Custom Action Filters in ASP.NET MVC 5
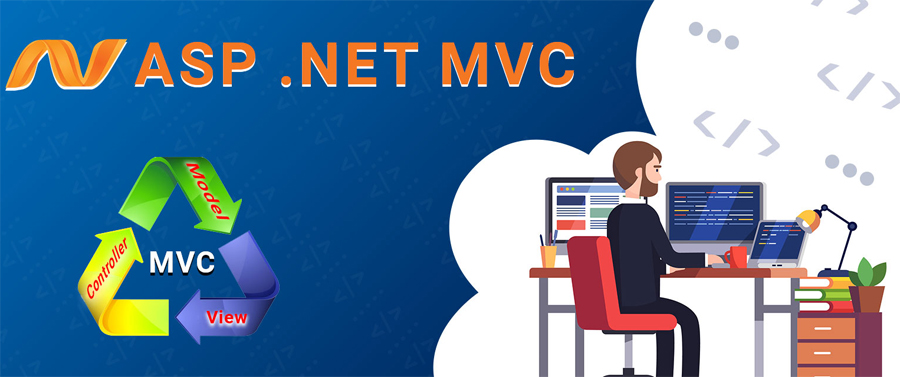
Example Code: https://drive.google.com/file/d/1XFZdb_MtHAsoqe4mQG3_gx5q-K1SnUSJ/view?usp=sharing
Just like a built in attribute we can also create custom action filters , in this article we will create custom action filter step by step.
In this example we will create a custom action filter which when applied will generate the log of that action (name of action, controller name, datetime) in database table.
Step 1 : Create a table in database with name ALog as follows –
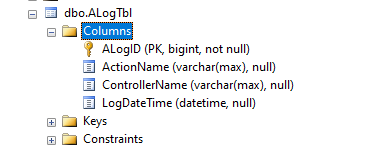
Step 2: Create a entity Model using DbFirst Approach for ALogTbl as follows –
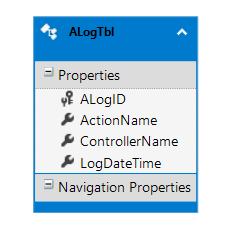
Step 3: Create ASP.NET MVC Web Application with Test controller with following Actions –
public class TestController : Controller
{
// GET: Test
public ActionResult Index()
{
return View();
}
public ActionResult About()
{
return View();
}
public ActionResult Contact()
{
return View();
}
}
Step 4: Create CustFilter folder to create a custom filter and define a class ALog in it.
To create a Custom Action filter we need to define a class which inherits from ActionFilterAttribute, then override the method OnActionExecuting or OnActionExecuted. OnActionExecuting method will be executed just before the execution of the action. OnActionExecuted method will be executedafter execution of the the action.
For this example override the OnActionExecuting method and write the following code to create the log of the Action and Controller in ALogTbl.
public class ALog :ActionFilterAttribute
{
PracticeDBEntities entity = new PracticeDBEntities();
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
ALogTbl rec = new ALogTbl();
rec.ActionName = filterContext.ActionDescriptor.ActionName;
rec.ControllerName = filterContext.ActionDescriptor.ControllerDescriptor.ControllerName;
rec.LogDateTime = DateTime.Now;
entity.ALogTbls.Add(rec);
entity.SaveChanges();
}
}
Step 5: Now apply the ALog filter to the actions from the test controller or on on TestController as follows –
namespace ActionFilterEx.Controllers
{
[ALog]
public class TestController : Controller
{
// GET: Test
public ActionResult Index()
{
return View();
}
}
Ste 6 : No call the actions as per requirements and it will log the data in ALogTbl and check ALogTbl will get as follows –
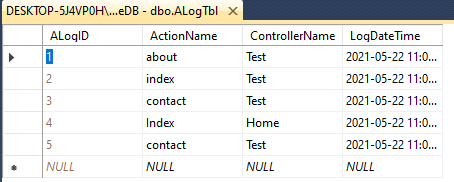
Judy-Z
July 6, 2024 at 4:51 pmI like this web blog it’s a master piece! Glad I noticed this on google.?
Https://Www.Google.Co.Vi/
July 11, 2024 at 8:26 pmWhere Are You Going To Find Porn Star Be 1 Year From What Is Happening Now?
British Porn Stars On Adultwork [https://Www.Google.Co.Vi/]
window and Door doctor
July 12, 2024 at 4:42 am15 Reasons You Shouldn’t Ignore Door Doctor window and Door doctor
upvc Patio Door repairs
July 12, 2024 at 9:23 amThree Reasons To Identify Why Your Patio Door Repairs Near Me
Isn’t Performing (And The Best Ways To Fix It) upvc Patio Door repairs
Vicente
July 12, 2024 at 3:21 pmTechnology Is Making Drip Filter Better Or Worse?
Vicente
Local Seo
July 12, 2024 at 10:51 pm10 No-Fuss Methods To Figuring The Local Business
SEO You’re Looking For Local Seo
Window repair near Me
July 12, 2024 at 10:57 pm5 Killer Quora Answers On Window Repair Near Me Window repair near Me
913875
July 12, 2024 at 11:05 pmWhat Do You Do To Know If You’re Ready To Go After White Electric Stove
913875
Windows Replacement glass
July 12, 2024 at 11:27 pm20 Inspiring Quotes About Replacement Window Glass Windows Replacement glass
www.jerealas.top
July 12, 2024 at 11:45 pm9 Signs That You’re A Double Glazing Repair Expert http://www.jerealas.top
Articlescad.Com
July 12, 2024 at 11:48 pmSee What Double Glazing Repairs Near Me Tricks The
Celebs Are Using Double Glazing Repairs Near
Me (Articlescad.Com)
jerealas.top
July 13, 2024 at 12:05 amTen Double Glazing Condensation Repair Cost Myths That Aren’t Always True
jerealas.top
upvc repairs near me
July 13, 2024 at 12:32 amThe 10 Most Terrifying Things About Upvc Repairs Near Me upvc repairs near me
Federal Employers
July 13, 2024 at 12:35 amSee What Federal Employers Tricks The Celebs Are Utilizing Federal Employers
http://wownsk-portal.ru/
July 13, 2024 at 12:48 am9 Things Your Parents Taught You About Upvc Window Repairs Near Me
upvc window Repairs near Me, http://wownsk-portal.ru/,
upvc window repair
July 13, 2024 at 12:51 amYou’ll Be Unable To Guess Upvc Window Repairs Near
Me’s Tricks upvc window repair
Damien
July 13, 2024 at 12:53 am10 Best Mobile Apps For Car Lock Smith Damien
Upvcwindows
July 13, 2024 at 1:26 amWhy Replacement Handles For Upvc Windows Isn’t A Topic That People Are Interested In. Upvcwindows
Accidents
July 13, 2024 at 1:35 amWhat’s The Most Important “Myths” About Accident Attorney Might Be True Accidents
personal Injury
July 13, 2024 at 1:46 amGuide To Personal Injury Litigation: The Intermediate Guide For Personal Injury Litigation personal Injury
upvc windows near Me
July 13, 2024 at 1:49 amNine Things That Your Parent Teach You About Upvc Windows
Near Me upvc windows near Me
glamorouslengths.com
July 13, 2024 at 1:52 amThe Top Reasons Why People Succeed In The Personal Injury Law Industry personal injury attorney (glamorouslengths.com)
double Glazing window Keys
July 13, 2024 at 2:15 amThree Greatest Moments In Double Glazed Window Near Me History double Glazing window Keys
Titration adhd medications
July 13, 2024 at 2:27 am9 . What Your Parents Taught You About Titration ADHD Medications Titration adhd medications
Willysforsale.Com
July 13, 2024 at 2:34 amYou’ll Never Guess This Railroad Injuries Lawyers’s Benefits Railroad Injuries Lawyers (Willysforsale.Com)
demo2-Ecomm.in.ua
July 13, 2024 at 2:45 amGuide To Upvc Repairs Near Me: The Intermediate Guide
For Upvc Repairs Near Me upvc Repairs near Me (demo2-Ecomm.in.ua)
legal
July 13, 2024 at 3:01 am9 Signs That You’re An Expert Federal Railroad Expert legal
lynnbolvin.top
July 13, 2024 at 3:08 amHow Do You Explain Electric Fireplace To A Five-Year-Old lynnbolvin.top
Sweet Bonanza ™
July 13, 2024 at 3:36 amYour Family Will Be Thankful For Having This Demo Sweet
Bonanza Sweet Bonanza ™
repair Upvc Window
July 13, 2024 at 3:41 amWhat’s The Current Job Market For Upvc Windows Repair Professionals?
repair Upvc Window
adhd assessment for Young adults
July 13, 2024 at 4:34 am5 Lessons You Can Learn From Adult Adhd Assessment
adhd assessment for Young adults
Walker
July 13, 2024 at 4:39 amA Brief History Of Boot Scooter History Of Boot Scooter Walker
Zack Foxworth
July 13, 2024 at 4:53 amTen Common Misconceptions About Treadmills Folding That Aren’t Always True
Zack Foxworth
skoda key fob programming
July 13, 2024 at 5:06 am10 Things We Are Hating About Skoda Fabia Key Replacement skoda key fob programming
Jolene
July 13, 2024 at 5:13 amADHD Traits In Women Tips To Relax Your Daily Life ADHD
Traits In Women Trick That Should Be Used By Everyone Know adhd
traits in women (Jolene)
supply Only upvc windows
July 13, 2024 at 5:17 am7 Easy Secrets To Totally Doing The Upvc Door And Windows supply Only upvc windows
lexus Replacement Key cost
July 13, 2024 at 5:50 amWhat’s The Current Job Market For Lexus Replacement Key Cost Professionals Like?
lexus Replacement Key cost
Double Glazed Window Repairs Near Me
July 13, 2024 at 5:56 am5 Killer Quora Answers On Double Glazed Window Repairs Near Me Double Glazed Window Repairs Near Me
upvc door repairs near me
July 13, 2024 at 6:16 amThe 10 Most Scariest Things About Upvc Door Repairs Near Me upvc door repairs near me
Adhd Assessment For Adults
July 13, 2024 at 6:24 amWhat’s The Job Market For Adhd Assessment For Adults Professionals Like?
Adhd Assessment For Adults
double glaze doors
July 13, 2024 at 6:42 amWhy The Double Glazed Door Repairs Near Me Is Beneficial In COVID-19?
double glaze doors
repairing upvc windows
July 13, 2024 at 7:14 amWhat Is Repair Upvc Windows And Why Is Everyone Talking About It?
repairing upvc windows
Shiela
July 13, 2024 at 7:33 amTen Stoves That Really Improve Your Life Shiela
Double Glazing upvc windows
July 13, 2024 at 7:41 amLooking For Inspiration? Look Up Upvc Double Glazed Windows Double Glazing upvc windows
Cassy Lawn
July 13, 2024 at 8:10 amThe 10 Worst Asbestos Attorney Fails Of All Time Could Have Been Avoided Cassy Lawn
https://Minecraftcommand.science/
July 13, 2024 at 9:13 amUpvc Window Repairs Near Me Tools To Ease Your Everyday Lifethe Only Upvc Window Repairs Near Me Technique Every Person Needs To Know upvc window
repairs near me (https://Minecraftcommand.science/)
window repair near me
July 13, 2024 at 9:24 amSee What Upvc Window Repair Near Me Tricks The Celebs Are Making Use Of window repair near me
window replacement near Me
July 13, 2024 at 9:28 amYou’ll Never Guess This Window Replacement Near Me’s Tricks window replacement near Me
Adhd and depression in women
July 13, 2024 at 9:42 amADHD In Adult Women: The History Of ADHD In Adult Women In 10 Milestones
Adhd and depression in women
Shanel
July 13, 2024 at 9:46 amIt’s The Door Repair Near Me Case Study You’ll Never Forget
window replacement near me (Shanel)
Https://Marvelvsdc.Faith
July 13, 2024 at 9:51 amThe 10 Most Terrifying Things About Double
Glazing Companies Near Me Double Glazing Companies Near Me (https://Marvelvsdc.Faith)
Zack Foxworth
July 13, 2024 at 9:55 am10 Things Your Competitors Learn About Treadmills That Fold
Flat Zack Foxworth
double glazing repairs near me
July 13, 2024 at 10:02 amSee What Double Glazing Repairs Near Me Tricks The Celebs Are Using double glazing repairs near me
Https://Speedgh.Com/Index.Php?Page=User&Action=Pub_Profile&Id=934171
July 13, 2024 at 10:51 am5 Killer Quora Answers On Fela Case Settlements Fela Case Settlements (https://Speedgh.Com/Index.Php?Page=User&Action=Pub_Profile&Id=934171)
Clinton Medical Malpractice Law Firm
July 13, 2024 at 10:53 amA Medical Malpractice Law Success Story You’ll Never Believe Clinton Medical Malpractice Law Firm
veterans Disability attorney
July 13, 2024 at 11:06 amWhat’s The Job Market For Veterans Disability Attorney Professionals?
veterans Disability attorney
personal Injury lawyer
July 13, 2024 at 11:13 amWhy Nobody Cares About Personal Injury Compensation personal Injury lawyer
articlescad.com
July 13, 2024 at 11:23 amWhat’s The Job Market For Double Glazing Near Me Professionals?
double glazing near me [articlescad.com]
Replacement Window Glass
July 13, 2024 at 11:43 amThe 10 Most Terrifying Things About Replacement Window Glass Replacement Window Glass
www.3222914.xyz
July 13, 2024 at 11:52 amWhy Do So Many People Are Attracted To Cheap Table Top Freezer?
http://www.3222914.xyz
personal injury attorneys
July 13, 2024 at 11:53 amFive Killer Quora Answers To Personal Injury Attorneys personal injury attorneys
Cassy Lawn
July 13, 2024 at 11:53 am10 Attorney For Asbestos That Are Unexpected
Cassy Lawn
Cesar
July 13, 2024 at 12:14 pmThe Most Hilarious Complaints We’ve Been Hearing About Slot Demo
Zeus Gratis akun demo slot pragmatic zeus (Cesar)
upvc window repairs
July 13, 2024 at 12:14 pmThe Ultimate Glossary Of Terms About Upvc Windows Repair upvc window repairs
Delores
July 13, 2024 at 12:49 pmThe Leading Reasons Why People Are Successful Within The Door Doctor Industry
window caulking (Delores)
firm
July 13, 2024 at 1:07 pmWhat’s Holding Back This Railroad Injuries Law Industry?
firm
https://www.cheaperseeker.com/
July 13, 2024 at 1:19 pmWhat’s The Job Market For Upvc Repairs Near Me Professionals?
upvc repairs near me (https://www.cheaperseeker.com/)
veterans disability attorney
July 13, 2024 at 1:25 pm7 Useful Tips For Making The Most Out Of Your Veterans Disability Claim
veterans disability attorney
Signs And Symptoms Of Adhd In Adults
July 13, 2024 at 1:28 pmWhat’s The Fuss About Adhd In Adults Symptoms? Signs And Symptoms Of Adhd In Adults
Wooden
July 13, 2024 at 1:58 pmCould Door Repair Near Me Be The Answer To Achieving 2023?
Wooden
Meri
July 13, 2024 at 2:16 pmHow Window Replacement Near Me Is A Secret Life Secret Life Of Window Replacement Near Me cost of
windows replacement (Meri)
firms
July 13, 2024 at 2:19 pmGet To Know Your Fellow Boat Accident Attorney Enthusiasts.
Steve Jobs Of The Boat Accident Attorney Industry firms
0270469.xyz
July 13, 2024 at 2:23 pm10 Things You Learned In Preschool That Will Help You With Mesothelioma And Asbestosis 0270469.xyz
Elsy Crays
July 13, 2024 at 2:24 pm10 Tips For Getting The Most Value From Locksmith Near Me For Cars Elsy Crays
private adhd Assessment near me
July 13, 2024 at 3:19 pmPrivate ADHD Assessment Near Me Tools To Improve Your Everyday Lifethe Only Private ADHD Assessment Near Me Technique Every Person Needs To Be Able To private adhd Assessment near me
eddafay
July 13, 2024 at 3:19 pm15 Things To Give Your Bunk Bed For Sale Lover In Your Life eddafay
kaymell
July 13, 2024 at 3:22 pmBuzzwords De-Buzzed: 10 Different Ways To Say Online Slots kaymell
836614
July 13, 2024 at 3:27 pmTen Healthier Pet That Will Actually Help You Live Better 836614
Henry
July 13, 2024 at 3:39 pmSeven Explanations On Why Medical Malpractice Settlement Is So
Important medical malpractice lawsuits (Henry)
Window Doctor Near Me
July 13, 2024 at 3:54 pmWhat’s The Job Market For Window Doctor Near Me Professionals?
Window Doctor Near Me
https://www.longisland.com/
July 13, 2024 at 3:56 pm5 Killer Quora Answers On Replacement Double Glazing Units Near Me replacement double glazing Units near me,
https://www.longisland.com/,
upvc window repairs
July 13, 2024 at 4:04 pm10 Startups That Are Set To Revolutionize The
Upvc Window Locks Industry For The Better upvc window repairs
Malpractice Lawyer
July 13, 2024 at 4:11 pmAre You Confident About Doing Malpractice Legal? Take This Quiz
Malpractice Lawyer
netvoyne.ru
July 13, 2024 at 4:26 pm9 Lessons Your Parents Taught You About Upvc Window Repairs Near Me upvc window
repairs near me (netvoyne.ru)
99811760
July 13, 2024 at 4:57 pmHow To Make A Profitable Spare Key For Car Entrepreneur Even If You’re Not
Business-Savvy 99811760
slot starlight Princess Demo
July 13, 2024 at 5:14 pmWhat Experts Say You Should Be Able To slot starlight Princess Demo
Window Doctor Near Me
July 13, 2024 at 6:24 pmFive Killer Quora Answers To Window Doctor Near Me
Window Doctor Near Me
www.023456789.xyz
July 13, 2024 at 6:27 pm10 Things We Are Hateful About 10kg Top Loader Washing
Machine http://www.023456789.xyz
Jere Alas
July 13, 2024 at 6:33 pm10 Mobile Apps That Are The Best For Repairs To Double Glazing Jere Alas
oscarreys.top
July 13, 2024 at 6:46 pmEverything You Need To Learn About Who Is Hades To Zeus oscarreys.top
slot Starlight Princess Demo
July 13, 2024 at 6:59 pmStarlight Princess Demo Tips From The Best In The Industry slot Starlight Princess Demo
adhd assessment
July 13, 2024 at 7:02 pmSome Of The Most Common Mistakes People Make With How To Get ADHD Diagnosis adhd assessment
upvc window repairs near me
July 13, 2024 at 7:03 pm5 Laws That Can Benefit The Upvc Window Repairs Industry upvc window repairs near me
https://www.eddafay.top
July 13, 2024 at 7:10 pm15 Gifts For The Kids Bunk Beds Lover In Your Life https://www.eddafay.top
Heidi
July 13, 2024 at 7:21 pmNine Things That Your Parent Teach You About Motorcycle Accident
Lawsuit motorcycle accident (Heidi)
Lynell
July 13, 2024 at 7:59 pmAre You Responsible For An Double Glazing Repair Near Me Budget?
12 Top Notch Ways To Spend Your Money double glazing near me – Lynell,
jerealas.top
July 13, 2024 at 8:20 pmThe Time Has Come To Expand Your Repair Double Glazing Window Options jerealas.top
legal
July 13, 2024 at 9:21 pmThe 12 Most Obnoxious Types Of Accounts You Follow On Twitter legal
https://www.5611432.xyz
July 13, 2024 at 9:24 pm11 Ways To Completely Revamp Your Car Keys Programmer https://www.5611432.xyz
023456789
July 13, 2024 at 9:40 pm10 Real Reasons People Dislike 10kg Load Grade A Washing Machines 10kg Load Grade
A Washing Machines 023456789
upvc windows repairs near me
July 13, 2024 at 9:48 pmYou Are Responsible For The Local Window Repair Budget? 12 Tips On How To Spend Your Money upvc windows repairs near me
telegra.ph
July 13, 2024 at 9:55 pmSee What Repair Upvc Windows Tricks The Celebs Are Using repair upvc windows [telegra.ph]
4452346.xyz
July 13, 2024 at 10:02 pmThe No. 1 Question Anyone Working In Velvet Sectional Sofa Should
Be Able To Answer 4452346.xyz
replacing a Upvc Door lock
July 13, 2024 at 10:32 pmThis Week’s Best Stories About Upvc Windows And Doors Near Me replacing a Upvc Door lock
private adhd assessment liverpool Cost
July 13, 2024 at 10:35 pmThe 10 Most Terrifying Things About Private ADHD Assessment
Liverpool Cost private adhd assessment liverpool Cost
Boat Accident Lawyers
July 13, 2024 at 11:10 pmCheck Out: How Boat Accident Compensation Is Taking Over And What
Can We Do About It Boat Accident Lawyers
fircrest veterans Disability attorney
July 13, 2024 at 11:55 pmFive Laws That Will Aid The Veterans Disability Compensation Industry fircrest veterans Disability attorney
window repair near me
July 14, 2024 at 12:27 am10 Things That Your Family Taught You About Upvc Window Repair Near Me window repair near me
Taylor
July 14, 2024 at 12:27 amWhy Do So Many People Are Attracted To Assessment For Adhd In Adults?
adhd assessment psychiatry uk; Taylor,
Lawyer
July 14, 2024 at 12:38 amThe Top 5 Reasons Why People Are Successful In The Personal Injury Attorneys Industry Lawyer
Private adult Adhd assessment
July 14, 2024 at 1:33 amWhat’s The Current Job Market For Private Adult ADHD Assessment Professionals?
Private adult Adhd assessment
Private Adhd Diagnosis Uk
July 14, 2024 at 2:12 amWhat’s The Job Market For Private ADHD Diagnosis UK Professionals Like?
Private Adhd Diagnosis Uk
https://copeland-hastings-3.blogbright.net/the-story-behind-local-Seo-packages-will-haunt-You-for-the-rest-of-your-life
July 14, 2024 at 2:17 amThe 9 Things Your Parents Teach You About Local SEO Services Uk local seo services uk (https://copeland-hastings-3.blogbright.net/the-story-behind-local-Seo-packages-will-haunt-You-for-the-rest-of-your-life)
installers
July 14, 2024 at 2:57 amFive Things You Don’t Know About Windows Near Me installers
Search Engine Optimisation Services
July 14, 2024 at 2:58 amSearch Engine Optimisation Services Tools To Make Your Daily Life Search Engine Optimisation Services Trick
That Everyone Should Know Search Engine Optimisation Services
www.5611432.xyz
July 14, 2024 at 3:21 am“The Ultimate Cheat Sheet For Key Programming Car http://www.5611432.xyz
upvc repairs Near Me
July 14, 2024 at 3:27 amUpvc Repairs Near Me Tools To Ease Your Daily Life Upvc Repairs Near Me Trick That Every Person Must Learn upvc repairs Near Me
Window Repairs Near Me
July 14, 2024 at 3:35 amThe 10 Most Scariest Things About Double Glazed Window Repairs Near Me Window Repairs Near Me
What is adhd titration
July 14, 2024 at 3:38 amWhat Is ADHD Titration Waiting List? History Of ADHD Titration Waiting List What is adhd titration
upvc repairs near me
July 14, 2024 at 3:50 amWhat’s The Current Job Market For Upvc Repairs Near Me Professionals Like?
upvc repairs near me
repair Upvc window
July 14, 2024 at 4:10 amWhy No One Cares About Upvc Windows Repair repair Upvc window
personal injury attorneys
July 14, 2024 at 4:23 amThe Three Greatest Moments In Personal Injury Attorney History
personal injury attorneys
Annett
July 14, 2024 at 4:31 amDoor Repair Near Me Explained In Fewer Than 140 Characters window replacement near me, Annett,
lynnbolvin.top
July 14, 2024 at 4:34 am10 Failing Answers To Common Wall Mounted Fireplaces
Questions: Do You Know The Right Ones? lynnbolvin.top
gastonia veterans disability Lawyer
July 14, 2024 at 4:53 amHow To Make An Amazing Instagram Video About
Veterans Disability Attorney gastonia veterans disability Lawyer
liability act fela
July 14, 2024 at 5:53 amSee What Employers Liability Act Fela Tricks The Celebs Are
Utilizing liability act fela
https://www.oscarreys.top/d7dl-1cll6m-tx2u2-a8x8-g31c8o9-2094
July 14, 2024 at 6:05 am5. Slot Demo Zeus Hades Rupiah Projects For Any
Budget https://www.oscarreys.top/d7dl-1cll6m-tx2u2-a8x8-g31c8o9-2094
Installer
July 14, 2024 at 6:11 amTen Double Glaze Repair Near Me That Will Actually Help You Live Better Installer
lineyka.org
July 14, 2024 at 6:16 amUpvc Windows Near Me Tools To Ease Your Everyday Lifethe Only Upvc Windows Near Me Trick Every Person Should Learn upvc windows near me (lineyka.org)
Son
July 14, 2024 at 6:31 am15 Best Replacement Lock For Upvc Door Bloggers You
Need To Follow upvc door near me – Son,
veterans disability lawsuits
July 14, 2024 at 6:31 amVeterans Disability Attorney: 10 Things I’d Love To Have Known In The Past veterans disability lawsuits
Private Adhd Assessment Reading
July 14, 2024 at 6:46 amThe 3 Greatest Moments In Private ADHD Diagnosis History Private Adhd Assessment Reading
upvc door Repair near Me
July 14, 2024 at 7:06 amPanels For Upvc Doors’s History History Of Panels
For Upvc Doors upvc door Repair near Me
adult testing For adhd
July 14, 2024 at 7:24 amFive ADHD Tests Lessons From The Pros adult testing For adhd
Accident Lawsuits
July 14, 2024 at 7:27 amHow The 10 Most Disastrous Accident Attorney Mistakes Of All Time Could Have Been Avoided
Accident Lawsuits
Felix Meyer
July 14, 2024 at 7:29 amHurray, this is just the right information that I needed. You make me want to learn more! Stop by my page Webemail24 about Social Media Marketing.
cassylawn.top
July 14, 2024 at 7:44 amA Look At The Future What’s In The Pipeline? Asbestos Exposure Attorney Industry Look Like
In 10 Years? cassylawn.top
Adhd adult test
July 14, 2024 at 7:48 amThe 10 Most Scariest Things About ADHD Adult Test Adhd adult test
what is Titration in adhd
July 14, 2024 at 8:28 amWhy No One Cares About ADHD Titration Waiting
List what is Titration in adhd
telegra.ph
July 14, 2024 at 8:29 am10 Things You’ve Learned About Preschool To Help You Get A Handle On Upvc Windows
Repair repair upvc windows; telegra.ph,
double glazing Upvc windows
July 14, 2024 at 8:41 amUpvc Window Near Me: The Good, The Bad, And The Ugly double glazing Upvc windows
https://highwave.kr
July 14, 2024 at 8:44 amWhy Is Boat Accident Lawyers So Popular? boat accident law firm (https://highwave.kr)
assessment Tools Mental health
July 14, 2024 at 8:46 amHow Adding A Assessment For Mental Health To Your Life Will Make All The Change assessment Tools Mental health
demo slot Sweet zeus
July 14, 2024 at 9:05 amThe Most Common Demo Slot Pragmatic Play Sweet Bonanza Debate
Could Be As Black And White As You May Think demo slot Sweet zeus
Jere Alas
July 14, 2024 at 9:26 am5 Double Glazing Repairs Near Me Myths You Should Avoid Jere Alas
double glazing repairs near me
July 14, 2024 at 9:51 amDouble Glazing Repairs Near Me Techniques To Simplify Your
Everyday Lifethe Only Double Glazing Repairs Near Me Technique Every Person Needs To Be Able To double glazing repairs near me
Compravivienda.com
July 14, 2024 at 9:56 amGuide To Double Glazing Near Me: The Intermediate Guide The Steps
To Double Glazing Near Me double glazing near
me (Compravivienda.com)
railroad Injuries Lawyer
July 14, 2024 at 9:56 amYou’ll Be Unable To Guess Railroad Injuries Lawyers’s Tricks railroad Injuries Lawyer
www.4452346.xyz
July 14, 2024 at 9:59 am20 Myths About Deep Sectional Sofa: Dispelled http://www.4452346.xyz
Personal Injury Attorney
July 14, 2024 at 10:14 amGuide To Personal Injury Attorney: The Intermediate Guide For Personal Injury Attorney
Personal Injury Attorney
https://Espersen-pennington-3.blogbright.net
July 14, 2024 at 10:39 amWhat’s The Job Market For High Wycombe Windows Professionals?
high wycombe windows (https://Espersen-pennington-3.blogbright.net)
Malpractice Lawsuits
July 14, 2024 at 10:48 am10 Key Factors Regarding Compensation You Didn’t Learn At School Malpractice Lawsuits
Boat Accidents
July 14, 2024 at 11:46 amThe Top Reasons Why People Succeed Within The Boat Accident Legal Industry
Boat Accidents
www.kaymell.uk
July 14, 2024 at 11:46 am10 Things Competitors Lean You On Online Slots http://www.kaymell.uk
5611432.xyz
July 14, 2024 at 11:46 amHow To Explain Programing Keys To Your Boss 5611432.xyz
Juanita
July 14, 2024 at 11:51 amWhy You Should Focus On Enhancing Adhd Assessment For Adults how
do adults get assessed for adhd; Juanita,
Upvc Panel Doors
July 14, 2024 at 11:52 amHow To Identify The Upvc External Doors To Be Right For You Upvc Panel Doors
railroad Injuries lawyer
July 14, 2024 at 12:02 pm10 Things That Your Family Taught You About Railroad Injuries
Lawyer railroad Injuries lawyer
medical Malpractice lawyer
July 14, 2024 at 12:07 pmAvoid Making This Fatal Mistake When It Comes To Your Medical Malpractice Attorney medical Malpractice lawyer
upvc bi fold doors
July 14, 2024 at 12:19 pm10 Things Everybody Hates About Upvc Doors Near Me Upvc Doors Near Me upvc bi fold doors
jejucordelia.Com
July 14, 2024 at 12:25 pmWhat Is The Future Of Accident Law Be Like In 100 Years?
accident lawyers (jejucordelia.Com)
Crawley Repairs
July 14, 2024 at 12:44 pmA Step-By-Step Guide To Glass Repair Crawley From Beginning To End
Crawley Repairs
demo Slot hades zeus
July 14, 2024 at 1:11 pmThe 12 Most Popular Demo Slot Hades Accounts To Follow On Twitter demo Slot hades zeus
Upvc Windows Online
July 14, 2024 at 1:21 pmIs There A Place To Research Upvc Windows Online Upvc Windows Online
non prescription adhd medication uk
July 14, 2024 at 1:27 pmThe 10 Most Scariest Things About Non Prescription ADHD
Medication Uk non prescription adhd medication uk
oscarreys
July 14, 2024 at 1:37 pmWhat Is Demo Slot Zeus Hades Rupiah And Why Is Everyone Dissing It?
oscarreys
treat adhd without Medication adults
July 14, 2024 at 1:44 pmThe 12 Most Unpleasant Types Of Natural ADD Treatment Users You
Follow On Twitter treat adhd without Medication adults
Bookmarks4.Men
July 14, 2024 at 2:24 pmHow To Beat Your Boss With Railroad Injuries Attorneys Railroad Injuries Lawsuit (Bookmarks4.Men)
Railroad Injuries Lawyer
July 14, 2024 at 2:32 pm14 Common Misconceptions Concerning Railroad Injuries Law Railroad Injuries Lawyer
www.arlennizo.top
July 14, 2024 at 2:44 pm5 Killer Quora Questions On Collapsible Mobility Scooter http://www.arlennizo.top
https://olderworkers.com.au/
July 14, 2024 at 3:03 pmThe 10 Scariest Things About Upvc Windows Repairs upvc Windows Repair (https://olderworkers.com.au/)
Psychiatrists for adhd near me
July 14, 2024 at 3:43 pm5 Killer Quora Answers On Psychiatrists For Adhd Near Me Psychiatrists for adhd near me
Compact scooters
July 14, 2024 at 4:07 pm“The Compact Folding Scooters Awards: The Most, Worst, And Strangest Things We’ve Ever Seen Compact scooters
Accidents
July 14, 2024 at 4:15 pm15 Things Your Boss Wishes You’d Known About Accident Claim Accidents
www.9363280.xyz
July 14, 2024 at 4:17 pmHow To Explain Asbestos Claims How Much To A 5-Year-Old http://www.9363280.xyz
Upvc windows repairs
July 14, 2024 at 4:33 pmWe’ve Had Enough! 15 Things About Upvc Windows And Doors We’re Sick Of Hearing
Upvc windows repairs
www.oscarreys.top
July 14, 2024 at 4:41 pm10 Ways To Create Your Demo Hades Empire http://www.oscarreys.top
anxiety Psychiatrist near me
July 14, 2024 at 4:53 pmWhat’s The Job Market For Anxiety Psychiatrist Near Me Professionals Like?
anxiety Psychiatrist near me
Upvc Windows Repair
July 14, 2024 at 4:55 pmWhat’s The Job Market For Upvc Windows Repair Professionals?
Upvc Windows Repair
anxiety disorders worksheet
July 14, 2024 at 4:56 pm10 Best Books On Anxiety Disorder Best Medication anxiety disorders worksheet
jerealas.top
July 14, 2024 at 5:15 pmSolutions To Issues With Double Glazing Lock Repairs jerealas.top
replacement double glazed windows
July 14, 2024 at 5:30 pmThis Week’s Best Stories Concerning Replacement Double Glazed Window replacement double glazed windows
Ralph
July 14, 2024 at 6:54 pmDo You Think You’re Suited For Upvc Window Locks?
Check This Quiz wickes upvc windows (Ralph)
double glazing glass replacement cost
July 14, 2024 at 6:55 pmLearn What Double Glazed Windows Near Me Tricks The Celebs Are
Using double glazing glass replacement cost
https://www.eddafay.top/tw0n-2lu-9a60h3f-6dp-z31fxyf-811
July 14, 2024 at 6:55 pmYour Family Will Be Grateful For Getting This Bunk Bed https://www.eddafay.top/tw0n-2lu-9a60h3f-6dp-z31fxyf-811
Malpractice Law Firm
July 14, 2024 at 6:57 pm15 Funny People Working In Malpractice Attorneys In Malpractice Attorneys Malpractice Law Firm
Evan
July 14, 2024 at 7:16 pmTen Taboos About Window Repair Near You Should Never Share
On Twitter window repair near me (Evan)
023456789
July 14, 2024 at 7:43 pm11 Ways To Destroy Your Washing Machines 10kg Capacity 023456789
http://Www.maxtremer.com/bbs/board.php?bo_table=qna_e&wr_id=602354
July 14, 2024 at 7:45 pmHow To Save Money On Accident accident lawsuits
(http://Www.maxtremer.com/bbs/board.php?bo_table=qna_e&wr_id=602354)
window lock Upvc
July 14, 2024 at 8:13 pmWhat Experts On Repair Upvc Windows Want You To Learn window lock Upvc
Motorcycle accident lawyers
July 14, 2024 at 8:40 pmA Step-By Step Guide For Choosing The Right Motorcycle Accident Settlement
Motorcycle accident lawyers
https://minecraftcommand.science/
July 14, 2024 at 8:54 pmAre You Responsible For An Upvc Patio Doors Budget?
10 Fascinating Ways To Spend Your Money letterbox For upvc Door – https://minecraftcommand.science/ –
elsycrays
July 14, 2024 at 9:03 pmCar Locksmiths Near Me Explained In Less Than 140 Characters elsycrays
https://www.9326527.xyz/
July 14, 2024 at 9:05 pmA Help Guide To How To Get Diagnosed With ADHD UK From Start To Finish
https://www.9326527.xyz/
Vickey
July 14, 2024 at 9:08 pmFive Killer Quora Answers On 10kg Laundry Appliance Offers Vickey
Kingranks.Com
July 14, 2024 at 9:40 pmThis Is How Renault Card Key Will Look In 10 Years’ Time Renault
Key Card Repair (Kingranks.Com)
Dermandar.com
July 14, 2024 at 9:40 pmThe 9 Things Your Parents Teach You About Act Fela fela (Dermandar.com)
service
July 14, 2024 at 9:48 pmThis Story Behind Window Repair Near Me Will Haunt You Forever!
service
4452346.xyz
July 14, 2024 at 10:06 pm20 Trailblazers Lead The Way In Sofas Sectionals 4452346.xyz
Motorcycle Accident Lawsuits
July 14, 2024 at 10:20 pm11 “Faux Pas” Which Are Actually Okay To Create With Your Motorcycle Accident Attorney Motorcycle Accident Lawsuits
Adhd assessments for adults
July 14, 2024 at 10:32 pmNine Things That Your Parent Teach You About Adhd Assessments For Adults
Adhd assessments for adults
George
July 14, 2024 at 10:36 pmWhat’s The Current Job Market For Upvc Window Near Me Professionals?
upvc window near me; George,
Private adhd assessment reading
July 14, 2024 at 11:03 pm20 Private ADHD Assessment Belfast Websites That Are Taking The Internet By Storm Private adhd assessment reading
Damaris
July 14, 2024 at 11:12 pmBest Psychiatrists Near Me Techniques To Simplify
Your Everyday Lifethe Only Best Psychiatrists Near Me Trick That Every Person Must Know best psychiatrists
near me (Damaris)
railroad Injuries lawyer
July 14, 2024 at 11:54 pmSee What Railroad Injuries Lawyer Tricks The Celebs Are Using
railroad Injuries lawyer
Federal employers
July 15, 2024 at 12:08 amThis Is How Fela Lawyer Will Look In 10 Years’ Time Federal employers
zackfoxworth
July 15, 2024 at 12:28 amThis Is How Treadmills Folding Will Look In 10 Years’ Time zackfoxworth
upvc windows repair Near me
July 15, 2024 at 12:53 amHow To Outsmart Your Boss On Upvc Window Locks upvc windows repair Near me
Adhd Symptoms Treatment
July 15, 2024 at 2:11 am10 Sites To Help You To Become A Proficient In Treat ADHD Adhd Symptoms Treatment
023456789.xyz
July 15, 2024 at 2:24 am10 10kgs Washing Machine That Are Unexpected 023456789.xyz
kaymell.uk
July 15, 2024 at 3:17 amThe Ultimate Glossary Of Terms For Online Slots kaymell.uk
Fix Car Door Lock Near Me
July 15, 2024 at 3:28 am4 Dirty Little Details About The Car Central Lock
Repair Near Me Industry Fix Car Door Lock Near Me
www.836614.xyz
July 15, 2024 at 4:23 am10 Unexpected Pets Tips Tips http://www.836614.xyz
double glazing units near Me
July 15, 2024 at 4:44 am5 Killer Quora Answers To Replacement Double Glazing Units Near Me
double glazing units near Me
psychiatric assessment form
July 15, 2024 at 4:51 amSee What Psychiatric Assessment Form Tricks The Celebs Are
Utilizing psychiatric assessment form
0270469.xyz
July 15, 2024 at 5:32 am10 Apps That Can Help You Manage Your Asbestos Lung Mesothelioma 0270469.xyz
cassylawn
July 15, 2024 at 5:42 am15 Things You’re Not Sure Of About Asbestos Attorney cassylawn
kaymell
July 15, 2024 at 5:57 am14 Questions You Might Be Insecure To Ask About Slot kaymell
attorney
July 15, 2024 at 6:09 amThe 10 Most Terrifying Things About Railroad Injuries Legal attorney
lawyers
July 15, 2024 at 6:33 amHow To Beat Your Boss On Fela lawyers
023456789
July 15, 2024 at 7:11 amWhere Will Washing Machines 10kg Capacity Be 1 Year From This Year?
023456789
Seoranko
July 15, 2024 at 7:24 amYour site visitors, especially me appreciate the time and effort you have spent to put this information together. Here is my website Seoranko for something more enlightening posts about Social Media Marketing.
Malpractice
July 15, 2024 at 7:38 am9 Lessons Your Parents Teach You About Malpractice Lawyer Malpractice
https://alexander-abernathy.technetbloggers.de/20-things-only-the-most-devoted-cost-to-replace-windows-uk-fans-should-know/
July 15, 2024 at 8:01 am7 Things You’ve Never Learned About Sash Window Replacement window replacement glass (https://alexander-abernathy.technetbloggers.de/20-things-only-the-most-devoted-cost-to-replace-windows-uk-fans-should-know/)
https://www.kaymell.uk/nllr18p-wq6v22h-5yax-hy1njol-0rwmiag-4752/
July 15, 2024 at 8:01 am5 Lessons You Can Learn From Casino Slot https://www.kaymell.uk/nllr18p-wq6v22h-5yax-hy1njol-0rwmiag-4752/
Kay Mell
July 15, 2024 at 8:21 am10 Slots Casino Real Money Tricks All Experts Recommend
Kay Mell
https://buffetwatch2.werite.net
July 15, 2024 at 8:41 amADHD Titration’s History History Of ADHD Titration private adhd medication Titration (https://buffetwatch2.werite.net)
cassylawn
July 15, 2024 at 9:21 amA Look Inside Causes Of Mesothelioma Other Than Asbestos’s Secrets Of Causes
Of Mesothelioma Other Than Asbestos cassylawn
Car Keys Replacement near Me
July 15, 2024 at 9:52 am9 Things Your Parents Taught You About Car Keys Replacement Near Me Car Keys Replacement near Me
Fredericka
July 15, 2024 at 10:31 amSolutions To Problems With Adhd Assessment For Adults adhd assessment liverpool [Fredericka]
adult adhd symptoms Quiz
July 15, 2024 at 11:05 amCould Adhd Symptoms In Women Be The Answer To Achieving 2023?
adult adhd symptoms Quiz
fela claims railroad employees
July 15, 2024 at 11:09 amWhat’s The Job Market For Fela Claims Railroad Employees Professionals Like?
fela claims railroad employees
accident
July 15, 2024 at 12:29 pmWhat’s The Current Job Market For Boat Accident Compensation Professionals?
accident
Https://minecraftcommand.science/Profile/tiewolf94
July 15, 2024 at 12:51 pm10 Key Factors About Adult ADHD Treatment You Didn’t Learn In School treatment For Adhd (https://minecraftcommand.science/Profile/tiewolf94)
www.257634.xyz
July 15, 2024 at 12:56 pmIs Tech Making Repairing Window Better Or Worse? http://www.257634.xyz
Double glazed window repairs near Me
July 15, 2024 at 12:58 pm10 Factors To Know On Window Glass Repairs You Didn’t Learn In The Classroom
Double glazed window repairs near Me
assess adhd
July 15, 2024 at 1:15 pm10 Adhd Assessment Tricks All Experts Recommend assess adhd
www.4452346.xyz
July 15, 2024 at 1:25 pmTen Common Misconceptions About Reclining Sectional Sofa That Aren’t Always True http://www.4452346.xyz
https://Glk-egoza.ru/user/knifegray4
July 15, 2024 at 1:30 pm7 Secrets About Non Stimulant ADHD Medication That Nobody Can Tell You adhd
medication guanfacine (https://Glk-egoza.ru/user/knifegray4)
untreated anxiety
July 15, 2024 at 1:45 pmWhat Makes The Anxiety Attack Treatment So Effective? For COVID-19 untreated anxiety
Upvc repairs near me
July 15, 2024 at 2:01 pmUpvc Repairs Near Me Tools To Help You Manage Your Everyday Lifethe Only Upvc Repairs Near Me Trick That Everybody Should Be Able To Upvc repairs near me
list of adhd symptoms
July 15, 2024 at 2:30 pm20 Quotes That Will Help You Understand Adhd Symptoms In Adult
Women list of adhd symptoms
Toni
July 15, 2024 at 2:46 pmSee What Double Glazing Repairs Near Me Tricks The Celebs Are Making Use Of double
glazing repairs near me (Toni)
full psychiatric assessment
July 15, 2024 at 2:49 pmFull Psychiatric Assessment Tools To Make
Your Daily Lifethe One Full Psychiatric Assessment Technique Every Person Needs To Learn full psychiatric assessment
Santo
July 15, 2024 at 3:16 pmDo Not Make This Blunder With Your Boot Scooter Santo
Normand
July 15, 2024 at 3:24 pm9 Things Your Parents Teach You About Double Glazed Window Suppliers Near Me double glazed window suppliers near me [Normand]
Personal Injury Attorney
July 15, 2024 at 3:27 pmGuide To Personal Injury Attorney: The Intermediate Guide For Personal Injury Attorney Personal Injury Attorney
kaymell.uk
July 15, 2024 at 3:28 pmHow To Tell If You’re Ready For Slot kaymell.uk
private Diagnosis For adhd
July 15, 2024 at 3:30 pmPrivate Diagnosis For ADHD Tips To Relax Your Daily Lifethe One Private Diagnosis For ADHD Trick
Every Person Should Be Able To private Diagnosis For adhd
Elsy Crays
July 15, 2024 at 4:01 pmNew And Innovative Concepts Happening With Local Locksmith For
Cars Elsy Crays
double glazing repairs near me
July 15, 2024 at 4:08 pmWhat’s The Job Market For Double Glazing Repairs Near Me
Professionals Like? double glazing repairs near me
Demo Slot Zeus Empire
July 15, 2024 at 4:23 pm20 Myths About Slot Game Zeus: Debunked Demo Slot Zeus Empire
upvc windows Repairs near me
July 15, 2024 at 4:38 pmWhat Is Upvc Door And Windows’s History? History Of Upvc Door And Windows upvc windows Repairs near me
Www.Cheaperseeker.Com
July 15, 2024 at 4:41 pm15 Unquestionably Reasons To Love Adult ADHD Treatments Treating Adhd – http://Www.Cheaperseeker.Com –
upvc windows for sale
July 15, 2024 at 5:06 pmA Positive Rant Concerning Upvc Window Repair upvc windows for sale
untreated adhd in adults Relationships
July 15, 2024 at 7:18 pm10 Easy Ways To Figure Out The Treating Adult
ADHD In Your Body. untreated adhd in adults Relationships
Shana
July 15, 2024 at 7:39 pmBest ADHD Medication For Adults With Anxiety And
Depression Tools To Improve Your Daily Lifethe One Best ADHD Medication For Adults With Anxiety And Depression Trick That Should Be Used By Everyone Learn best adhd medication for adults with anxiety and depression – Shana –
key fob programming
July 15, 2024 at 8:13 pmWhat Is The Evolution Of Lexus Replacement Key Fob key fob programming
Main akun Demo sweet bonanza
July 15, 2024 at 8:43 pmWhy Sweet Bonanza Candyland Demo Is Your Next Big Obsession Main akun Demo sweet bonanza
occupational health Assessment mental health
July 15, 2024 at 8:59 pmIt’s Enough! 15 Things About Mental Health Assessment
Test We’re Tired Of Hearing occupational health Assessment mental health
Malpractice
July 15, 2024 at 9:08 pmGuide To Malpractice Compensation: The Intermediate Guide For
Malpractice Compensation Malpractice
Vimeo.Com
July 15, 2024 at 10:06 pmThe No. Question That Everyone In Malpractice Lawsuit Should
Be Able To Answer Vimeo.Com
symptoms of Adhd in adult women
July 15, 2024 at 10:52 pm15 Interesting Facts About Adhd In Adults Symptoms That You Didn’t Know symptoms of Adhd in adult women
salisbury Malpractice lawyer
July 16, 2024 at 12:27 amWhat Do You Do To Know If You’re In The Right
Place To Go After Malpractice Lawyer salisbury Malpractice lawyer
lawsuit
July 16, 2024 at 12:37 amResponsible For A Railroad Injuries Claim Budget? Twelve Top Ways To Spend Your Money lawsuit
mental assessment Test
July 16, 2024 at 1:11 amA Guide To Mental Assessments From Beginning To End mental assessment Test
eddafay
July 16, 2024 at 1:25 am12 Stats About Adults Bunk Bed To Make You Seek Out Other People eddafay
utahsyardsale.com
July 16, 2024 at 2:22 amYour Worst Nightmare About Good Psychiatrists Near Me Get Real psychiatrists
near me adhd (utahsyardsale.com)
arlennizo
July 16, 2024 at 2:45 amIts History Of Car Boot Scooter arlennizo
attorney
July 16, 2024 at 4:09 am20 Best Tweets Of All Time About Semi Truck attorney
adrestyt.Ru
July 16, 2024 at 4:46 amThink You’re Cut Out For Locksmith Near Me Auto? Do This Test auto Locksmith service
(adrestyt.Ru)
www.257634.xyz
July 16, 2024 at 5:13 am5 Local Window Repair Projects That Work For Any Budget http://www.257634.xyz
0270469.xyz
July 16, 2024 at 5:40 amTen Things You Need To Be Aware Of Attorneys For Asbestos Exposure 0270469.xyz
lawyer
July 16, 2024 at 6:29 am17 Reasons Why You Shouldn’t Beware Of Veterans Disability Law lawyer
female symptoms of adhd
July 16, 2024 at 7:21 am10 Top Mobile Apps For Adult Adhd Symptoms female symptoms of adhd
what is adhd titration
July 16, 2024 at 7:55 amWhat’s The Current Job Market For What Is ADHD Titration Professionals Like?
what is adhd titration
Private Psychiatrist Assessment
July 16, 2024 at 7:56 amYou’ll Never Guess This Private Psychiatrist Assessment’s Tricks Private Psychiatrist Assessment
medical malpractice lawsuits
July 16, 2024 at 8:28 amMedical Malpractice Lawsuit Tips From The Best In The Business medical malpractice lawsuits
key For mercedes
July 16, 2024 at 8:37 amTen Things You Learned In Kindergarden To Help You Get Started
With Spare Mercedes Key key For mercedes
motorcycle accident attorney
July 16, 2024 at 8:39 amForget Motorcycle Accident Compensation: 10 Reasons Why You Don’t Have It motorcycle accident attorney
adhd assessment for adults what to expect
July 16, 2024 at 8:58 amHow To Beat Your Boss With Private Adult Adhd Assessment adhd assessment for adults what to expect
double Glazed units near me
July 16, 2024 at 9:12 amGuide To Double Glazed Units Near Me: The Intermediate Guide
Towards Double Glazed Units Near Me double Glazed units near me
Private Psychiatrist Wheathampstead
July 16, 2024 at 9:30 amWho Is Responsible For An Private Psychiatrist South Wales
Budget? 12 Best Ways To Spend Your Money Private Psychiatrist Wheathampstead
truck Accident Lawsuit
July 16, 2024 at 10:06 amThe 10 Scariest Things About Truck Accident Attorneys
truck Accident Lawsuit
Adhd in Adults Treatment
July 16, 2024 at 10:38 amSee What ADHD In Adults Treatment Tricks The Celebs Are Utilizing Adhd in Adults Treatment
cost of private psychiatric assessment
July 16, 2024 at 11:42 am20 Resources That Will Make You More Efficient
With Private Psychiatrists cost of private psychiatric assessment
private Adhd assessment ireland Adult
July 16, 2024 at 12:27 pmYour Family Will Be Thankful For Having This Private Assessments For ADHD private Adhd assessment ireland Adult
http://test.gitaransk.Ru/
July 16, 2024 at 12:38 pmThe 9 Things Your Parents Taught You About ADHD Medication Ritalin adhd medication ritalin – http://test.gitaransk.Ru/,
Beryl
July 16, 2024 at 12:47 pm10 Things You Learned In Kindergarden That’ll Help You With Natural ADD Treatment symptoms of adhd in adults and treatment – Beryl –
Https://Imoodle.Win/Wiki/Why_Truck_Accident_Settlement_Is_Everywhere_This_Year
July 16, 2024 at 1:47 pmThe 10 Most Terrifying Things About Truck Accident Attorneys Truck Accident
Attorneys (https://Imoodle.Win/Wiki/Why_Truck_Accident_Settlement_Is_Everywhere_This_Year)
sweet Bonanza free spin Demo
July 16, 2024 at 3:08 pmDon’t Make This Mistake You’re Using Your Slots
Sweet Bonanza sweet Bonanza free spin Demo
srv29897.ht-test.ru
July 16, 2024 at 3:11 pmThis Week’s Top Stories Concerning Private Diagnosis Of ADHD private
adhd assessment newcastle; srv29897.ht-test.ru,
adhd online test
July 16, 2024 at 3:49 pmYou’ll Never Guess This ADHD Online Test’s Tricks adhd online test
Upvc door and windows
July 16, 2024 at 3:55 pmTen Upvc Door And Windows That Really Improve Your Life Upvc door and windows
Separation Anxiety Disorder Therapies
July 16, 2024 at 4:14 pm14 Common Misconceptions Concerning High Functioning Anxiety Disorder Separation Anxiety Disorder Therapies
Julianne
July 16, 2024 at 4:51 pmThe Three Greatest Moments In Double Glazed
Window Near Me History double glazing door handles (Julianne)
vimeo.com
July 16, 2024 at 5:22 pmResponsible For An Veterans Disability Claim Budget?
10 Amazing Ways To Spend Your Money perth amboy veterans
disability lawyer (vimeo.com)
wikimapia.Org
July 16, 2024 at 5:35 pmThis Week’s Most Remarkable Stories About Upvc Replacement Window Handles Window
handle upvc [wikimapia.Org]
Spare Keys Near Me
July 16, 2024 at 7:02 pmA Intermediate Guide To Get A Spare Car Key Made Spare Keys Near Me
how much Is a new mini Key
July 16, 2024 at 11:38 pmOne Of The Most Untrue Advices We’ve Ever Heard About Mini Cooper Key Fob Replacement how much Is a new mini Key
Programming
July 17, 2024 at 12:15 amAudi A4 Car Key Replacement It’s Not As Expensive As You Think
Programming
heavenarticle.com
July 17, 2024 at 2:29 amTen Upvc Window Doctors That Really Help You
Live Better window Doctors [heavenarticle.com]
sash window repair
July 17, 2024 at 3:06 amBuzzwords De-Buzzed: 10 Different Ways Of Saying Double Glazing Doctor sash window repair
Carmel
July 17, 2024 at 4:12 amThe 10 Most Scariest Things About Upvc Door Doctor upvc door doctor (Carmel)
Leeds glazing
July 17, 2024 at 10:34 pm7 Simple Secrets To Totally You Into Door Fitters Leeds
Leeds glazing
asbestos Attorney
July 18, 2024 at 12:30 amMesothelioma is a form of cancer that affects the
thin layer of tissue that covers organs in the body.
It is typically caused by exposure to asbestos Attorney, a substance that is used in a variety
of industrial applications.
glamorouslengths.com
July 18, 2024 at 2:56 pmWindows Doctor Tools To Make Your Daily Lifethe One Windows Doctor Trick That Every
Person Must Learn windows doctor (glamorouslengths.com)
Tamie
July 19, 2024 at 4:46 amWhere Will Tier 2 Seo One Year From Now? single tier vs multi tier architecture (Tamie)
malpractice lawsuits
July 19, 2024 at 4:53 am7 Things You’ve Always Don’t Know About Malpractice Settlement malpractice lawsuits
Archie
July 19, 2024 at 5:35 amUpvc Window Repairs Near Me Tools To Help You Manage Your Daily Life Upvc Window Repairs Near Me Trick Every Individual Should Be Able
To upvc window repairs near me; Archie,
mazda Keyless Remote
July 19, 2024 at 6:28 amIt’s A Mazda 2 Key Success Story You’ll Never Remember mazda Keyless Remote
malpractice Attorney
July 19, 2024 at 8:52 amWhat’s The Current Job Market For Malpractice Attorney Professionals?
malpractice Attorney
Mesothelioma Claims
July 19, 2024 at 9:06 amMesothelioma Tools To Simplify Your Daily Life Mesothelioma Claims
www.349338.xyz
July 19, 2024 at 9:28 amThere Is No Doubt That You Require Multi Fuel Stoves For Sale http://www.349338.xyz
upvc door Fittings
July 19, 2024 at 11:16 am5 Killer Queora Answers On Upvc Windows And Doors Near Me
upvc door Fittings
Medical malpractice Lawsuit
July 19, 2024 at 3:50 pmResponsible For A Medical Malpractice Legal Budget? 12 Top Ways To Spend Your Money Medical malpractice Lawsuit
https://championsleage.review
July 19, 2024 at 6:02 pmIt Is The History Of Double Glazed Door Repairs Near Me
In 10 Milestones double Glaxing (https://championsleage.review)
0270469
July 19, 2024 at 6:35 pmWhat’s The Current Job Market For Asbestos And Mesothelioma Professionals Like?
0270469
nelson-kure-2.blogbright.net
July 19, 2024 at 6:53 pmGuide To Double Glazed Window Near Me: The Intermediate Guide For Double Glazed Window Near Me double glazed Window near me (nelson-kure-2.blogbright.net)
https://willysforsale.com
July 19, 2024 at 7:30 pmThe 9 Things Your Parents Taught You About Cream Sectional Sofa sectional sofa [https://willysforsale.com]
How To Get Chain Skewer Hades
July 19, 2024 at 7:53 pmWhat’s The Current Job Market For How To Get Chain Skewer Hades Professionals Like?
How To Get Chain Skewer Hades
www.36035372.xyz
July 19, 2024 at 8:22 pmA Reference To American Fridge Frezzers From Beginning To End http://www.36035372.xyz
Single gb stroller
July 19, 2024 at 8:44 pm10 Failing Answers To Common Single Stroller Pushchair Questions Do You Know Which Ones?
Single gb stroller
Angelina
July 19, 2024 at 8:48 pmMedical Malpractice Lawyers Tips To Relax Your Everyday Lifethe Only Medical Malpractice Lawyers Trick That Everybody Should
Be Able To medical malpractice lawyer (Angelina)
http://jejucordelia.com/eng/bbs/board.php?bo_table=review_e&wr_id=264085
July 19, 2024 at 9:39 pmThe Top Retro Fridge Freezer Gurus Do 3 Things retro american style fridge freezer – http://jejucordelia.com/eng/bbs/board.php?bo_table=review_e&wr_id=264085 –
new lexus Key
July 19, 2024 at 9:46 pm5 Killer Quora Answers To New Lexus Key new lexus Key
Upvcwindows
July 19, 2024 at 9:57 pmUpvc Window Handle Replacement: 11 Thing You’ve Forgotten To
Do Upvcwindows
Malpractice Lawsuit
July 19, 2024 at 9:59 pmSee What Malpractice Lawsuit Tricks The Celebs Are Utilizing Malpractice Lawsuit
https://www.257634.xyz/4ne5tsk-ax558u-8xnvv1q-68t43-r8igt-241
July 19, 2024 at 10:01 pm15 Strange Hobbies That Will Make You Smarter At Window Repair Near https://www.257634.xyz/4ne5tsk-ax558u-8xnvv1q-68t43-r8igt-241
Prams Travel System
July 20, 2024 at 1:32 amGuide To Prams Travel System: The Intermediate Guide The Steps To Prams Travel System Prams Travel System
semi truck accident attorney
July 20, 2024 at 1:34 amYour Family Will Be Grateful For Getting This Semi Truck Claim
semi truck accident attorney
Https://Bookmarkfeeds.Stream
July 20, 2024 at 2:14 amDo You Know How To Explain Railroad Injuries Law To
Your Boss Railroad Injuries Lawyers (https://Bookmarkfeeds.Stream)
Oscar Reys
July 20, 2024 at 2:26 amThe Largest Issue That Comes With Demo Hades, And How You Can Solve It Oscar Reys
Starlight princess demo
July 20, 2024 at 3:13 amDemo Slot Starlight Princess X1000 Strategies That Will Change
Your Life Starlight princess demo
Marti
July 20, 2024 at 3:39 am10 Tell-Tale Warning Signs You Need To Buy A Upvc Windows And Doors upvc window repairs – Marti –
private adhd assessment wirral
July 20, 2024 at 4:55 am10 Reasons You’ll Need To Be Educated About Private ADHD Diagnosis UK
Cost private adhd assessment wirral
double glazed units near me
July 20, 2024 at 5:05 amGuide To Double Glazed Units Near Me: The Intermediate Guide Towards Double Glazed Units Near Me double glazed units near me
Upvc Double Doors
July 20, 2024 at 9:54 amYour Worst Nightmare About Replacement Lock For Upvc
Door Come To Life Upvc Double Doors
high functioning adhd symptoms
July 20, 2024 at 10:12 am5 Killer Quora Questions On Symptoms Of Adhd Adults Test high functioning adhd symptoms
fitting
July 20, 2024 at 10:25 amResponsible For The Double Glazing Supplies Near Me Budget?
10 Wonderful Ways To Spend Your Money fitting
car key maker near me
July 20, 2024 at 10:50 amThe Reasons To Focus On Enhancing Replacement Mini Cooper Key car key maker near me
023456789.xyz
July 20, 2024 at 11:04 amWhat Experts In The Field Of 10kg Capacity Washing Machine Want You To
Be Able To 023456789.xyz
Personal injury
July 20, 2024 at 12:04 pm5 Killer Quora Answers To Personal Injury Attorneys Personal injury
upvc window Repair near Me
July 20, 2024 at 12:19 pmYou’ll Never Guess This Upvc Window Repair Near Me’s Benefits upvc window Repair near Me
Window Repairs Near Me
July 20, 2024 at 1:27 pmSee What Window Repairs Near Me Tricks The Celebs Are Making Use Of Window Repairs Near Me
Double glazing companies near Me
July 20, 2024 at 2:19 pmThe 10 Most Terrifying Things About Double Glazing Companies Near
Me Double glazing companies near Me
Demo Slot Pragmatic Lengkap
July 20, 2024 at 2:26 pm20 Trailblazers Lead The Way In Slot Demo Demo Slot Pragmatic Lengkap
double glazing company near me
July 20, 2024 at 3:43 pmWatch Out: How Double Glazing Near Me Is Taking Over And How To
Respond double glazing company near me
Https://Telegra.Ph/
July 20, 2024 at 3:58 pmA Peek Inside Assessing Adhd In Adults’s Secrets Of Assessing Adhd In Adults Adhd In Adults Assessment; https://Telegra.Ph/,
Personal Injury Lawyers
July 20, 2024 at 4:02 pm8 Tips To Increase Your Personal Injury Case Game Personal Injury Lawyers
peatix.com
July 20, 2024 at 4:39 pmFive Killer Quora Answers On Treadmills For Home UK treadmills for home
uk; peatix.com,
best fold away Treadmill
July 20, 2024 at 4:42 pm15 Best Fold Away Treadmill Bloggers You Should Follow best fold away Treadmill
4452346
July 20, 2024 at 9:03 pmWhy Is Most Comfortable Sectional Sofa So Popular?
4452346
Mervin
July 20, 2024 at 9:41 pmThe Mesothelioma Asbestosis Mistake That Every Beginner Makes Mervin
land rover Lr4 key fob programming
July 20, 2024 at 10:34 pmThen You’ve Found Your Land Rover Key Fob … Now What?
land rover Lr4 key fob programming
lock upvc door
July 20, 2024 at 10:37 pmTen Stereotypes About Upvc Door Panel Replacement That Aren’t Always
True lock upvc door
injuries
July 20, 2024 at 10:56 pmA. The Most Common Federal Employers Debate Doesn’t Have
To Be As Black And White As You Might Think injuries
window Repairs near me
July 20, 2024 at 11:16 pm5 Must-Know-How-To-Hmphash Double Glazed Window
Repair Methods To 2023 window Repairs near me
Window Repairs Near Me
July 20, 2024 at 11:31 pm10 Tell-Tale Symptoms You Need To Get A New Upvc Window Repair Near Me
Window Repairs Near Me
Comprehensive Psychiatric assessment
July 20, 2024 at 11:51 pm15 Private Psychiatrist Assessment Near Me Benefits Everyone Should Know Comprehensive Psychiatric assessment
435871
July 21, 2024 at 12:38 am3 Ways In Which The 3 Wheel Compact Stroller Can Influence Your Life
435871
Antoinette
July 21, 2024 at 1:27 amWhy Upvc Windows And Doors Near Me Is Relevant 2023 upvc windows for sale (Antoinette)
Railroad Injuries Law Firm
July 21, 2024 at 1:37 am11 “Faux Pas” That Are Actually Acceptable To Create Using
Your Railroad Injuries Compensation Railroad Injuries Law Firm
injury
July 21, 2024 at 1:40 amWhat’s The Job Market For Personal Injury Attorney
Professionals Like? injury
Double glasing
July 21, 2024 at 3:27 amWhy Do So Many People Would Like To Learn More About Double Glazing Doors Near Me?
Double glasing
https://riley-santana.thoughtlanes.net
July 21, 2024 at 4:18 amThe 10 Scariest Things About Federal Employers
Liability Act employers’ Liability act fela – https://riley-santana.thoughtlanes.net,
jerealas
July 21, 2024 at 4:32 amWhy Everyone Is Talking About Misted Double
Glazing Repairs Right Now jerealas
Windows Double Glazed
July 21, 2024 at 5:17 amAll The Details Of Double Glazed Front Doors Near Me Dos And Don’ts Windows Double Glazed
Darin
July 21, 2024 at 5:28 amWood Stoves It’s Not As Hard As You Think Darin
double glazing companies near me
July 21, 2024 at 5:57 amThe 10 Most Scariest Things About Double Glazing Companies Near Me double glazing companies near me
Double Glazinf
July 21, 2024 at 6:05 am7 Essential Tips For Making The Greatest Use Of Your Double
Glazing Units Near Me Double Glazinf
replacement nissan qashqai key
July 21, 2024 at 6:45 amA Intermediate Guide To Nissan Juke Key Replacement replacement nissan qashqai key
6699101.xyz
July 21, 2024 at 9:12 am5 Must-Know Buying A Second Hand Mobility Scooter-Practices You Need To Know For
2023 6699101.xyz
Birth Injury Lawsuits
July 21, 2024 at 11:03 amThe People Who Are Closest To Birth Injury Case Uncover Big Secrets Birth Injury Lawsuits
9779342
July 21, 2024 at 11:06 am5 Laws That Anyone Working In Best Oil Filled Radiator Should Be Aware Of 9779342
zackfoxworth.top
July 21, 2024 at 11:57 amThis Is The New Big Thing In Treadmill That Folds Up zackfoxworth.top
Personal Injury Lawsuit
July 21, 2024 at 12:06 pmThe Reasons You Should Experience Personal Injury Settlement At
Least Once In Your Lifetime Personal Injury Lawsuit
screwfix Upvc Windows
July 21, 2024 at 12:14 pmTen Upvc Window-Related Stumbling Blocks You Shouldn’t Share On Twitter
screwfix Upvc Windows
Nikole
July 21, 2024 at 12:35 pm15 Things You’re Not Sure Of About Mesothelioma Asbestos Nikole
upvc door repairs near me
July 21, 2024 at 12:43 pmThe 10 Scariest Things About Upvc Door Repairs Near Me
upvc door repairs near me
federal railroad
July 21, 2024 at 1:38 pmA Time-Travelling Journey The Conversations People Had About Fela Lawyer
20 Years Ago federal railroad
residential window repairs
July 21, 2024 at 2:01 pm10 Things We We Hate About Luton Double Glazing residential window repairs
arlennizo
July 21, 2024 at 2:14 pmWhat’s The Reason Boot Mobility Scooters Is Fast
Becoming The Hottest Trend Of 2023 arlennizo
https://rollcrib91.bravejournal.net
July 21, 2024 at 2:35 pmIs Upvc Door And Window The Greatest Thing There Ever Was?
upvc window (https://rollcrib91.bravejournal.net)
emplois.fhpmco.fr
July 21, 2024 at 2:58 pmThe Next Big Trend In The Window Repair Near Industry upvc
Window repair, emplois.fhpmco.fr,
023456789
July 21, 2024 at 4:26 pmThe Reasons Why Adding A 10kg Washing Machine Deals To Your
Life Can Make All The An Impact 023456789
Double Glazing Door Repair
July 21, 2024 at 4:51 pm20 Tips To Help You Be More Successful At Crawley Door And
Window Double Glazing Door Repair
Letterbox for upvc doors
July 21, 2024 at 4:53 pmUpvc Doors Repair: It’s Not As Difficult As You Think Letterbox for upvc doors
www.36035372.xyz
July 21, 2024 at 5:20 pm10 Things You Learned In Kindergarden That’ll Help You With Integral Fridge
http://www.36035372.xyz
Donte
July 21, 2024 at 7:21 pm10 Things Everyone Gets Wrong About The Word “Double Glazing Glass Replacement Near Me.” double glazed window locks (Donte)
auto fold mobility scooter uk
July 21, 2024 at 7:38 pm10 Life Lessons We Can Learn From Auto Fold Mobility Scooter auto fold mobility scooter uk
913875
July 21, 2024 at 8:23 pmThis Is The Complete Listing Of Modern Wood Burning Stove Dos And Don’ts 913875
https://posteezy.com/it-history-glass-doctor-near-me
July 21, 2024 at 8:33 pmWhat NOT To Do In The Window Doctor Near Me Industry the window doctor (https://posteezy.com/it-history-glass-doctor-near-me)
Window Glass Replacements
July 21, 2024 at 8:53 pmReplacement Glass For Windows: What’s The Only Thing
Nobody Is Talking About Window Glass Replacements
upvc Door Repairs near me
July 21, 2024 at 9:15 pm15 Shocking Facts About Door Repairs Near Me That You Never Knew upvc Door Repairs near me
lineyka.org
July 21, 2024 at 9:44 pm7 Things You’ve Never Learned About Adhd
In Adults Symptoms Female adhd symptoms list – lineyka.org,
Car diagnostics mobile
July 21, 2024 at 9:46 pmWhy Do So Many People Want To Know About Mobile Mechanic Near Me?
Car diagnostics mobile
Seat Keys Replacement
July 21, 2024 at 9:57 pmSeat Keys Replacement Tools To Streamline Your Everyday Lifethe Only Seat Keys
Replacement Trick That Should Be Used By Everyone Learn Seat Keys Replacement
햇살론 무직자 대출
July 21, 2024 at 10:28 pmHow You’re A Financial Information With A $10,000 Limit In 5 Steps 햇살론 무직자 대출
www.arlennizo.top
July 22, 2024 at 12:18 amWhat To Do To Determine If You’re Set For Car Boot Mobility Scooter http://www.arlennizo.top
railroad injuries lawyer
July 22, 2024 at 12:53 am9 Lessons Your Parents Taught You About Railroad Injuries Lawyer railroad injuries lawyer
Floy
July 22, 2024 at 1:20 am11 Strategies To Completely Redesign Your Wall Mount Fireplace Floy
Csgo Cases
July 22, 2024 at 2:04 amThe 9 Things Your Parents Teach You About CSGO Cases
How To Get Csgo Cases
Cassy Lawn
July 22, 2024 at 2:25 amThe Secret Secrets Of Asbestos Mesothelioma Life
Expectancy Cassy Lawn
257634
July 22, 2024 at 3:56 am20 Inspiring Quotes About Repairs To Upvc Windows
257634
www.9326527.xyz
July 22, 2024 at 4:25 amThe Unspoken Secrets Of How To Get An ADHD Diagnosis http://www.9326527.xyz
0270469.xyz
July 22, 2024 at 6:37 amWhere Do You Think Mesothelioma And Asbestos Be
1 Year From Right Now? 0270469.xyz
counter-strike cases
July 22, 2024 at 7:19 am10 Tell-Tale Signals You Should Know To Buy A Best CSGO Opening
Site counter-strike cases
www.913875.xyz
July 22, 2024 at 7:35 amThe Reasons Wood Burning Stoves Is Everywhere This Year http://www.913875.xyz
jerealas
July 22, 2024 at 8:16 amWhy Glazing Repair Doesn’t Matter To Anyone jerealas
replacement double glazing units near Me
July 22, 2024 at 8:20 amFive Killer Quora Answers To Replacement Double Glazing Units Near Me replacement double glazing units near Me
Wendy
July 22, 2024 at 8:32 am5. Akun Slot Demo Projects For Any Budget Wendy
elsycrays.top
July 22, 2024 at 8:46 am15 Amazing Facts About Car Lock Smith That You Didn’t Know About elsycrays.top
Arlen Nizo
July 22, 2024 at 8:54 amThe Unspoken Secrets Of Boot Mobility Scooters Arlen Nizo
Lynn Bolvin
July 22, 2024 at 9:07 amBio Ethanol Fireplace Tools To Improve Your Everyday Lifethe Only Bio
Ethanol Fireplace Trick That Every Person Should Know Lynn Bolvin
www.836614.xyz
July 22, 2024 at 9:21 amWhich Website To Research Designer Handbags On Sale Online http://www.836614.xyz
Erika
July 22, 2024 at 9:36 amThe Top 5 Reasons People Thrive In The Fireplace Industry Erika
timber
July 22, 2024 at 9:54 amBe On The Lookout For: How Double Glazing Near Me Is Taking Over And What You Can Do About It timber
Oscar Reys
July 22, 2024 at 10:20 am10 Facts About How To Unlock Zeus Heart Hades That Will Instantly Put You In The
Best Mood Oscar Reys
0270469
July 22, 2024 at 10:54 am15 Secretly Funny People Work In Asbestos Exposure Mesothelioma 0270469
9326527.xyz
July 22, 2024 at 11:13 amThe Top Reasons Why People Succeed In The Getting A Diagnosis For ADHD Industry
9326527.xyz
https://www.4182051.xyz
July 22, 2024 at 11:38 amA Look At The Good And Bad About Coffee Machines https://www.4182051.xyz
Jere Alas
July 22, 2024 at 12:02 pm15 Weird Hobbies That Will Make You More Effective At Double Glazing
Repair Jere Alas
Iesha
July 22, 2024 at 12:16 pmThe Reason Behind Tier Links Has Become The Obsession Of Everyone In 2023 tiered link (Iesha)
Sandy
July 22, 2024 at 12:31 pmNine Things That Your Parent Teach You About Window Doctor Near Me window doctor near me (Sandy)
Double glazing repairs Near me
July 22, 2024 at 12:51 pmWhat’s The Job Market For Double Glazing Repairs Near Me Professionals?
Double glazing repairs Near me
notabug.Org
July 22, 2024 at 1:20 pmGuide To Federal Employers: The Intermediate Guide
To Federal Employers Federal employers (notabug.Org)
double Glazed window Repairs
July 22, 2024 at 1:20 pmWhat’s The Job Market For Double Glazed Window Repairs Professionals?
double Glazed window Repairs
elsycrays
July 22, 2024 at 1:57 pm10 Best Mobile Apps For Locksmith Car Key elsycrays
Double glazing near me
July 22, 2024 at 2:06 pm9 . What Your Parents Taught You About Double
Glazing Repair Near Me Double glazing near me
upvc door doctor near Me
July 22, 2024 at 2:18 pmYou’ll Never Be Able To Figure Out This Upvc Door Doctor Near
Me’s Benefits upvc door doctor near Me
railroad injuries attorney
July 22, 2024 at 2:26 pmGet Rid Of Railroad Injuries Compensation: 10 Reasons Why You Don’t Have It railroad injuries attorney
double Glazing windows
July 22, 2024 at 5:41 pmYou’ll Be Unable To Guess Double Glazing Windows Near Me’s Tricks double Glazing windows
Upvc Windows Near Me
July 22, 2024 at 5:51 pmGuide To Upvc Windows Near Me: The Intermediate Guide In Upvc Windows
Near Me Upvc Windows Near Me
Railroad Injuries lawyers
July 22, 2024 at 5:59 pmA Railroad Injuries Law Success Story You’ll Never Be Able To Railroad Injuries lawyers
Treatment for anxiety symptoms
July 22, 2024 at 6:58 pmTen Things You Learned In Kindergarden That Will Help You Get Treatment Anxiety Treatment for anxiety symptoms
Jere Alas
July 22, 2024 at 8:17 pmHow Double Glazing Condensation Repair Cost Became The
Hottest Trend Of 2023 Jere Alas
Fela claims Railroad employees
July 22, 2024 at 9:42 pmWhat’s The Job Market For Fela Claims Railroad Employees Professionals?
Fela claims Railroad employees
https://www.arlennizo.top/
July 22, 2024 at 9:49 pmThe Three Greatest Moments In Car Boot Scooter History https://www.arlennizo.top/
Fela Railroad
July 22, 2024 at 9:52 pm5 Killer Quora Answers To Fela Railroad Fela Railroad
http://web018.dmonster.kr/bbs/board.php?bo_Table=b0601&wr_id=723389
July 22, 2024 at 9:53 pmWhat’s The Current Job Market For Window Repair Near Me Professionals Like?
window repair near me (http://web018.dmonster.kr/bbs/board.php?bo_Table=b0601&wr_id=723389)
personal injury attorney
July 22, 2024 at 9:56 pm5 People You Should Meet In The Personal Injury Law Industry personal injury attorney
Volvo Small key Fob
July 22, 2024 at 10:00 pm10 Tips For Getting The Most Value From Volvo C30 Key
Volvo Small key Fob
Lynn Bolvin
July 22, 2024 at 10:09 pmThe Little Known Benefits Of Wall Mounted Fireplace Lynn Bolvin
Cassy Lawn
July 22, 2024 at 10:48 pmAre You Getting The Most The Use Of Your Mesothelioma Asbestos Lung Cancer?
Cassy Lawn
257634.xyz
July 22, 2024 at 10:58 pmA Vibrant Rant About Window Repair 257634.xyz
Edda Fay
July 22, 2024 at 11:18 pm17 Reasons Why You Should Ignore Kids Bunk Beds Edda Fay
kaymell.uk
July 22, 2024 at 11:45 pmWhat Is Slot And Why Is Everyone Speakin’ About It?
kaymell.uk
attorney
July 23, 2024 at 12:31 amWhat Is Railroad Injuries Case? How To Make Use Of It attorney
4182051
July 23, 2024 at 1:45 amWhy Coffee Filter Machine Should Be Your Next Big Obsession? 4182051
Max
July 23, 2024 at 1:48 am4 Dirty Little Tips On Replacement Window Glass Near Me Industry Replacement Window Glass Near Me Industry
replacement of window glass; Max,
window repairs near me
July 23, 2024 at 1:53 amUpvc Window Repairs Near Me Tools To Streamline Your Daily Lifethe One Upvc Window Repairs Near Me
Trick That Every Person Must Know window repairs near me
Semi truck accident Law Firm
July 23, 2024 at 2:21 am15 Shocking Facts About Semi Truck Compensation That You
Never Knew Semi truck accident Law Firm
oscarreys
July 23, 2024 at 2:43 am11 Ways To Completely Redesign Your Akun Demo Hades oscarreys
구글 검색엔진최적화
July 23, 2024 at 2:45 am3 Guidelines To Make Your Google Adwords Traffic More Effective 구글 검색엔진최적화
double glazed glass Replacement
July 23, 2024 at 2:45 amDon’t Stop! 15 Things About Double Glazing Shops Near
Me We’re Sick Of Hearing double glazed glass Replacement
Window repair Near me
July 23, 2024 at 2:50 amWhat Is Upvc Repairs Near Me And Why Are We Speakin’ About It?
Window repair Near me
Aja
July 23, 2024 at 3:13 amDon’t Buy Into These “Trends” Concerning Glass Window Repairs broken glass repair
(Aja)
fela accident attorney
July 23, 2024 at 3:28 amGuide To Fela Accident Attorney: The Intermediate Guide The Steps To Fela Accident Attorney
fela accident attorney
Double Glazed Window Suppliers Near Me
July 23, 2024 at 3:53 amNine Things That Your Parent Taught You About Double Glazed Window Suppliers Near
Me Double Glazed Window Suppliers Near Me
Mesothelioma Legal
July 23, 2024 at 4:10 amWhat’s The Current Job Market For Mesothelioma Legal Question Professionals
Like? Mesothelioma Legal
http://srv29897.ht-test.ru/index.php?subaction=userinfo&user=kevinresult1
July 23, 2024 at 4:12 am14 Questions You Shouldn’t Be Uneasy To Ask Window Repair Near Me window repairs near me (http://srv29897.ht-test.ru/index.php?subaction=userinfo&user=kevinresult1)
railroad injuries lawsuits
July 23, 2024 at 5:07 am15 Of The Best Twitter Accounts To Learn More About Railroad Injuries Attorneys railroad injuries lawsuits
Lexus ct200h key replacement cost
July 23, 2024 at 6:19 amNine Things That Your Parent Teach You About Lexus Ct200h Key
Replacement Cost Lexus ct200h key replacement cost
Https://emplois.fhpmco.fr/author/feetcornet06/
July 23, 2024 at 7:03 am13 Things You Should Know About Best Truck Accident Attorney
That You Might Not Have Known lawyer truck accident – https://emplois.fhpmco.fr/author/feetcornet06/ –
Double Glazed Window Suppliers Near Me
July 23, 2024 at 7:37 am10 Things That Your Family Teach You About Double Glazed Window Suppliers Near Me Double Glazed Window Suppliers Near Me
36035372.xyz
July 23, 2024 at 7:50 amThe Most Innovative Things That Are Happening With American-Style Fridge 36035372.xyz
Tempaste.Com
July 23, 2024 at 8:02 amNine Things That Your Parent Taught You About Personal Injury Lawyer Personal Injury
(Tempaste.Com)
elsycrays.top
July 23, 2024 at 8:17 am7 Tricks To Help Make The Most Out Of Your Carlock Smith elsycrays.top
American Fridge Freezer Deals
July 23, 2024 at 8:23 amWhy You Should Concentrate On Enhancing American Fridge Freezers American Fridge Freezer Deals
durable mobility scooters
July 23, 2024 at 9:05 amThe 10 Most Scariest Things About Durable Mobility Scooters durable mobility scooters
personal Injury
July 23, 2024 at 11:32 amWhat’s The Job Market For Personal Injury Compensation Professionals?
personal Injury
vauxhall insignia replacement key fob
July 23, 2024 at 11:33 am16 Facebook Pages You Must Follow For Vauxhall Insignia Key Replacement-Related Businesses vauxhall insignia replacement key fob
Rochester Truck Accident Lawyers
July 23, 2024 at 11:50 amTruck Crash Lawyer Tips That Can Change Your Life Rochester Truck Accident Lawyers
Kathrin
July 23, 2024 at 11:53 amAsk Me Anything: 10 Answers To Your Questions About Play
Slots Online Kathrin
https://Compravivienda.com/author/officetray8/
July 23, 2024 at 12:32 pmWhat Is It That Makes Citroen Berlingo Key Fob So Popular?
citroen c1 key replacement price (https://Compravivienda.com/author/officetray8/)
36035372.xyz
July 23, 2024 at 12:53 pmWhy American Side By Side Fridge Freezer Is Tougher Than You Imagine
36035372.xyz
zackfoxworth.top
July 23, 2024 at 1:37 pm10 Things Everybody Hates About American Freezer Fridge
zackfoxworth.top
diagnostic
July 23, 2024 at 2:16 pmSee What Average Cost Of Car Diagnostic Uk Tricks The Celebs Are Making Use Of diagnostic
전세자금 대출
July 23, 2024 at 2:36 pmTop 10 Free Bank Holiday Weekend Ideas 전세자금 대출
personal injury Lawsuits
July 23, 2024 at 3:58 pmTen Myths About Personal Injury Case That Aren’t Always The Truth personal injury Lawsuits
volkswagen keys Made
July 23, 2024 at 4:39 pmFive Things You’re Not Sure About About Volkswagen Key Replacement volkswagen keys Made
injuries
July 23, 2024 at 5:16 pm5 Killer Quora Answers On Railroad Injuries Law injuries
Skoda car keys
July 23, 2024 at 6:57 pm10 Startups That Will Change The Skoda Fabia
Key Replacement Industry For The Better Skoda car keys
Soila
July 23, 2024 at 7:33 pmYou’ve Forgotten Vauxhall Astra Key Fob Replacement:
10 Reasons Why You Don’t Really Need It vauxhall zafira key fob (Soila)
cahill-mullins-4.technetbloggers.de
July 23, 2024 at 7:51 pmThe Reasons To Work With This Adhd Symptoms In Women adhd inattentive type symptoms (cahill-mullins-4.technetbloggers.de)
www.cassylawn.top
July 23, 2024 at 8:08 pmFive People You Should Know In The Asbestos Mesothelioma Industry http://www.cassylawn.top
https://www.elsycrays.top/y30i1c0-d5p8-nz87pi-ky0-b11q0yx-2030/
July 23, 2024 at 8:19 pmThe Most Profound Problems In Local Car Locksmith
https://www.elsycrays.top/y30i1c0-d5p8-nz87pi-ky0-b11q0yx-2030/
asbestos law
July 23, 2024 at 8:45 pmAffected individuals will need an asbestos law
lawyer to help them hold companies accountable for
their exposure. Many times, the companies knew about the link between mesothelioma and asbestos but did not take responsibility.
Fela Lawyers
July 23, 2024 at 10:00 pmTen Fela Lawyers That Really Help You Live Better Fela Lawyers
Jere Alas
July 24, 2024 at 12:30 amNew And Innovative Concepts Happening With Double Glazing Door Repairs Near Me Jere Alas
https://www.elsycrays.top
July 24, 2024 at 1:24 amThe Reasons You’re Not Successing At Locksmiths Car https://www.elsycrays.top
www.kaymell.uk
July 24, 2024 at 1:42 am15 Play Slots Online Benefits You Should All Know http://www.kaymell.uk
다바오 보너스코드
July 24, 2024 at 3:08 amFast Poor Credit Loan – Some Important Questions 다바오 보너스코드
when zeus was born
July 24, 2024 at 4:01 amThe Ultimate Guide To Demo Slot Zeus when zeus was born
https://wikimapia.org/
July 24, 2024 at 5:04 amHow To Create An Awesome Instagram Video About Double Glazed
Window Replacement Near Me repair double glazing (https://wikimapia.org/)
Moneyasia2024visitorview.Coconnex.com
July 24, 2024 at 7:51 amHow To Explain 3 Wheeled Pushchair To Your Mom 3 wheel all Terrain buggy (Moneyasia2024visitorview.Coconnex.com)
Oscar Reys
July 24, 2024 at 7:58 amIt Is The History Of Demo Slot Zeus Vs Hades Pragmatic In 10
Milestones Oscar Reys
locks for upvc doors
July 24, 2024 at 7:59 amHow To Outsmart Your Boss In Upvc Door Locking Mechanism locks for upvc doors
Federal Railroad
July 24, 2024 at 8:51 amFive Killer Quora Answers To Federal Railroad Federal Railroad
Double glazing near Me
July 24, 2024 at 9:24 amGuide To Double Glazing Near Me: The Intermediate Guide Towards Double Glazing Near Me Double glazing near Me
lynnbolvin
July 24, 2024 at 9:50 am10 Healthy Habits To Use Wall Mounted Electric Fireplace lynnbolvin
cassylawn
July 24, 2024 at 10:51 am15 Gifts For The Mesothelioma Asbestos Lover In Your Life cassylawn
Fela Lawyer
July 24, 2024 at 11:47 am20 Quotes Of Wisdom About Fela Railroad Fela Lawyer
duplicate mini Cooper key
July 24, 2024 at 12:12 pmWhat Is The Heck Is Replacement Mini Keys?
duplicate mini Cooper key
window Repair near me
July 24, 2024 at 12:23 pm10 Upvc Repairs Near Me Tips All Experts Recommend window Repair near me
design21.Net
July 24, 2024 at 12:37 pmYou’ll Never Guess This 18 Wheeler Accident Law Firm’s Secrets 18 wheeler accident law firm (design21.Net)
Jayme
July 24, 2024 at 12:44 pm15 Best Pinterest Boards Of All Time About Car Boot Scooters
Jayme
emplois.fhpmco.fr
July 24, 2024 at 1:23 pmSeven Reasons Why Upvc Windows And Doors Is Important replacing upvc door locks (emplois.fhpmco.fr)
cassylawn
July 24, 2024 at 2:20 pm5 Lessons You Can Learn From Mesothelioma Asbestos cassylawn
double Glaze units
July 24, 2024 at 2:25 pm7 Things You’ve Never Known About Double Glazing Companies Near Me double Glaze units
elsycrays
July 24, 2024 at 2:42 pmKey Smith For Cars Explained In Fewer Than 140 Characters elsycrays
eddafay.top
July 24, 2024 at 2:53 pmWhat You Must Forget About Making Improvements To Your Bunk Bed Price eddafay.top
medication given for adhd
July 24, 2024 at 3:35 pm10 Facts About Medication For ADHD And Anxiety That Will Instantly Put You In A Good Mood medication given for adhd
repair upvc windows
July 24, 2024 at 4:48 pm7 Small Changes That Will Make A Big Difference With Your Upvc Windows Repair repair upvc windows
The Double Glazing Doctor
July 24, 2024 at 5:04 pm5 Laws To Help The Double Glazing Doctor Near Me Industry The Double Glazing Doctor
Porsche Immobilizer Repair
July 24, 2024 at 5:05 pmA Intermediate Guide The Steps To Porsche Key Shell Replacement Porsche Immobilizer Repair
Botdb.Win
July 24, 2024 at 5:23 pmThe Most Underrated Companies To Keep An Eye On In The Double
Glazing Repairs Bristol Industry Bifold Door Repairs Near Me [Botdb.Win]
upvc handle Window
July 24, 2024 at 5:33 pmHow Much Do Upvc Windows Experts Earn? upvc handle Window
Lemuel
July 24, 2024 at 5:43 pmGuide To Shopping Online In Uk For Products: The Intermediate Guide To Shopping Online In Uk For Products shopping online in uk for
products (Lemuel)
Over The Counter Adhd Medication For Adults
July 24, 2024 at 5:54 pmDon’t Make This Silly Mistake With Your Best ADHD
Medication For Adults Over The Counter Adhd Medication For Adults
Oscar Reys
July 24, 2024 at 5:56 pmHow To Outsmart Your Boss On Hades Zeus Slot Demo Oscar Reys
Can You Order Stuff Online
July 24, 2024 at 7:20 pmWhat’s The Job Market For Can You Order Stuff Online Professionals Like?
Can You Order Stuff Online
0270469
July 24, 2024 at 7:30 pmAsbestos Exposure Mesothelioma Explained In Less Than 140 Characters 0270469
Aida
July 24, 2024 at 7:37 pmA Look In The Secrets Of Demo Slot pragmatic play demo rupiah (Aida)
Landon
July 24, 2024 at 7:48 pmAmazon Best Sellers Products Tools To Help You Manage Your Daily Lifethe One Amazon Best Sellers Products Trick That Every
Person Must Know amazon best sellers products – Landon,
학생 대출
July 24, 2024 at 7:57 pmCan You Open A Bank Account With A Bad History 학생 대출
Double glazing units near me
July 24, 2024 at 8:12 pm5 Killer Quora Answers On Replacement Double Glazing Units Near Me
Double glazing units near me
Upvc Patio Doors
July 24, 2024 at 8:49 pmUpvc Door Locking Mechanism: What’s New? No One Has Discussed Upvc Patio Doors
Window Repairs Near Me
July 24, 2024 at 9:38 pmNine Things That Your Parent Taught You About
Upvc Window Repairs Near Me Window Repairs Near Me
구글상위노출 대행사
July 24, 2024 at 9:43 pmHow To Focus On The Best Internet Advertising Search Engine Optimization Guide Book?
구글상위노출 대행사
Tst.Ezmir.Co.Kr
July 24, 2024 at 10:32 pm20 Great Tweets Of All Time Concerning Semi Truck Semi Truck Accident
Law Firm (Tst.Ezmir.Co.Kr)
Peugeot Partner Key Fob
July 24, 2024 at 11:03 pmHow To Save Money On Peugeot Key Replacement Near Me Peugeot Partner Key Fob
lawsuit
July 24, 2024 at 11:48 pmThe Worst Advice We’ve Heard About Federal Employers Liability Act lawsuit
Renault Key Replacement Cost
July 25, 2024 at 12:11 am10 Renault Megane Key Card Replacement Related Projects To Expand Your Creativity Renault Key Replacement Cost
san Francisco truck accident Lawyer
July 25, 2024 at 12:14 am14 Creative Ways To Spend Extra Truck Crash Attorney Budget san Francisco truck accident Lawyer
Replacement Window Near Me
July 25, 2024 at 12:41 am11 Ways To Fully Defy Your Window Pane Replacement Replacement Window Near Me
search engine optimisation Manchester
July 25, 2024 at 12:42 amYou’ve Forgotten Seo Optimization: 10 Reasons Why You Don’t Have It search engine optimisation Manchester
Fridgefreezers16393.Bloggip.Com
July 25, 2024 at 12:58 amThe 9 Things Your Parents Teach You About American Style Fridge
Freezers American Style Fridge Freezer (Fridgefreezers16393.Bloggip.Com)
Drusilla
July 25, 2024 at 1:05 amYour Family Will Be Thankful For Getting This Volvo Key
Replacement volvo key programming near me (Drusilla)
railroad injuries Lawsuits
July 25, 2024 at 1:12 amA Productive Rant Concerning Railroad Injuries Attorneys railroad injuries Lawsuits
Lg fridge freezers Uk
July 25, 2024 at 1:30 am20 Rising Stars To Watch In The Large Chest Freezer Uk Industry Lg fridge freezers Uk
replacement units for Double glazing
July 25, 2024 at 4:04 amBlown Double Glazing Repairs Near Me Explained In Fewer Than 140 Characters replacement units for Double glazing
window doctor near me
July 25, 2024 at 4:44 amWhat’s The Job Market For Window Doctor Near Me Professionals?
window doctor near me
double glaze repair near me
July 25, 2024 at 6:33 amYou’ll Be Unable To Guess Double Glaze Repair Near Me’s Tricks double glaze repair near me
에볼루션 양방조회
July 25, 2024 at 7:34 amHow To Get To The Top Of The Marketing Food Chain 에볼루션 양방조회
다바오 설치방법
July 25, 2024 at 12:35 pmPoker Tools Are Ideal For Anyone Deal With 다바오 설치방법
다바오 시티
July 25, 2024 at 6:44 pmWhat Their Bank Won’t Cover Mortgage Refinancing 다바오 시티
프라그마틱 무료체험
July 26, 2024 at 2:54 pmPoker Strategies And Tips – The Right Way To Win Poker Games 프라그마틱 무료체험
Edda Fay
July 27, 2024 at 2:36 am14 Savvy Ways To Spend Leftover Kids Bunk Bed Budget Edda Fay
다바오 특급 호텔
July 27, 2024 at 3:58 amThe Benefit Of Poker Tables With A Table Top 다바오 특급 호텔
Www.5097533.xyz
July 27, 2024 at 6:33 amHow Much Can Treatments For Anxiety Disorders Experts Earn? http://Www.5097533.xyz