 at 1:54 amFor many years, I have actually fought unforeseeable blood sugar swings that left
me really feeling drained and inactive. But because
incorporating Sugar Defender right into my routine, I’ve observed a significant enhancement in my overall energy and stability.
The dreadful mid-day distant memory, and I appreciate that this natural remedy accomplishes these outcomes without any undesirable or unfavorable
reactions. honestly been a transformative discovery for me.
sugar defender ingredients
November 8, 2024 at 1:58 amFor years, I’ve battled unpredictable blood sugar level swings that left me
really feeling drained and lethargic. However since integrating Sugar
my power degrees are currently secure and consistent, and
I no more hit a wall in the afternoons. I value that it’s
a gentle, natural strategy that does not come with any
type of undesirable negative effects. It’s truly transformed my day-to-day live.
Sugar Defender Reviews
November 8, 2024 at 2:00 amSugarcoating Defender to my day-to-day routine was among the
best choices I’ve made for my health and wellness.
I beware about what I eat, however this supplement includes an added layer of support.
I feel a lot more steady throughout the day, and my cravings have actually decreased dramatically.
It behaves to have something so easy that makes
such a large distinction!
sugar defender reviews
November 8, 2024 at 2:07 amAs somebody that’s constantly been cautious regarding my blood
glucose, locating Sugar Defender has actually been a relief.
I really feel so much extra in control, and my recent exams have actually revealed positive enhancements.
Understanding I have a dependable supplement to support my regular provides me satisfaction. I’m so grateful for Sugar
Protector’s effect on my health!
sugar defender reviews
November 8, 2024 at 2:08 amIntegrating Sugar Defender into my daily regimen has been a game-changer for my overall
well-being. As somebody who currently prioritizes healthy and balanced consuming, this supplement has offered an included
boost of defense. in my power levels, and my wish for undesirable snacks so
uncomplicated can have such a profound influence on my life.
8x bet
November 8, 2024 at 7:36 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
tubidy
November 9, 2024 at 3:26 amWay cool! Some extremely valid points! I appreciate you penning this write-up plus the rest of the website is also very good.
MetaMask Extension
November 9, 2024 at 6:17 amThanks. https://sites.google.com/view/metamask-extension-62165/metamask-extension-for-chrome-firefox-and-brave-a-comprehensive-guide
esl lesson plans
November 9, 2024 at 4:18 pmGood information. Lucky me I ran across your website by chance (stumbleupon). I’ve book marked it for later!
MetaMask Extension for Chrome
November 9, 2024 at 7:34 pmGrateful for your interest. Thank you! https://docs.webstore.it.com/
Suncity
November 9, 2024 at 8:22 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
primepickingsplace.shop
November 10, 2024 at 12:45 amHowdy! I simply wish to give you a big thumbs up for your great information you have got here on this post. I will be coming back to your site for more soon.
tubidy
November 10, 2024 at 8:26 pmbookmarked!!, I love your site!
메타마스크
November 11, 2024 at 9:50 amHeartfelt thanks for being here! https://webstore.builders
warm blankets
November 11, 2024 at 8:31 pmHello there! Do you know if they make any plugins to help with
Search Engine Optimization? I’m trying to get my blog to rank for some targeted keywords but
I’m not seeing very good results. If you know of any please share.
Many thanks! You can read similar article here:
Warm blankets
온카족
November 12, 2024 at 1:42 amYou made some decent points there. I looked on the internet for more info about the issue and found most individuals will go along with your views on this website.
PSAT Test Prep
November 12, 2024 at 9:31 amThis blog was… how do you say it? Relevant!! Finally I have found something that helped me. Thanks a lot.
snaptik.icu
November 12, 2024 at 4:23 pmI used to be able to find good info from your blog articles.
Joann Kakn
November 12, 2024 at 5:49 pmWith your post, your readers, particularly those beginners who are trying to explore this field won’t leave your page empty-handed. Here is mine at QN9 I am sure you’ll gain some useful information about Airport Transfer too.
blue dream strain
November 12, 2024 at 8:24 pmThat is a very good tip particularly to those new to the blogosphere. Short but very accurate information… Thank you for sharing this one. A must read post!
supplies still available journal entry
November 13, 2024 at 3:47 amHello there! I could have sworn I’ve been to this web site before but after looking at many of the posts I realized it’s new to me. Anyhow, I’m definitely delighted I found it and I’ll be book-marking it and checking back regularly!
스키강습
November 13, 2024 at 11:12 amI like looking through a post that will make men and women think. Also, thanks for allowing me to comment.
ĐáGà
November 13, 2024 at 11:55 amDaga – Introducing to you the most prestigious online entertainment address today. Visit now to experience now!
moissanite jewelry
November 13, 2024 at 6:52 pmAn intriguing discussion is definitely worth comment. I do think that you need to publish more about this subject matter, it may not be a taboo subject but usually folks don’t speak about these topics. To the next! All the best.
Mood gummies
November 14, 2024 at 3:16 amYou need to take part in a contest for one of the best websites online. I will highly recommend this website!
Raw garden live sauce
November 14, 2024 at 8:40 amYour style is unique in comparison to other folks I’ve read stuff from. Thanks for posting when you have the opportunity, Guess I will just book mark this site.
두정오피
November 14, 2024 at 7:21 pmWay cool! Some extremely valid points! I appreciate you penning this post plus the rest of the website is very good.
Fake id generator
November 15, 2024 at 12:43 amPretty! This has been an incredibly wonderful article. Thanks for providing this information.
Moroccovacation
November 15, 2024 at 6:15 amVery good article. I certainly appreciate this site. Thanks!
Wordle cheats and hints
November 15, 2024 at 11:41 amThe next time I read a blog, I hope that it doesn’t disappoint me as much as this particular one. I mean, Yes, it was my choice to read, however I actually thought you would have something interesting to talk about. All I hear is a bunch of complaining about something that you can fix if you weren’t too busy searching for attention.
Romance Ebooks
November 15, 2024 at 5:38 pmYour style is so unique compared to other people I have read stuff from. I appreciate you for posting when you’ve got the opportunity, Guess I’ll just bookmark this web site.
west ham 3rd kit 21/22
November 15, 2024 at 7:31 pmOnce or twice a century, they could pop up in the southern United States, Mexico and the equatorial areas.
Online Dispensary that ships to all states
November 16, 2024 at 12:53 amI’m amazed, I have to admit. Seldom do I come across a blog that’s equally educative and engaging, and let me tell you, you’ve hit the nail on the head. The problem is an issue that not enough people are speaking intelligently about. I’m very happy that I found this during my search for something concerning this.
CNY Goodies
November 16, 2024 at 3:46 amOh my goodness! Amazing article dude! Many thanks, However I am encountering troubles with your RSS. I don’t understand why I cannot subscribe to it. Is there anybody else getting similar RSS issues? Anyone that knows the answer can you kindly respond? Thanx.
rebirth ro server
November 16, 2024 at 6:37 amIt’s hard to come by educated people about this subject, however, you sound like you know what you’re talking about! Thanks
Régénération cellulaire
November 16, 2024 at 9:30 amPretty! This was an extremely wonderful article. Thanks for supplying this info.
pgslotauto
November 16, 2024 at 12:23 pmGood web site you have here.. It’s hard to find excellent writing like yours nowadays. I truly appreciate individuals like you! Take care!!
120VAC Power for add-on electronics – General Questions – Ubiquity Robotics Discourse
November 16, 2024 at 3:12 pmVery good article. I’m facing a few of these issues as well..
best vpn nordvpn
November 16, 2024 at 6:06 pmAn outstanding share! I have just forwarded this onto a colleague who has been doing a little homework on this. And he in fact bought me dinner simply because I discovered it for him… lol. So let me reword this…. Thanks for the meal!! But yeah, thanks for spending time to discuss this topic here on your website.
슬롯추천검증
November 16, 2024 at 11:28 pmWhen I initially commented I seem to have clicked the -Notify me when new comments are added- checkbox and from now on whenever a comment is added I get 4 emails with the exact same comment. Is there a means you are able to remove me from that service? Thanks a lot.
동대구오피
November 17, 2024 at 4:15 amI couldn’t resist commenting. Perfectly written.
India call girls
November 17, 2024 at 9:58 amVery nice article. I definitely love this site. Keep writing!
Online Dispensary that ships to all states
November 17, 2024 at 12:47 pmA fascinating discussion is definitely worth comment. I do believe that you ought to publish more on this topic, it might not be a taboo matter but typically people don’t discuss such topics. To the next! Cheers.
daga.com
November 17, 2024 at 3:45 pmDaga – Introducing to you the most prestigious online entertainment address today. Visit now to experience now!
Stairlift leeds
November 17, 2024 at 6:30 pmAfter checking out a few of the blog posts on your site, I honestly appreciate your way of writing a blog. I book marked it to my bookmark site list and will be checking back soon. Take a look at my web site as well and let me know how you feel.
MetaMask Extension
November 18, 2024 at 9:43 pmThank you. https://docs.webstore.guru/
how to get clients on linkedin
November 18, 2024 at 11:55 pmYou have made some decent points there. I checked on the internet for more info about the issue and found most people will go along with your views on this website.
porn
November 19, 2024 at 12:39 amThis page truly has all of the info I wanted concerning this subject and didn’t know who to ask.
Casibom
November 19, 2024 at 12:59 amThanks. https://casibom.agency/
Interactive fiction books
November 19, 2024 at 2:48 amEveryone loves it when people come together and share thoughts. Great site, stick with it!
Casibom
November 19, 2024 at 6:37 amThanks. https://casiboms.it.com/
dog puffer jacket
November 19, 2024 at 8:36 amI used to be able to find good advice from your blog articles.
prijs website laten maken
November 19, 2024 at 1:29 pmThe next time I read a blog, I hope that it won’t disappoint me as much as this one. After all, Yes, it was my choice to read, nonetheless I truly thought you’d have something helpful to talk about. All I hear is a bunch of whining about something you could fix if you weren’t too busy searching for attention.
https://gamebaidoithuong.page/blog/game-bai/
November 19, 2024 at 1:45 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
BJ88
November 19, 2024 at 2:28 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
https://sky88.how/
November 19, 2024 at 4:04 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
https://sv88.deals/
November 19, 2024 at 5:07 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
Quay lén trong phòng wc
November 19, 2024 at 6:13 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
mk sports Quay lén phụ nữ
November 19, 2024 at 7:03 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
forex trading signals
November 19, 2024 at 7:32 pmGreetings! Very helpful advice within this post! It’s the little changes that make the most significant changes. Thanks a lot for sharing!
Hiếp dâm bà già
November 19, 2024 at 7:47 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
this site
November 20, 2024 at 2:53 amYou made some good points there. I checked on the internet to find out more about the issue and found most people will go along with your views on this site.
MetaMask Extension
November 20, 2024 at 4:02 amThanks. https://extensions.work/
hoe geld verdienen online
November 20, 2024 at 10:53 pmAw, this was an extremely nice post. Spending some time and actual effort to create a really good article… but what can I say… I hesitate a whole lot and don’t manage to get anything done.
Casibom
November 21, 2024 at 12:31 amThanks. https://casibom.works/
porn
November 21, 2024 at 4:22 amAfter looking at a handful of the blog posts on your site, I seriously appreciate your technique of writing a blog. I saved as a favorite it to my bookmark webpage list and will be checking back soon. Please check out my website as well and let me know how you feel.
MetaMask Extension
November 21, 2024 at 6:26 amMetaMask Extension provides secure wallet integration, dApp connectivity, and seamless access to DeFi platforms. Start exploring Web3 today! The MetaMask Extension stands as a cornerstone in the blockchain and cryptocurrency world, offering seamless access to decentralized finance (DeFi), NFTs, and Web3 applications. https://webstore.work/
jumping castle hire
November 21, 2024 at 10:20 pmMay I simply say what a comfort to uncover someone who actually knows what they’re talking about on the internet. You certainly realize how to bring a problem to light and make it important. More people have to look at this and understand this side of your story. I was surprised that you’re not more popular because you definitely have the gift.
Chainlist
November 22, 2024 at 12:24 amChainlist lets you easily connect to multiple blockchain networks. Simplify wallet setups, save time, and boost your crypto management! https://chainlist.cam/
situs bokep online
November 22, 2024 at 3:38 amAw, this was a very nice post. Taking the time and actual effort to produce a very good article… but what can I say… I hesitate a lot and never seem to get nearly anything done.
gamdom
November 22, 2024 at 8:01 amI love it when individuals get together and share ideas. Great site, stick with it.
sport bet
November 22, 2024 at 10:51 amI quite like reading an article that can make men and women think. Also, thanks for permitting me to comment.
Néon pizza
November 22, 2024 at 4:27 pmI could not refrain from commenting. Well written.
sex video hindi
November 22, 2024 at 4:38 pmThank you so much!
Burç Özellikleri
November 22, 2024 at 9:43 pmBurç özellikleri hakkında merak edilen her şey! Tüm burçların genel ve özel karakteristik özelliklerini keşfetmek için bu rehberi inceleyin. https://tedez.com/burc-ozellikleri/
貼海報
November 22, 2024 at 10:36 pmHaving read this I believed it was extremely informative. I appreciate you finding the time and effort to put this content together. I once again find myself spending a significant amount of time both reading and posting comments. But so what, it was still worth it.
uk chat rooms free: a great way to meet new people
November 23, 2024 at 1:18 amCan I simply say what a relief to uncover somebody who really understands what they’re talking about on the net. You certainly know how to bring an issue to light and make it important. More and more people must look at this and understand this side of the story. It’s surprising you aren’t more popular because you surely have the gift.
MetaMask Extension
November 23, 2024 at 3:41 amDownload MetaMask Extension for Chrome to securely manage your crypto wallet. Follow this easy guide to install and start using MetaMask in minutes! https://sites.google.com/view/download-metamask-extension-41/home
My Online Billboard
November 23, 2024 at 4:03 amI’m amazed, I must say. Rarely do I come across a blog that’s equally educative and interesting, and without a doubt, you’ve hit the nail on the head. The problem is something that not enough men and women are speaking intelligently about. I am very happy I came across this during my hunt for something regarding this.
https://fun88.social
November 23, 2024 at 5:54 amFun88 – Introducing to you the most prestigious online entertainment address today. Visit now to experience now!
casibom nedir
November 23, 2024 at 10:04 pmThank you so much!
MetaMask Extension
November 24, 2024 at 12:48 pmDownload MetaMask extension today for secure crypto wallet access. Follow our step-by-step guide to install it effortlessly on your browser. https://extension.wtf/
MetaMask Extension
November 24, 2024 at 7:48 pmMetaMask is a browser extension and mobile application designed to simplify the interaction between users and blockchain-based applications (dApps). https://sites.google.com/view/metamask-extension-guide-39/home
안전슬롯사이트
November 24, 2024 at 11:33 pmYou’re so interesting! I don’t believe I’ve truly read through something like this before. So wonderful to discover another person with unique thoughts on this issue. Seriously.. many thanks for starting this up. This website is something that is required on the internet, someone with a bit of originality.
silver exchange India
November 25, 2024 at 5:33 amI like it when individuals get together and share ideas. Great website, keep it up.
supergoodsplaza.shop
November 25, 2024 at 8:19 amWonderful post! We are linking to this particularly great content on our site. Keep up the good writing.
Silver Jewellery manufacturer
November 25, 2024 at 11:01 amHello! I simply want to give you a big thumbs up for the excellent info you have right here on this post. I’ll be returning to your site for more soon.
HESED EduSuite
November 25, 2024 at 7:15 pmI’m very happy to uncover this web site. I want to to thank you for your time for this wonderful read!! I definitely liked every part of it and I have you book marked to see new information on your site.
快连
November 26, 2024 at 2:26 amYou need to be a part of a contest for one of the most useful blogs online. I am going to highly recommend this site!
MetaMask Extension
November 26, 2024 at 6:32 pmThanks for checking out this post! Your feedback and engagement make this space special.
porn
November 27, 2024 at 1:35 amAw, this was a really nice post. Finding the time and actual effort to generate a superb article… but what can I say… I hesitate a lot and don’t seem to get nearly anything done.
gay porn
November 27, 2024 at 12:03 pmYou should be a part of a contest for one of the most useful blogs online. I am going to highly recommend this web site!
porn
November 28, 2024 at 12:58 amHi! I simply want to give you a huge thumbs up for the excellent info you have got here on this post. I am coming back to your web site for more soon.
Telegram官网
November 29, 2024 at 2:27 amYou made some decent points there. I checked on the internet for more information about the issue and found most individuals will go along with your views on this web site.
Level Transmitter
November 29, 2024 at 7:42 amWay cool! Some very valid points! I appreciate you writing this article and the rest of the site is extremely good.
peelerie.com
November 29, 2024 at 4:47 pmThis blog was… how do you say it? Relevant!! Finally I’ve found something that helped me. Thank you.
porn
November 30, 2024 at 1:43 amIt’s nearly impossible to find knowledgeable people about this subject, but you sound like you know what you’re talking about! Thanks
read more
December 1, 2024 at 1:07 amCan I simply say what a relief to find somebody that actually understands what they are talking about on the web. You definitely realize how to bring a problem to light and make it important. More and more people should check this out and understand this side of the story. I was surprised that you aren’t more popular given that you certainly possess the gift.
분당오피
December 1, 2024 at 4:07 amIt’s hard to come by experienced people on this subject, but you seem like you know what you’re talking about! Thanks
mythemeshop
December 1, 2024 at 10:28 amVery good information. Lucky me I found your website by chance (stumbleupon). I have bookmarked it for later.
straight from the source
December 2, 2024 at 2:28 amA fascinating discussion is worth comment. There’s no doubt that that you ought to publish more on this subject, it might not be a taboo subject but generally folks don’t speak about such topics. To the next! Cheers.
porn
December 2, 2024 at 5:25 amI was able to find good info from your blog articles.
y2mate
December 2, 2024 at 7:48 amPretty! This was an extremely wonderful article. Thank you for providing this info.
porn
December 3, 2024 at 1:03 amCan I just say what a relief to find a person that really knows what they are talking about online. You definitely realize how to bring an issue to light and make it important. A lot more people really need to look at this and understand this side of the story. I was surprised that you’re not more popular given that you most certainly have the gift.
investigate this site
December 3, 2024 at 4:50 amYou made some really good points there. I checked on the internet to learn more about the issue and found most people will go along with your views on this website.
porn
December 3, 2024 at 4:17 pmEveryone loves it when individuals get together and share thoughts. Great blog, stick with it.
porn
December 3, 2024 at 9:18 pmVery good post. I absolutely appreciate this website. Stick with it!
Insomnia Treatment Singapore
December 3, 2024 at 9:22 pmNext time I read a blog, I hope that it does not disappoint me just as much as this particular one. After all, Yes, it was my choice to read, nonetheless I really thought you’d have something helpful to say. All I hear is a bunch of crying about something you could possibly fix if you were not too busy seeking attention.
porn
December 4, 2024 at 2:18 amGood day! I simply wish to give you a big thumbs up for the great info you have got here on this post. I am coming back to your blog for more soon.
Slottica PL
December 4, 2024 at 8:49 amGood day! I could have sworn I’ve visited this site before but after looking at a few of the posts I realized it’s new to me. Anyways, I’m definitely delighted I discovered it and I’ll be bookmarking it and checking back often.
blog link
December 4, 2024 at 3:06 pmGreat web site you have got here.. It’s hard to find high-quality writing like yours these days. I honestly appreciate individuals like you! Take care!!
pandora jewelry
December 4, 2024 at 3:59 pmAw, this was a really good post. Taking the time and actual effort to create a very good article… but what can I say… I put things off a lot and never seem to get nearly anything done.
파라존카지노
December 4, 2024 at 10:43 pmMay I just say what a comfort to find a person that genuinely knows what they are talking about online. You actually realize how to bring a problem to light and make it important. More people ought to read this and understand this side of the story. I was surprised that you are not more popular because you definitely have the gift.
라카지노 사이트
December 5, 2024 at 5:54 amAfter I originally commented I seem to have clicked on the -Notify me when new comments are added- checkbox and now every time a comment is added I get four emails with the exact same comment. Perhaps there is a way you are able to remove me from that service? Thanks.
what is the best news
December 5, 2024 at 12:59 pmThis is a topic that’s near to my heart… Take care! Where are your contact details though?
click now
December 5, 2024 at 4:38 pmI used to be able to find good info from your blog posts.
see here
December 5, 2024 at 9:00 pmI could not refrain from commenting. Exceptionally well written.
look here
December 6, 2024 at 1:20 amPretty! This was an extremely wonderful post. Many thanks for providing this info.
fast and hard porn
December 6, 2024 at 9:23 amThere is certainly a lot to know about this topic. I really like all of the points you’ve made.
Is This Finally Our Chance to Find Jobs We Love?
December 6, 2024 at 10:45 amPretty! This was an incredibly wonderful article. Thank you for providing this information.
femboy interracial porn
December 6, 2024 at 2:08 pmI’m very happy to discover this site. I want to to thank you for your time due to this fantastic read!! I definitely savored every bit of it and I have you saved as a favorite to look at new information on your site.
porn in 15 seconds
December 6, 2024 at 7:11 pmThat is a very good tip especially to those fresh to the blogosphere. Brief but very precise information… Thank you for sharing this one. A must read post!
https://dgo88.net/
December 6, 2024 at 7:48 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
hardcore crying porn
December 7, 2024 at 12:14 amYou’re so awesome! I do not suppose I’ve read through anything like that before. So great to find someone with genuine thoughts on this subject matter. Seriously.. many thanks for starting this up. This site is one thing that is needed on the internet, someone with a bit of originality.
Go88
December 7, 2024 at 2:07 amIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
kim possible porn gif
December 7, 2024 at 5:17 amI used to be able to find good information from your blog posts.
パン屋粗利益率
December 7, 2024 at 8:36 amBut the whole idea of philosophy is to place an idea of worth into the universe, which appertains to humans, and by extension to all life types.
売り掛け債権
December 7, 2024 at 9:53 amJust like the Pro it comes with two totally different sizes, 14″ and 15.6″.
札幌手稲スキー場天気
December 7, 2024 at 10:09 amCWT is a business-to-enterprise-for-workers travel administration platform.
https://iwin20.com
December 7, 2024 at 10:28 amIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
mickey blue porn
December 7, 2024 at 10:29 amVery good information. Lucky me I recently found your site by chance (stumbleupon). I’ve bookmarked it for later!
https://iwin33.com
December 7, 2024 at 1:54 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
billie eilish blowjob porn
December 7, 2024 at 3:45 pmGreat web site you have here.. It’s difficult to find high quality writing like yours nowadays. I honestly appreciate people like you! Take care!!
iwin club
December 7, 2024 at 5:43 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
katrina thicc porn
December 7, 2024 at 8:46 pmWonderful post! We are linking to this particularly great content on our website. Keep up the great writing.
iwin club
December 7, 2024 at 10:39 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
iwinclub
December 8, 2024 at 2:00 amIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
iwin club
December 8, 2024 at 11:56 amIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
iwinclub
December 8, 2024 at 1:27 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
furry porn site
December 8, 2024 at 2:29 pmI really love your blog.. Great colors & theme. Did you develop this website yourself? Please reply back as I’m looking to create my own blog and would like to learn where you got this from or exactly what the theme is called. Thank you!
pandoranyxie porn
December 8, 2024 at 8:18 pmYou need to be a part of a contest for one of the most useful websites on the web. I’m going to recommend this site!
porn 17
December 9, 2024 at 1:54 amGood info. Lucky me I came across your site by accident (stumbleupon). I have saved as a favorite for later!
sugar defender
December 9, 2024 at 12:23 pmsugar defender I’ve had problem with blood sugar level fluctuations for many
years, and it actually influenced my energy levels throughout the day.
Because beginning Sugar Defender, I really feel much more
well balanced and sharp, and I do not experience
those afternoon slumps any longer! I like that
it’s an all-natural solution that works with no rough side effects.
It’s genuinely been a game-changer for me
anna.caarter onlyfans porn
December 9, 2024 at 4:47 pmThat is a very good tip especially to those fresh to the blogosphere. Brief but very accurate information… Thanks for sharing this one. A must read post.
redbox porn
December 9, 2024 at 11:19 pmThis site was… how do you say it? Relevant!! Finally I have found something that helped me. Thanks!
seattle dad porn
December 10, 2024 at 5:45 amI must thank you for the efforts you have put in writing this site. I am hoping to see the same high-grade blog posts by you in the future as well. In truth, your creative writing abilities has inspired me to get my own, personal blog now 😉
top porn cites
December 10, 2024 at 12:15 pmThis is the perfect web site for everyone who wishes to understand this topic. You understand a whole lot its almost hard to argue with you (not that I actually will need to…HaHa). You definitely put a new spin on a topic which has been written about for a long time. Great stuff, just excellent.
MetaMask Extension
December 10, 2024 at 12:40 pmThank you for breaking this down so clearly!
milf porn tubes
December 10, 2024 at 6:43 pmGood site you have here.. It’s difficult to find high-quality writing like yours nowadays. I seriously appreciate individuals like you! Take care!!
gia porn
December 11, 2024 at 1:01 amSaved as a favorite, I like your blog!
irene silver porn
December 11, 2024 at 7:36 amWonderful post! We are linking to this particularly great article on our site. Keep up the good writing.
here
December 11, 2024 at 8:24 amCan I simply just say what a comfort to find somebody who genuinely understands what they are discussing on the net. You definitely realize how to bring an issue to light and make it important. A lot more people ought to read this and understand this side of the story. I was surprised that you are not more popular since you certainly possess the gift.
my company
December 12, 2024 at 6:37 amGreetings! Very helpful advice within this post! It’s the little changes which will make the most significant changes. Thanks a lot for sharing!
ブラジル暮らし
December 12, 2024 at 9:43 amSince 1996, over 300 employers have received the freedom Award.
MetaMask Extension
December 12, 2024 at 11:42 pmThank you for sharing such valuable insights!
国旗 トルコ
December 13, 2024 at 3:13 amA typical story pertains to an incident that occurred at the tournament banquet, when the St.
火 音読み 訓読み
December 13, 2024 at 11:08 pmBuilding directions (plugs and wires only) is supplied with the sport House Balls (known as Star Balls in its schematics web page).
MetaMask Extension
December 14, 2024 at 1:05 pmI never thought of it this way before. Very eye-opening—great job! https://metasmak.com/
100 pure cotton bedsheets
December 15, 2024 at 12:42 pmI was very happy to find this page. I need to to thank you for your time just for this fantastic read!! I definitely loved every bit of it and I have you book marked to check out new stuff in your blog.
necネッツエスアイ 配当
December 15, 2024 at 1:30 pmAlong with one or two favourite pieces — the mattress, say, and a chest you’ve got had since childhood — odds and ends accumulate, such as a rocker that will not do within the living room or an outdated portrait.
糊口をしのぐ 由来
December 15, 2024 at 6:05 pmCharles County, Missouri, to the north of Route ninety four at 7:32 p.m.
Best Book Analytical Reasoning
December 15, 2024 at 7:02 pmWonderful beat ! I would like to apprentice while you amend your site, how could i subscribe for a blog site? The account helped me a acceptable deal. LSAT Analytical Reasoning I had been tiny bit acquainted of this your broadcast offered bright clear concept.
scaffolding companies near me
December 15, 2024 at 9:02 pmI tend to some what disagree with what you said but in hindsight I don’t follow the subject very well. You might have a much better grasp than me.
snaptik
December 15, 2024 at 9:08 pmI couldn’t refrain from commenting. Very well written!
rolling scaffold
December 16, 2024 at 8:38 amWhoa. That was a great article. Please keep writing because I love your style.
6m scaffold tower
December 16, 2024 at 12:06 pmWe’ve to say, I take pleasure in reading this web page. Maybe you could let me know how I can subscribing with it.
tubidy mp3 download
December 16, 2024 at 1:42 pmEverything is very open with a very clear clarification of the issues. It was definitely informative. Your site is very helpful. Thanks for sharing!
portable scaffold tower
December 16, 2024 at 3:38 pmI like your writing style genuinely loving this internet site .
used scaffold towers for sale near me
December 17, 2024 at 7:52 amMerci à vous pour cette information. C’est toujours important de savoir ce genre d’avis.
aluminium scaffolding for sale
December 17, 2024 at 8:37 amJust lately, I didnrrrt offer lots of consideration for you to departing answers on-page page accounts and still have positioned replies actually much less. Examining by using your enjoyable article, can assist myself to do this sometimes.
scaffolding
December 17, 2024 at 8:52 amCan you please add Google Reader compatibility? It’s not able to recognize your RSS feed. You can email me if you need help in getting it to work.
scaffolding cost
December 17, 2024 at 12:22 pmI’d forever want to be update on new posts on this web site , saved to my bookmarks ! .
second hand scaffolding for sale
December 17, 2024 at 1:24 pmOh my goodness! an amazing post dude. Thank you Nevertheless We are experiencing issue with ur rss . Do not know why Cannot register for it. Is there any person obtaining identical rss dilemma? Anyone who knows kindly respond. Thnkx
adjustable scaffolding
December 17, 2024 at 1:40 pmI gotta favorite this site it seems extremely helpful handy
mi tower scaffold
December 17, 2024 at 3:51 pmpregancy is quite critical and also requires more of your time so that you can monitor your health progress“
downloader instagram
December 17, 2024 at 5:50 pmI need to to thank you for this very good read!! I certainly loved every bit of it. I have you book-marked to check out new stuff you post…
rolling scaffold
December 17, 2024 at 5:58 pmThank you of this blog. That’s all I’m able to say. You definitely have made this web site into an item thats attention opening in addition to important. You definitely know a great deal of about the niche, youve covered a multitude of bases. Great stuff from this the main internet. All over again, thank you for the blog.
mobile scaffold tower
December 17, 2024 at 6:14 pmYou’re the best, I was doing a google search and your site came up for foreclosures in Oviedo, FL but anyway, I have thoroughly enjoyed reading it, keep it up!
scaffolding contractors near me
December 17, 2024 at 7:21 pmThank you for this kind of information I was looking around all Yahoo to discover it!
portable scaffold tower
December 17, 2024 at 9:24 pmMusically, the film has majority of its songs written partly in hip hop which have a limited appeal.
portable scaffolding
December 17, 2024 at 9:42 pmI was recommended this web site by my cousin. I’m now not positive whether or not this publish is written by him as no one else understand such targeted approximately my difficulty. You are wonderful! Thank you!
MetaMask Download
December 18, 2024 at 1:07 amThis was really helpful. Thanks! https://metadocs.org
home scaffolding
December 18, 2024 at 7:43 amyou should always be careful with recruitment agencies because some of them are just scammers;;
mini scaffold
December 18, 2024 at 7:52 amI admit, I have not been on this webpage in a long time… however it was one more joy to see It is such an significant topic and also ignored by so many, even professionals. I thank you to support making people more aware of possible issues.
aluminium scaffolding
December 18, 2024 at 7:59 amYoure so cool! I dont suppose Ive read something such as this before. So nice to search out somebody with authentic applying for grants this subject. realy i appreciate you for starting this up. this fabulous website are some things that’s needed on the internet, somebody after some bit originality. helpful purpose of bringing new things to your web!
youtubetomp3
December 18, 2024 at 9:30 amGood post. I learn something new and challenging on sites I stumbleupon on a daily basis. It’s always exciting to read articles from other writers and practice a little something from their websites.
kwikstage scaffolding
December 18, 2024 at 11:29 amThere are some fascinating points with time here but I do not know if all of them center to heart. You can find some validity but I am going to take hold opinion until I consider it further. Very good post , thanks so we want much more! Added to FeedBurner as well
lewis scaffold tower
December 18, 2024 at 11:43 amEmployee relations should be given more importance in an office environment as well as on any other business establishment..
aluminium scaffold tower
December 18, 2024 at 11:59 amThis would be the correct weblog for everyone who is wishes to be familiar with this topic. You already know much its nearly difficult to argue with you (not that I really would want…HaHa). You certainly put a new spin for a topic thats been revealed for several years. Wonderful stuff, just great!
kwikstage scaffold for sale
December 18, 2024 at 3:00 pmnice post, keep up with this interesting work. It really is good to know that this topic is being covered also on this web site so cheers for taking time to discuss this!
mobile scaffold tower
December 18, 2024 at 3:09 pmAppreciate it for this marvelous post, I am glad I observed this website on yahoo.
scaffolding contractors
December 18, 2024 at 3:59 pmAw, this was a very nice post. In thought I want to put in writing like this additionally ?taking time and actual effort to make an excellent article?however what can I say?I procrastinate alot and under no circumstances seem to get one thing done.
interior scaffolding
December 18, 2024 at 8:23 pmi love to also get some beach body but it requires a lot of diet modification and exercise~
aluminium scaffolding
December 18, 2024 at 8:26 pmThanks i love your article about Life is Priceless | Fr. Frank Pavone’s Blog
types of scaffolding
December 18, 2024 at 8:39 pmvery good post, i surely enjoy this web site, persist with it
パナソニック 志望動機
December 18, 2024 at 10:27 pmThe latter have merely enthroned quantum events on the seat of God, the place their forerunners had positioned first Freudian, later Skinnerian psychology and ultimately genes, every with as little transparency as the burning bush itself; although trumpeting forth with the identical excessive pretensions as the dormitory precept and cognate forms of humbug.
固定資産税 減税申請
December 19, 2024 at 1:38 amThe Monkey’s Bum first appeared in print 5 years later within the British Chess Magazine.
my site
December 19, 2024 at 2:24 amHowdy, I think your blog could be having web browser compatibility problems. Whenever I take a look at your blog in Safari, it looks fine however when opening in Internet Explorer, it has some overlapping issues. I just wanted to provide you with a quick heads up! Apart from that, great website.
youngman scaffold tower
December 19, 2024 at 8:31 amThere are a couple of interesting points over time here but I do not determine if these people center to heart. You can find some validity but I most certainly will take hold opinion until I take a look at it further. Excellent article , thanks therefore we want far more! Combined with FeedBurner also
scaffolding for sale near me
December 19, 2024 at 8:35 amI’m not sure where you’re getting your info, but great topic. I needs to spend some time learning much more or understanding more.
mi tower
December 19, 2024 at 8:48 amI couldn’t resist writing comments. “All successful people men and women are big dreamers. They imagine what their future could be, ideal in every respect, and then they work every day toward their distant vision, that goal or purpose.” by Brian Tracy..
cuplock scaffolding
December 19, 2024 at 12:29 pmHi, It’s hard to find knowledgeable people on this topic, but you sound like you know what you’re talking about! Thanks
used scaffolding for sale near me
December 19, 2024 at 12:36 pmWow that was odd. I just wrote an incredibly long comment but after I clicked submit my comment didn’t show up. Duch Œwiêty Grrrr… well I’m not writing all that over again. Anyways, just wanted to say fantastic blog!
scaffolding equipment
December 19, 2024 at 12:44 pmA few things i have seen in terms of laptop or computer memory is that often there are features such as SDRAM, DDR etc, that must fit in with the specs of the mother board. If the personal computer’s motherboard is reasonably current while there are no operating-system issues, improving the memory literally will take under a couple of hours. It’s one of the easiest computer upgrade types of procedures one can envision. Thanks for sharing your ideas.
b&q scaffold tower
December 19, 2024 at 3:53 pmThe writers simply did not give the characters an opportunity to display the complexity of the relationship.
scaffolder
December 19, 2024 at 4:01 pmAn impressive share, I merely given this onto a colleague who was doing a little analysis for this. Anf the husband in truth bought me breakfast simply because I found it for him.. smile. So i want to reword that: Thnx for your treat! But yeah Thnkx for spending plenty of time to talk about this, I feel strongly regarding this and really like reading regarding this topic. If it is possible, as you grow expertise, can you mind updating your blog site with a lot more details? It’s highly useful for me. Huge thumb up with this writing!
5m scaffold tower
December 19, 2024 at 4:12 pmThanks for the sensible critique. Me & my neighbor were just preparing to do some research on this. We got a grab a book from our local library but I think I learned more from this post. I’m very glad to see such fantastic info being shared freely out there.
左折可 多い県
December 19, 2024 at 6:47 pmNature and weather situations can harm tourism; having a mild but warm summer temperature attracts vacationers from many locales, but storms and forest fires in its local weather generally is a detraction.
bps scaffold tower
December 19, 2024 at 7:47 pmI believe this site holds very good pent subject material posts .
hop over to here
December 19, 2024 at 8:03 pmSaved as a favorite, I like your website.
西宮市ホームページ
December 19, 2024 at 11:17 pmEnterprise journey, convention or leisure trips, no matter the reason being, in case you are bound to London, you have to name on a vacation spot management firm to get professional help in finding out the best hotel or location.
投資セミナー講師
December 20, 2024 at 3:58 amVery primary laptop programs can do it too, these days if you copy paste random strings to google translate it is going to recognise languages form of.
used scaffolding for sale
December 20, 2024 at 8:06 amAfter a brief firefight that results in the hood of Highsmith’s car getting blown into the windshield, the two inadvertently crash into the side of a double decker bus.
access scaffolding
December 20, 2024 at 8:10 amsome tanning salons have high power uv lamps which can shorten the amount of time you need to expose yourself::
construction scaffolding
December 20, 2024 at 8:23 amThis blog is disseminating valuable information to people who are most concerned of the following issues being targeted by this site. Many certainly will keep coming back to check out updated posts.
second hand scaffolding for sale
December 20, 2024 at 1:30 pmYou were quite interesting.. But sadly I didnrrrt trust them much :/ Although I might disagree I still give you support as how confident you are on your writing lol
wickes scaffold tower
December 20, 2024 at 1:33 pmgood read,found more information available HERE for anyone who is interested it really helped more a more in depth look, good site btw
rent scaffolding near me
December 20, 2024 at 1:46 pmyou have a great blog here! would you like to make some invite posts on my weblog?
gas safety
December 21, 2024 at 7:52 amHi, I just found your blog via google. Your viewpoint is truly applicable to my life right now, and I’m really pleased I discovered your website.
gas engineer professional development
December 21, 2024 at 8:07 amSome times its a pain in the ass to read what people wrote but this site is really user friendly!
gas system retrofitting
December 21, 2024 at 11:21 amWow! This can be one particular of the most useful blogs We’ve ever arrive across on this subject. Basically Excellent. I’m also an expert in this topic therefore I can understand your effort.
gas engineer apprenticeships
December 21, 2024 at 12:14 pmThe tips you provided here are extremely precious. It turned out this sort of pleasurable surprise to acquire that watching for me after i awakened today. There’re constantly to the point and to comprehend. Thanks a ton to the valuable ideas you’ve got shared here.
gas appliance repairs
December 21, 2024 at 4:48 pmI see something genuinely special in this website .
boiler maintenance
December 21, 2024 at 5:03 pmmost of the best ringtone sites are pay sites, does anyone know of a good free ringtone site?,
神経質言い換え面接
December 21, 2024 at 8:02 pmCDs, or certificates of deposit, are savings vehicles insured by the Federal Deposit Insurance Corporation (FDIC).
Marcosew
December 21, 2024 at 8:58 pmblog here phantom wallet
DamienWaP
December 21, 2024 at 9:08 pmadvice phantom Download
Danieltex
December 21, 2024 at 9:23 pmtop article phantom Download
AlfonsoPetry
December 21, 2024 at 9:42 pmpop over to this web-site phantom wallet
Shermannex
December 21, 2024 at 9:53 pmClicking Here keplr Extension
Arthuraccig
December 21, 2024 at 11:17 pmbrowse around this site MetaMask Download
EdwardNak
December 21, 2024 at 11:22 pmuseful content phantom wallet
JosephBoume
December 21, 2024 at 11:23 pmwhy not try these out Metamask Extension
AnthonyFraps
December 21, 2024 at 11:25 pmfind out phantom Download
今日の天気横須賀市
December 22, 2024 at 3:26 amIn January 1741, some 3,255 officers and men of Gooch’s “American Regiment” were on board ship within the harbor of Kingston, Jamaica.
神戸ymca キャンプ
December 23, 2024 at 1:30 amOn June 26, 12 more counties entered the green part: Berks, Bucks, Chester, Delaware, Erie, Lackawanna, Lancaster, Lehigh, Montgomery, Northampton, Philadelphia and Susquehanna.
女性 入院 保険
December 23, 2024 at 2:04 amThe Sullivan column would take River Highway from Bear Tavern to Trenton while Washington’s column would follow Pennington Street, a parallel route that lay a couple of miles inland from the Delaware River.
借金 金利 安い
December 23, 2024 at 7:03 amThey were in search of a real salvage yard, any person with a bit of bit of all the things.
AlvinTom
December 24, 2024 at 1:43 amLook At This MetaMask Download
Thomasfeesy
December 24, 2024 at 1:43 amread the article MetaMask Download
Fosterrak
December 24, 2024 at 2:11 amher explanation phantom wallet
WilliamOpedo
December 24, 2024 at 2:26 amlinked here MetaMask Download
Albertsen
December 24, 2024 at 2:27 amhelpful site phantom Download
Brandontew
December 24, 2024 at 2:37 amnews phantom Download
JamesinemE
December 24, 2024 at 2:40 amclick to read more phantom Download
Howardovaky
December 24, 2024 at 3:01 amvisit the website phantom Extension
HaroldBog
December 24, 2024 at 3:02 amhave a peek at this website keplr wallet
ThomasBrAnd
December 24, 2024 at 3:43 amher response rabby wallet extension
Ramonmog
December 24, 2024 at 3:59 ammore keplr wallet
see this website
December 24, 2024 at 9:58 amI blog often and I genuinely appreciate your content. This article has really peaked my interest. I’m going to take a note of your blog and keep checking for new information about once a week. I subscribed to your RSS feed too.
Kym Muncrief
December 24, 2024 at 2:50 pmI have learn some good stuff here. Definitely price bookmarking for revisiting. I wonder how a lot attempt you set to create one of these wonderful informative website.
more hints
December 24, 2024 at 4:47 pmHi, I do believe this is a great blog. I stumbledupon it 😉 I am going to return once again since i have bookmarked it. Money and freedom is the best way to change, may you be rich and continue to help other people.
find out here now
December 24, 2024 at 11:35 pmI could not resist commenting. Exceptionally well written!
click this over here now
December 25, 2024 at 6:15 amYou are so awesome! I do not believe I’ve read through a single thing like that before. So great to find another person with genuine thoughts on this issue. Really.. thanks for starting this up. This website is one thing that is needed on the web, someone with some originality.
redirected here
December 25, 2024 at 1:06 pmThe very next time I read a blog, I hope that it does not fail me as much as this one. I mean, Yes, it was my choice to read through, however I really believed you would have something interesting to talk about. All I hear is a bunch of moaning about something you can fix if you were not too busy looking for attention.
home
December 25, 2024 at 8:22 pmHi, I think your site may be having internet browser compatibility issues. Whenever I look at your blog in Safari, it looks fine however when opening in Internet Explorer, it’s got some overlapping issues. I simply wanted to give you a quick heads up! Besides that, wonderful website!
have a peek here
December 26, 2024 at 3:44 amHello! I could have sworn I’ve visited this blog before but after going through many of the posts I realized it’s new to me. Nonetheless, I’m certainly delighted I discovered it and I’ll be bookmarking it and checking back often!
visit this website
December 26, 2024 at 11:05 amGreetings, I do think your blog could possibly be having browser compatibility problems. Whenever I take a look at your blog in Safari, it looks fine however when opening in I.E., it’s got some overlapping issues. I merely wanted to give you a quick heads up! Apart from that, excellent blog!
現実 逃避 妄想 病気
December 27, 2024 at 4:34 amIf the right company to hitch personal fairness agency, which is an excellent ebook, decent workers and a community, your job is done.
starzcotedivoire
December 27, 2024 at 6:53 amProfitez d’une plateforme fiable avec 888starz bookmaker.
Cesarsnark
December 28, 2024 at 1:59 pmCrypto scam – coin drain, best crypto drainer
BarryBaics
December 28, 2024 at 2:08 pmчитать https://forum.hpc.name/thread/2/65364/polnoe-shifrovanie-diskov-v-freebsd-zagruzka-yadra-s-fleshki.html
RandallNeogE
December 29, 2024 at 2:15 pmabout his Twitter Provider
Augustnuh
December 29, 2024 at 4:25 pmpublished here Immediate Affinity
GeorgeNenry
December 29, 2024 at 5:16 pmзеленый мир маркетплейс – zmir вход, зеленый мир даркнет
RobertUnlal
December 29, 2024 at 5:27 pmнова darknet ссылка – нова дарк, нова рабочее зеркало
Edwarddix
December 29, 2024 at 6:20 pmjaxx io – jaxx app, jaxx io
Kennethreumn
December 29, 2024 at 6:23 pmtry here https://jaxx-liberty.com/
MetaMask Extension
December 30, 2024 at 1:25 amThanks for stopping by! Don’t forget to explore https://metakit.org/
借地権売買 相場
December 30, 2024 at 2:58 amHow many species of wild cats exist on this planet in the present day?
pkwin
December 30, 2024 at 1:46 pmThank for share! Play PKWIN at https://pkwin8386.com
starzkz
December 31, 2024 at 1:47 amAndroid ?шін 888starz ?осымшасын бірден алы?ыз.
MetaMask Download
December 31, 2024 at 2:37 amThanks for another great post! Here’s something related you might enjoy: https://metaium.org/
Kacy Martt
December 31, 2024 at 7:08 amI’m still learning from you, but I’m improving myself. I definitely liked reading everything that is posted on your blog.Keep the information coming. I liked it!
house painting
December 31, 2024 at 8:21 amI request more people would write sites like this that are as a matter of fact constructive to read. With all the fluff floating almost on the web, it is rare to look over a position like yours instead.
pg88
December 31, 2024 at 9:55 pmThank for share! Play at https://nhacaipg88.com to win
telegram @seo_linkk
January 1, 2025 at 3:35 pmAfter checking out a number of the articles on your web site, I honestly like your way of writing a blog. I saved it to my bookmark website list and will be checking back soon. Please visit my website too and let me know your opinion.
Snaptik
January 3, 2025 at 2:45 amYou made some decent points there. I checked on the internet to find out more about the issue and found most people will go along with your views on this website.
ゴルフ 紫外線対策
January 3, 2025 at 7:53 amSlicing and assembling paper shapes and colors into delicate photographs fascinates me, whether utilizing vintage or modern papers.
20 ユーロ 日本 円
January 3, 2025 at 8:00 amEver-increasing budgets are now allocated to search marketing, and a new era of Internet growth has been established – the era of Inbound Marketing.
山口 県 中部 天気 予報
January 3, 2025 at 12:25 pmHesselius would, later, play an indirect position in the portraiture of George Washington, when he took on a younger apprentice saddlemaker who aspired to turn out to be a painter.
MetaMask Extension
January 3, 2025 at 3:39 pmLoved this perspective! Check out https://metaink.org for more.
pg88
January 3, 2025 at 4:20 pmThank for share! Play at https://kccommand.com to win
無料 掲示板 埋め込み
January 4, 2025 at 12:13 amForeign direct investment is distinguished from foreign portfolio investment, a passive investment in the securities of another country such as public stocks and bonds, by the element of “control”.
めいめい 英語
January 4, 2025 at 5:47 amPVM, who enquired about the early-morning trades.
薄いグレー カラーコード
January 4, 2025 at 10:02 amCustomers admire the worth of the pot holders.
starzbaza
January 4, 2025 at 10:36 pmhttps://onlinetri.com/common/pgs/888starz-site-officiel_1.html 888starz
high-quality residential painting
January 5, 2025 at 8:54 amYou need to get upset! Really its a must to take a look past everything and get upset. Generally this will allow you to take the inititive to make things happen.
Metaback
January 5, 2025 at 10:06 amExcellent content! For those interested, https://metaback.org/ has similar topics.
professional painting services
January 5, 2025 at 10:13 pmI like the valuable information you offer for your articles. I will be able to bookmark your blog and feature my kids check up here generally. I am somewhat certain they are going to be informed numerous new stuff right here than anyone else!
exterior painting
January 5, 2025 at 11:32 pmTo tell you the teuth, I was passing around and come across your site. It is wonderful. I mean as a content and design. I added you to my list and decided to spent the rest of the weekend browsing. Well done!
starzUZB
January 6, 2025 at 1:44 am888starz platformasi haqida ma’lumot olish uchun 888starz rasmiy ilovasini yuklab oling va xizmatlardan to‘liq foydalaning. Ushbu dastur sport stavkalari va kazino o‘yinlarini amalga oshirish uchun eng yaxshi tanlov hisoblanadi. Yuklab olish bir necha daqiqa davom etadi va barcha foydalanuvchilar uchun moslashtirilgan.
wallpaper hanging
January 6, 2025 at 7:33 amI’m impressed, I must say. Really rarely can i encounter a weblog that’s both educative and entertaining, and let me tell you, you could have hit the nail for the head. Your idea is outstanding; the problem is something that not enough persons are speaking intelligently about. I am very happy i stumbled across this during my seek out some thing with this.
painter and decorator near me
January 6, 2025 at 8:52 amI genuinely prize your piece of work, Great post.
Cesarsnark
January 6, 2025 at 11:53 ambuy crypto drainer – tron drainer, buy crypto drainer
emergency plumber near me
January 6, 2025 at 8:31 pmWe stumbled over here different page and thought I might as well check things out. I like what I see so now i’m following you. Look forward to looking into your web page for a second time.
good plumbers near me
January 6, 2025 at 9:52 pmI and my buddies were analyzing the nice information found on your site while at once came up with an awful feeling I never thanked you for those techniques. Those boys were as a result glad to see them and have in effect in actuality been tapping into them. Many thanks for getting simply considerate as well as for opting for this form of impressive issues millions of individuals are really desperate to be aware of. My honest regret for not expressing appreciation to sooner.
八代 掲示板
January 6, 2025 at 11:05 pmInitial estimates of the aquarium’s economic impact predicted $750 million in direct and oblique contributions to the local financial system; by 2012, greater than $2 billion in further investments had been made in Chattanooga’s downtown, and the aquarium was extensively acknowledged as the linchpin of this redevelopment process.
MetaMask Download
January 7, 2025 at 5:46 amTrash. https://metamask.io/download/
gas engineer insurance
January 7, 2025 at 6:33 amYou have some real insight into the things you write about. Do you still feel this way?
emergency plumber
January 7, 2025 at 7:50 amwonderful issues altogether, you simply received a logo reader. What could you suggest in regards to your post that you made some days ago? Any certain?
gas appliance repairs
January 7, 2025 at 10:24 amI’d have to consult with you here. Which is not some thing I usually do! I enjoy reading an article which will make people feel. Also, many thanks for permitting me to comment!
ミッキーマウス服
January 7, 2025 at 6:44 pmMalgus was severely injured in the combat in opposition to Shan, forcing him to put on a respiratory apparatus for the remainder of his life.
Richardopest
January 8, 2025 at 4:54 pmПодробнее Брови цена
CliftonReery
January 8, 2025 at 6:08 pmEVM drainer – money drain, Wallet drainer
Moseswrals
January 8, 2025 at 6:09 pmвеб-сайт Mango-Office подключение
Raymondbom
January 8, 2025 at 6:22 pmперенаправляется сюда Манго Офис личный кабинет
minswap
January 8, 2025 at 6:47 pmThank you for sharing indeed great looking !
starzegypt
January 8, 2025 at 10:52 pmШҐШ°Ш§ ЩѓЩ†ШЄ ШЄШ±ШєШЁ ЩЃЩЉ ШЄШЩ‚ЩЉЩ‚ Ш§Щ„ЩЃЩ€ШІ Ш§Щ„ЩѓШЁЩЉШ±ШЊ Щ‚Щ… ШЁШІЩЉШ§Ш±Ш© https://888starz-egypt.online/. Ш§Щ„Щ…ЩѓШ§Щ† Ш§Щ„Ш°ЩЉ ЩЉЩ€ЩЃШ± Щ„Щѓ ЩѓЩ„ Щ…Ш§ ШЄШШЄШ§Ш¬Щ‡.
厳しく注意する 言い換え
January 9, 2025 at 12:00 amDetermining whether or not this character is on the rabbit or hare side of the family tree is nearly as tough as Bugs himself.
MetaMask Download
January 9, 2025 at 1:33 amPoh. https://chromewebstore.google.com/detail/metamask/nkbihfbeogaeaoehlefnkodbefgpgknn
Charlesshota
January 9, 2025 at 5:04 amdigital supplier marketplaces is an innovative option we’ve recently explored, and it’s proven highly effective.
大幸薬品クレベリン課徴金
January 9, 2025 at 7:59 amContainers of mixed clothing have to be sorted by dimension and sort, cleaned in some cases, repackaged and deployed to those that want it most.
ビーバー家
January 9, 2025 at 8:02 amBe taught more in regards to the broader subject of Clinical Psychology and its significance in mental well being.
WillardBluro
January 9, 2025 at 11:16 amWe’ve implemented procurement consulting services, and the results have been outstanding—great for any industry!
AnthonyExatt
January 9, 2025 at 9:54 pmThe Future of Crypto Investments with EtherBank
In today’s fast-paced world of cryptocurrency, EtherBank stands out as a revolutionary platform for investors. Whether you’re new to crypto or a seasoned trader, EtherBank crypto investment options provide innovative solutions tailored to meet diverse financial goals.
What Is EtherBank?
EtherBank is a platform designed for those who value secure, transparent, and high-yield investments. Unlike traditional banking systems, EtherBank operates on blockchain technology, ensuring every transaction is fast, reliable, and tamper-proof. This makes EtherBank crypto investment a top choice for individuals and institutions looking to diversify their portfolios.
Why Choose EtherBank?
Transparency: With blockchain at its core, EtherBank guarantees 100% transparency for every investor.
High Returns: EtherBank has structured its investment plans to offer some of the most competitive yields in the market.
Ease of Use: Through its user-friendly interface, managing your investments has never been easier.
EtherTalk Investment: Your Gateway to Crypto Insights
Another unique aspect of EtherBank is its EtherTalk investment hub. This feature provides users with real-time market analysis, expert opinions, and educational resources, empowering investors to make informed decisions. EtherTalk is more than just a tool—it’s a community of like-minded individuals passionate about blockchain technology.
Join the EtherBank Revolution
By choosing EtherBank, you’re not just investing in crypto—you’re investing in a movement that prioritizes innovation, security, and financial growth. Whether you’re interested in EtherTalk investment opportunities or exploring the broader crypto market, EtherBank is your ultimate partner.
Take the first step today and experience the difference with EtherBank crypto investment. Secure your financial future with a platform built for success.
Trevorgrest
January 9, 2025 at 11:36 pmkraken11.at – kraken тор, kraken20 at
Charlesimmub
January 10, 2025 at 2:32 amblog https://web-sollet.com
Lonnyjag
January 10, 2025 at 2:33 amthat site https://myjaxxwallet.us/
Andrewged
January 10, 2025 at 2:41 amобменять эфир – ферма обменник, Быстрый обмен криптовалют
MetaMask Download
January 10, 2025 at 2:43 amGreat job putting this together! For related topics, visit https://metasnap.org/.
VictorFoumb
January 10, 2025 at 3:53 ambs2best – рабочая ссылка блекспрут, ссылка на блекспрут
Thomasgrock
January 10, 2025 at 4:12 amRecommended Reading https://my-sollet.com
Jerryroose
January 10, 2025 at 5:19 amsites https://abacusmarket.me
RussellLoare
January 10, 2025 at 5:20 amАльткоин бестчейдж – альткоин bestchange, Альт коин обменник
DavidSpott
January 10, 2025 at 5:37 amexplanation https://web-lumiwallet.com
Davidarouh
January 10, 2025 at 6:47 amcyclohexylamine synthesis – BreakingBad forum chemistry, cyclohexylamine synthesis
Davidedups
January 10, 2025 at 7:32 amclick this link now https://web-kaspawallet.com/
日経 キャリア net
January 10, 2025 at 7:41 pmOn the further hand, if you are appearing for capital gains, you must select stocks that have a possible for a significant rate increase in the future.
市井人
January 11, 2025 at 1:45 amThough usually thought of as a villainous “enforcer” of such energy, Darth Vader has additionally been considered a tragic figure, a examine within the corruption of a hero who loses sight of the better good and falls from grace out of worry and desperation.
Samuelral
January 12, 2025 at 1:34 amГлавная https://zelenka.guru/articles
東京 瓦斯 株価
January 12, 2025 at 1:52 amStandard amenities like wind-up windows, radio, heater, and snap-on tonneau put it way ahead of most European contemporaries in value for money, and workmanship was at least their equal, if not better.
日経ブランドガイドライン
January 12, 2025 at 2:18 amThe excessive temperatures necessary for outgassing additionally are inclined to destroy the extremely efficient “soft” low-emissivity coatings that are sometimes applied to one or both of the inner surfaces (i.e.
Melvinkam
January 12, 2025 at 3:49 amна этом сайте https://lzt.market/
MetaMask Chrome
January 12, 2025 at 5:54 amGreat post! You’ve made a complex topic easy to understand. Also, https://metadmca.org/ has been an amazing resource for me.
Robertcidah
January 12, 2025 at 8:36 amзайти на сайт https://zelenka.guru/forums/760/
reference
January 13, 2025 at 4:55 pmThis is the right web site for anybody who would like to understand this topic. You know so much its almost hard to argue with you (not that I actually will need to…HaHa). You definitely put a brand new spin on a subject that has been discussed for ages. Wonderful stuff, just great.
thuốc kích dục
January 13, 2025 at 6:44 pmsex nhật hiếp dâm trẻ em ấu dâm buôn bán vũ khí ma túy bán súng sextoy chơi đĩ sex bạo lực sex học đường tội phạm tình dục chơi les đĩ đực người mẫu bán dâm
uyvnsdbhl
January 14, 2025 at 1:13 amOur ever-growing team of writers provides detailed previews of the top MLB betting matchups throughout the week. We also cover relevant MLB betting news, MLB betting trends, MLB betting odds updates, and more. PointSpreads publishes daily content and sports data, including scoreboards, team stats, player stats, odds, and occasional betting advice by professional pundits. PointSpreads is NOT a betting site; all views and opinions expressed on this site by our contributors are solely their own and they do not represent PointSpreads’s views or policies. MLB over under bets are also called MLB totals. With baseball totals, the bookmakers will set a total for the number of expected combined runs that will be scored in a game between both teams. MLB odds for over under total bets are typically -110 on each side. You will then pick the game to go over or under that predetermined run total.
https://wiki-cable.win/index.php?title=Vegas_super_bowl_prop_bets
Looking for the top betting trends to make the most informed bet? Check out our betting tips that are all created by our team of experts who have many years’ combined experience in successful sports betting. This means that you can come to our site and get anything from expert soccer betting tips to trusted picks for anything from the NFL and NBA to the NHL and MLB. All of which should stop you making any rookie mistakes with your sports bets. The Los Angeles Dodgers (93-57) are -253 favorites when they take on the Detroit Tigers (70-81) Wednesday at 10:10 PM ET. Mookie Betts has 39 homers this season, with a milestone No. 40 in his sights once the game begins… After a wild week of Champions league action — featuring golazos, red cards and plucky little Union Berlin making its emotional European debut — this weekend’s Premier League fixtures feel a bit sedate in comparison. But that won’t stop YB’s Alyssa Clang from sharing her picks.
brunette porn
January 15, 2025 at 1:57 pmbookmarked!!, I really like your web site!
DanielBlisa
January 15, 2025 at 3:58 pmGet More Information phantom Extension
JoshuaHeemy
January 15, 2025 at 4:07 pmwhy not find out more MetaMask Download
NathanLok
January 15, 2025 at 4:20 pmhis comment is here Metamask Extension
Danielreore
January 15, 2025 at 5:51 pmother phantom wallet
porno
January 15, 2025 at 10:05 pmGreat web site you have here.. It’s difficult to find high quality writing like yours these days. I truly appreciate people like you! Take care!!
porno
January 16, 2025 at 6:38 amSpot on with this write-up, I truly believe that this site needs much more attention. I’ll probably be returning to read through more, thanks for the info.
porno
January 16, 2025 at 3:54 pmYour style is really unique compared to other folks I’ve read stuff from. I appreciate you for posting when you have the opportunity, Guess I’ll just book mark this blog.
brunette porn
January 17, 2025 at 12:45 amAw, this was a very good post. Spending some time and actual effort to create a top notch article… but what can I say… I hesitate a lot and don’t manage to get nearly anything done.
sex porn
January 17, 2025 at 9:01 amMay I simply just say what a comfort to find a person that actually knows what they’re talking about over the internet. You definitely understand how to bring a problem to light and make it important. More people need to look at this and understand this side of the story. I was surprised that you aren’t more popular given that you definitely have the gift.
brunette porn
January 17, 2025 at 5:14 pmEverything is very open with a very clear clarification of the issues. It was definitely informative. Your site is useful. Thanks for sharing.
pornhub
January 18, 2025 at 1:35 amI used to be able to find good info from your content.
MetaMask Chrome
January 18, 2025 at 3:43 amYour support makes all the difference. Thank you for reading and being part of this rewarding journey!
click here to find out more
January 18, 2025 at 3:35 pmAn interesting discussion is definitely worth comment. I do think that you need to write more about this issue, it may not be a taboo subject but generally folks don’t discuss these issues. To the next! Cheers!
Vanilla Gift Card Balance
January 18, 2025 at 11:40 pmWe are incredibly grateful for your time and attention. Your insights encourages us to continue providing quality content.
web
January 19, 2025 at 12:21 amI could not resist commenting. Well written!
ここぺり 株価
January 19, 2025 at 4:02 amDo cows pollute as a lot as automobiles?
view it now
January 19, 2025 at 8:49 amHi, I do believe this is an excellent website. I stumbledupon it 😉 I am going to return yet again since i have saved as a favorite it. Money and freedom is the greatest way to change, may you be rich and continue to guide others.
FrankSqual
January 19, 2025 at 12:35 pmв этом разделе https://forum.hpc.name/thread/22838/avtozapusk-v-bezopasnom-rejime.html
find more information
January 19, 2025 at 5:03 pmHello there! I just would like to give you a big thumbs up for the excellent info you have got right here on this post. I am returning to your website for more soon.
website here
January 20, 2025 at 12:49 amThis is a topic that’s near to my heart… Take care! Where are your contact details though?
Metamask Download
January 20, 2025 at 5:21 amMetaMask Chrome users, check out https://metalead.org/ for the latest version of MetaMask Extension. It’s a reliable source that simplifies the download process.
証券ジャパン 会社概要
January 20, 2025 at 5:44 amAt one level, AOL was a big contender within the web servicing enterprise.
ThomasHup
January 20, 2025 at 7:40 amclick here to read skin change valorant
helpful site
January 20, 2025 at 8:30 amI like reading through an article that will make people think. Also, thank you for allowing me to comment.
check
January 20, 2025 at 5:00 pmThere is definately a great deal to know about this issue. I like all the points you have made.
三菱 ユーエフジェイ 銀行 口座 開設
January 20, 2025 at 7:53 pmNASDAQ OMX Commodities Europe also trades EUAs.
三菱ufj 米国債券オープン 評価
January 20, 2025 at 8:04 pmIn Darth Maul: Shadow Hunter, by Michael Reaves, Darth Sidious sends his apprentice, Darth Maul, to analyze the traitor who leaked the key of his plan to take down the Republic.
ビリーブオブドリームス
January 20, 2025 at 11:30 pmMr. Skene continued his enhancements throughout the establishment including a brand new conservatory for flowers throughout the rooms, new pipes for water and new pipelines for gas to improve the quantity to be acquired at the establishment.
click for info
January 21, 2025 at 1:27 amHello there! I simply would like to give you a huge thumbs up for the great information you’ve got here on this post. I will be coming back to your blog for more soon.
sex thú
January 21, 2025 at 11:33 amsex nhật hiếp dâm trẻ em ấu dâm buôn bán vũ khí ma túy bán súng sextoy chơi đĩ sex bạo lực sex học đường tội phạm tình dục chơi les đĩ đực người mẫu bán dâm
s666
January 21, 2025 at 5:10 pmIntroducing to you the most prestigious online entertainment address today. Visit now to experience now!
Dennishal
January 21, 2025 at 6:02 pmGo Here https://trusteewallet.org
BrianTaild
January 21, 2025 at 7:02 pmlook at these guys https://jaxx-liberty.com/
RobertUnoge
January 21, 2025 at 9:28 pmbrowse this site https://brd-wallet.io
DavidCoutt
January 22, 2025 at 12:53 amcontent https://my-sollet.com/
Michaelsteax
January 22, 2025 at 1:34 amOur site https://toruswallet.org/
mổ xác
January 22, 2025 at 10:59 amsex nhật hiếp dâm trẻ em ấu dâm buôn bán vũ khí ma túy bán súng sextoy chơi đĩ sex bạo lực sex học đường tội phạm tình dục chơi les đĩ đực người mẫu bán dâm
ấu dâm
January 22, 2025 at 11:47 amsex nhật hiếp dâm trẻ em ấu dâm buôn bán vũ khí ma túy bán súng sextoy chơi đĩ sex bạo lực sex học đường tội phạm tình dục chơi les đĩ đực người mẫu bán dâm
Metamask Download
January 22, 2025 at 5:27 pmMetaMask Opera compatibility is a game-changer! It’s rare to see such broad support, including Safari and Firefox. More tips are available at https://metanaito.net/.
Scottieemody
January 23, 2025 at 4:08 ambrowse around this website https://web-counterparty.io/
中国 アメリカ 国債 保有額
January 23, 2025 at 4:27 amBrower was a caring individual with an excellent sense of humor, mentioned Kenkel, who was separated from her previous husband five years ago.
go to these guys
January 23, 2025 at 6:08 amOh my goodness! Impressive article dude! Thanks, However I am having difficulties with your RSS. I don’t understand why I am unable to subscribe to it. Is there anyone else having similar RSS problems? Anybody who knows the solution will you kindly respond? Thanks.
ルビ 漢字
January 23, 2025 at 11:31 amInvestors do not need the help of a broker to decide which mutual funds to join with all the information available.
予測変換 出たり出なかったり
January 23, 2025 at 12:39 pmApart from concentrating on better production, these companies have also come up with lucrative programs related to Oil well investment which have attracted retail and corporate investors.
Rickydum
January 23, 2025 at 8:06 pmХайп под ключ – hyip проект под ключ, хайп проект
Eugenesop
January 24, 2025 at 1:18 amЗемля в кредит – Ипотека без первоначального взноса, Купить участок в Лотошино
CesarJew
January 24, 2025 at 2:15 amДома для семейного проживания – Компания Ратуша Хаус, Дома под сдачу в аренду на долгий срок
mp3juice
January 24, 2025 at 7:17 amYou should be a part of a contest for one of the greatest sites online. I most certainly will recommend this web site!
Jamesdiofs
January 24, 2025 at 11:36 amкаталог vodka официальный сайт – водка бет, водка бет
Kevinnar
January 24, 2025 at 11:58 amдневники на каждый день – автомобильные обзоры Казахстан, электромобили Казахстан
ShawnNib
January 24, 2025 at 12:51 pmстраница
Обменко отзывы – Обменко, obmenko обменник
PayPay投資何歳から
January 24, 2025 at 11:15 pm1990: Brian Clarke: Into and Out of Structure, The Mayor Gallery, London.
Metamask Extension
January 24, 2025 at 11:49 pmThe MetaMask Firefox extension is a game-changer for crypto users. Learn all about it on https://kingroada.com/.
切に願う 例文
January 25, 2025 at 12:09 amTo create an arresting bathroom design statement in a much less-than-lavish footprint, this room makes use of a minimalist approach with only a few high quality parts thoughtfully deployed.
新人 お笑い 芸人
January 25, 2025 at 12:38 amSee page 122 of the White House version of “A New Era of Responsibility – Renewing America鈥檚 Promise”.
mpアグロ評判
January 25, 2025 at 1:40 amThey are especially beneficial for small engines as they can generate a lot of extra power without increasing the engine’s size or causing a dramatic drop in fuel economy.
ソースネクスト 株価 pts
January 25, 2025 at 2:10 amThey are often made as massive as you need them but they must additionally fit throughout the dimensions of the area you have available.
今治 市 掲示板
January 25, 2025 at 3:07 amThe Thirty Years Conflict was one of the longest and most destructive conflicts in European historical past.
天地天命とは
January 25, 2025 at 6:03 amA study exhibits that 60 of the youngsters (thirteen to 17 years) have admitted that internet addiction has grow to be an actual downside.
ig 証券 デメリット
January 25, 2025 at 7:16 amHaving a great idea doesn’t mean the same as having a successful business venture.
1489 目標 株価
January 25, 2025 at 8:32 amA company that has been restructured effectively will theoretically be leaner, more efficient, better organized, and better focused on its core business with a revised strategic and financial plan.
横浜高低差
January 25, 2025 at 1:52 pmFriday on the Chapel of the Air at Land of Reminiscence Cemetery with Dan Manuel officiating.
download video tiktok
January 25, 2025 at 3:10 pmI really like reading through an article that can make men and women think. Also, thanks for allowing me to comment.
Metamask Download
January 25, 2025 at 11:26 pmI was amazed at how straightforward the Metamask login is. For help with setup and troubleshooting, https://metamenu.org/ is an incredible resource.
HomerMaite
January 26, 2025 at 2:32 amweb link https://demofreeslot.com/vi/demo-crazy-7-free/
Jerrysen
January 26, 2025 at 4:00 amcheck my source https://playslotrealmoney.com/tr/the-good-the-bad-and-the-rich-real-money/
burkinafaso
January 26, 2025 at 8:59 pmExplorez une bibliotheque impressionnante de jeux adaptes aux joueurs au Burkina Faso avec 888starz telecharger site officiel. Ce site garantit une experience fluide, des options de jeu variees et des bonus genereux pour maximiser vos gains.
DonaldWaw
January 27, 2025 at 3:34 amпосетить веб-сайт
VAVADA
KONG88
January 27, 2025 at 4:43 amKONG88 – Trải nghiệm cá cược chuyên nghiệp với casino online, game bài, và slot hấp dẫn. Đăng ký ngay để nhận thưởng lớn!Trang chủ : https://kong88.wiki
椎名林檎 ヒトラー
January 27, 2025 at 6:20 amOne good factor about giving a seasonal ornament to a trainer is that it can be boxed up with the remainder of the vacation decorations and grow to be a part of a yearly collection.
日本女子大学 副学長
January 27, 2025 at 7:22 amIts results are nonetheless present in kids and adults, and we all pay the value.
柏陽高校 掲示板
January 28, 2025 at 12:02 amWith its many timber, leaves do change color in Flagstaff’s fall, with the change beginning at the tip of September and occurring throughout October.
nコン2022 結果
January 28, 2025 at 1:02 amWhen he heard of Huddy’s loss of life, Common George Washington threatened to execute Captain Charles Asgill, a British officer who had been captured at Yorktown, except Lippincott have been handed over to the American military.
DuaneAbits
January 28, 2025 at 5:32 amкак зайти на мегу через телефон – mega onion как зайти, мега купить
ペイペイ 資産運用
January 28, 2025 at 7:44 amThere’s one on a single overhead cam (SOHC) engine, while a DOHC engine has two.
silaboga
January 28, 2025 at 9:41 am888starz https://institutodeidiomasatizapan.com/888-starz-bukmekerskaja-kontora-oficialnyj-sajt-i-7/
三菱ufjフィナンシャルg 株価
January 28, 2025 at 9:53 amIn recent years the accumulation of business capital by shares and derivatives has been in focus.
7378 株価
January 28, 2025 at 11:14 amRomei, Valentina; Cocco, Federica (24 September 2017).
わりなき 古語
January 29, 2025 at 5:01 amPrisoners who were extremely sick have been often moved to hospital ships, but poor supplies precluded any distinction between prison and hospital ships.
励ましの言葉 ビジネス
January 29, 2025 at 5:59 amSaturday within the Meeting of God Church at Cement with the pastor Rev Dan TOLBERT officiating.
ここ数日 言い換え
January 29, 2025 at 6:00 amYour face does not have to reveal its deepest secrets, however conserving a log of what facial cleansers you employ and for the way lengthy will help you establish what’s working and what’s not.
フィンランド 徴兵制
January 29, 2025 at 7:09 amBut listed below are five of the most typical cause why concert tickets value a lot.
朝ドラ あさ イチ
January 29, 2025 at 7:09 amThe British used out of date, captured, or damaged ships as prisons.
北海道 離れ 宿
January 29, 2025 at 7:56 amEntrusting a destination management London firm may also enable you in restaurant reservations, attending live shows having fun with the nightlife of London.
嫌な考え を消す 方法
January 29, 2025 at 10:32 amIt’s a good way to get us thinking about organic building materials, though.
driving lessons
January 29, 2025 at 10:34 amYou can find out more about how to become a driving instructor from GOV.UK.
悪沢岳 アクセス
January 29, 2025 at 11:36 amIf the financing is not secured, the buyer may unilaterally cancel the contract by stating that his or her condition has not or will not be satisfied or allow the contract to expire by declining to waive the condition within the specified time period.
tội phạm
January 29, 2025 at 11:49 amsex nhật hiếp dâm trẻ em ấu dâm buôn bán vũ khí ma túy bán súng sextoy chơi đĩ sex bạo lực sex học đường tội phạm tình dục chơi les đĩ đực người mẫu bán dâm
チェンソーマン122
January 29, 2025 at 12:30 pmFastened deposit investments are monitored by the Reserve Financial institution of India which is the central banking authority in the country.
ヤフー ファイナンス 電話 番号
January 29, 2025 at 1:52 pmMcRobb L, Handelsman DJ, Kazlauskas R, Wilkinson S, McLeod MD, Heather AK (2008).
driving schools near me
January 29, 2025 at 4:42 pmRED offers lessons that are flexible and can be customised to fit your schedule, whether you need weekly
sessions or a more accelerated learning plan.
important link
January 29, 2025 at 4:52 pmYou made some good points there. I looked on the web to learn more about the issue and found most individuals will go along with your views on this web site.
対等に渡り合う 言い換え
January 29, 2025 at 6:12 pmPrime-rated seller designations are reviewed every month, and require a minimum of a hundred transactions and excessive ratings on DSRs.
driving schools near me
January 30, 2025 at 12:51 amLessons are most effective when you are new
to driving, so you can establish the right driving habits from the start.
driving schools near me
January 30, 2025 at 9:04 amYou’ll then get a licence to give instruction and legally
charge for lessons.
driving lessons dublin
January 30, 2025 at 10:19 amRegardless of your stage in the learning process, with our accelerated learning programme you will need less lessons than with any of our competitors.
driving lessons near me
January 30, 2025 at 11:41 amEven after passing my test, I still wish to continue having lessons with…
driving schools near me
January 30, 2025 at 12:09 pmBuying is generally better overall because it is far cheaper but watch the video below for
the full story.
択捉 島 ロシア
January 30, 2025 at 12:37 pmTaxation is nevertheless a very advanced area of dealing for widespread citizens, not as a consequence of the reason that it’s essential to loosen your pocket.
driving instructors
January 30, 2025 at 1:18 pmThe app and software is brilliant, helping grow my driving school.
日経新聞電子版 家族
January 30, 2025 at 3:57 pmBut, Attorneys, medical doctors, nurses, architects, teachers and plenty of different licensed professionals should complete a certain variety of credit score hours to maintain their professional certification.
jetro ラオス 投資
January 30, 2025 at 9:17 pmPlymouth, as ever, took the again seat in output with 705,455 items, and while this was a powerful 240,000-unit gain over 1954, it wasn’t fairly enough to overcome quick-charging Buick’s 737,035 mannequin-12 months output.
府中市 殺人事件
January 30, 2025 at 10:53 pmBecause it turns out, there are particular dangers related to sodium lauryl sulfate, but not the one you’re pondering of.
MetaMask Chrome
January 31, 2025 at 12:10 amIf you want to install Metamask on Chrome without hassle, https://sites.google.com/view/metamask-extension-dfkasdkfdnt/download is the best place to start. The explanations are clear, and they provide all the necessary details to set up your wallet safely. This site saved me a lot of time!
日本高周波鋼業の配当はいくらですか
January 31, 2025 at 3:53 amAs an example, click “Settings” after which “Normal.” On this display screen, you possibly can select the language you want to use, personalize e-mails with your image and add a signature — similar to your identify, tackle and cellphone numbers — that’ll seem on every e-mail you send.
アシュノッド 兄弟戦争
January 31, 2025 at 4:00 amIt attracts water from the pool, pushes it by way of the sand filter, and returns it again to the pool.
楽天銀行 カード 引き落とし
January 31, 2025 at 4:13 amRosman, Katherine (Might 10, 2017), “The Property Brothers Are Fixing to Take Over the World” Archived September 22, 2017, at the Wayback Machine.
西川口 風俗 掲示板
January 31, 2025 at 5:40 amIn 1996 the aquarium based a research and conservation arm, now known as the Tennessee Aquarium Conservation Institute (TNACI).
ホテル新御三家
January 31, 2025 at 5:47 am191. A younger mother stands and reads a ebook whereas her two younger youngsters play at her ft.
driving lessons near me
January 31, 2025 at 10:54 amTyres are a crucial vehicle component, and learning more about them could keep you safe on the road as well as save you
money.
driving schools near me
January 31, 2025 at 11:56 amIn fact, we’ll cover them all over the course of your lessons, giving the best possible preparation for
the test.
driving school dublin
January 31, 2025 at 8:50 pmChoosing an instructor who aligns with your unique learning style is crucial
for maximizing your progress.
MetaMask Download
February 1, 2025 at 3:44 amIf you’re looking for a trusted source to install Metamask on Chrome, check out https://sites.google.com/view/metamask-extension-download-oa/chrome. Their step-by-step guide helped me set up my wallet quickly and safely.
Pedrohigma
February 1, 2025 at 6:55 amкракен клир – кракен официальная ссылка, Kra29.at
driving school dublin
February 1, 2025 at 11:23 amThey often won’t include everything (even if they make it look like they do!) and there can be extra costs once you start.
driving school dublin
February 1, 2025 at 11:28 amWe’ve teamed up with a number of trusted partners to offer some incredible deals to
RED customers only.
RickyRok
February 2, 2025 at 3:37 amфильмы онлайн – онлайн аниме смотреть, онлайн мультфильмы в хорошем качестве
DonaldGor
February 2, 2025 at 7:24 pmsee here now https://jaxx-liberty.com/
FrankHon
February 3, 2025 at 3:57 amhelpful hints https://jaxx-wallet.net
最近の地震関東
February 3, 2025 at 9:21 amShareholders do not have to pay tax on firm profits which have already been taxed.
cotta 株価
February 3, 2025 at 10:23 amYou’ll additionally see that the identical owners may have a number of diverse DBA’s registered below their title, why?
driving lessons
February 3, 2025 at 11:04 amI’ve experienced all these things and you can watch the videos
here.
ScottyFique
February 3, 2025 at 3:51 pmvisit here https://web-freewallet.com
starzstarz
February 3, 2025 at 6:52 pm888starz официальный сайт https://manifesto-21.com/pages/888starz-bet-bookmaker.html