What is Web Storage in HTML5?
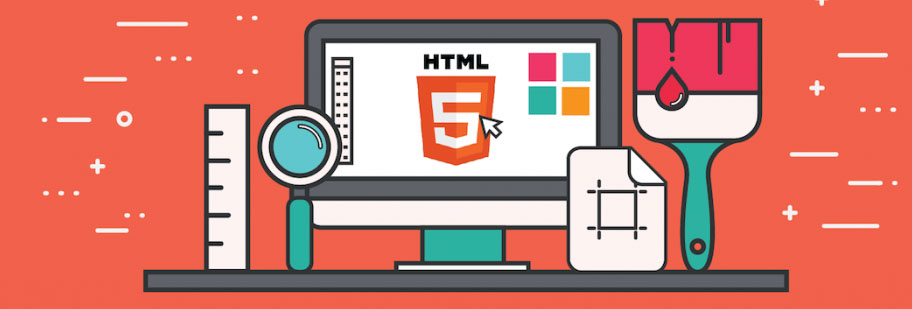
The HTML5’s web storage feature lets you store some information locally on the user’s computer, similar to cookies. Web storage is more better option than the cookies, as per security is concern both are same.
The information stored in the web storage isn’t sent to the web server as opposed to the cookies. Cookies let you store a small amount of data (nearly 4KB), the web storage allows you to store up to 5MB of data and you can also increase it.
There are two types of web storage:
- Local Storage
- Session Storage
Local storage:
The local storage uses the localStorage is built in object provided by HTML 5 to store data for your entire website on a permanent basis. That means the stored local data will be available on the next day, the next week, or the next year unless you remove it.
e.g. Write data using html 5.
<html>
<head>
<title> storage </title>
</head>
<body>
Enter Name: <input type="text" id="nm" /> <br>
Enter Email ID:<input type="email" id="em" /> <br>
Mobile No:<input type="mobile" id="mb" /> <br>
<input type="button" value="write" onclick="writedt()"/>
<script type="text/javascript">
function writedt()
{
var nm = document.getElementById("nm").value;
var em= document.getElementById("em").value;
var mb = document.getElementById("mb").value;
if(typeof(Storage)!="undefined")
{
localStorage.UserName= nm;
localStorage.EmailID = em;
localStorage.MobileNo=mb;
alert("Write Successfull!");
location.href="storageread.html";
}
else
{
alert("Browser do not support storage!");
}
}
</script>
</body>
</html>
Session storage:
The session storage uses the sessionStorage built in object to store data on a temporary basis, for a single browser window or tab. The data disappears when session ends i.e. when the user closes that browser window or tab.
<html>
<head>
<title> read </title>
</head>
<body>
Name: <span id="nm"> </span> <br>
Email ID:<span id="em"></span> <br>
Mobile No: <span id="mb"> </span> <br>
<input type="button" value="read" onclick="readdt()"/>
<script type="text/javascript">
function readdt() {
if(typeof(Storage)!="undefined")
{
document.getElementById("nm").innerText = localStorage.UserName;
document.getElementById("em").innerText=localStorage.EmailID;
document.getElementById("mb").innerText=localStorage.MobileNo;
}
else {
alert("Browser do not support storage!");
}
}
</script>
</body>
</html>
In this article, we learned about web storage, in next article we will try to focus on web workers in html 5.