What is Url Routing? How it works in ASP.NET MVC 5?
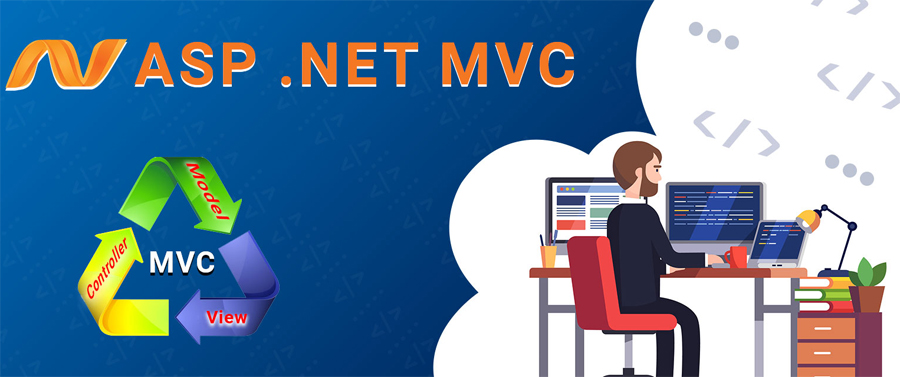
Example Link : https://drive.google.com/file/d/1LdPfsfpnRy0BytOUCaLJnauN6_9hRpnR/view
The ASP.NET Routing module/Route Engine is responsible for mapping incoming browser requests to particular MVC controller actions. When you create a new ASP.NET MVC application, the application is already configured to use ASP.NET Routing. ASP.NET MVC uses Route Table for routing and Route table contains entires/recors called route and every route is composed of url pattern and handling mechnism or code.
How Routing Works?
In simple word, we can say that ASP.NET MVC Routing is a pattern matching mechanism that handles the incoming request (i.e. incoming URL) and figures out what to do with that incoming request (i.e. incoming URL). At runtime, Routing engine uses the Route table for matching the incoming request’s URL pattern against the URL patterns defined in the Route table.
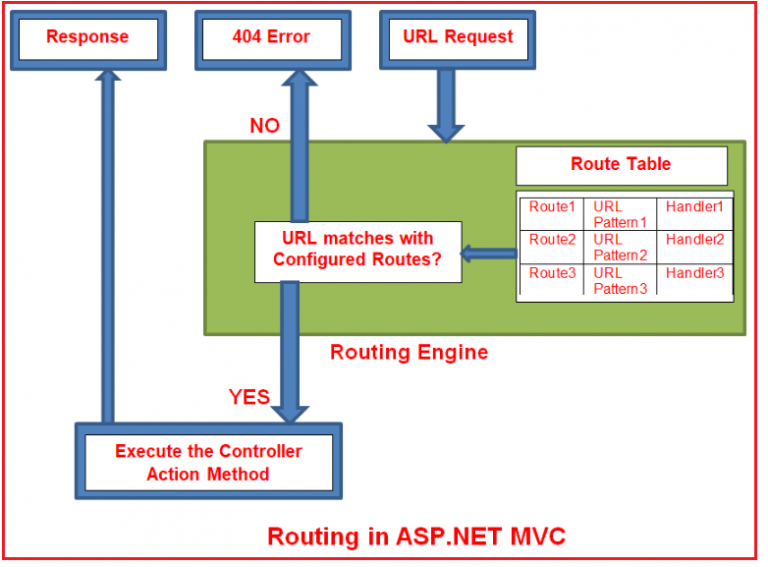
ASP.NET Routing is setup a route table in the application’s Global.asax file. The Global.asax file is a special file that contains event handlers for ASP.NET application lifecycle events. The route table is created during the Application Start event.
namespace MVCFirstApp {
public class MvcApplication : System.Web.HttpApplication {
protected void Application_Start(){
AreaRegistration.RegisterAllAreas();
RouteConfig.RegisterRoutes(RouteTable.Routes);
}
}
}
Following is the implementation of RouteConfig class, which contains one method RegisterRoutes.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Routing;
namespace MVCFirstApp {
public class RouteConfig {
public static void RegisterRoutes(RouteCollection routes){
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new{ controller = "Home", action = "Index", id = UrlParameter.Optional});
}
}
}
As you can see in the above code, the Routing is configured using the MapRoute() extension method of RouteCollection class, where the Route name is “Default” and the URL pattern is “{controller}/{action}/{id}“. The Defaults value for the controller is Home, and the default action method is Index and the id parameter is optional.
Example to understand Routing:
http://localhost:53605/ => controller = Home, action = Index, id = none, since default value of controller and action are Home and Index respectively.
http://localhost:53605/Home => controller = Home, action = Index, id = none, since default value of action is Index
http://localhost:53605/Home/Index => controller = Home, action = Index, id=none
http://localhost:53605/Home/Index/5 => controller = Home, action = Index, id = 5
Creating Custom Routes in ASP.NET MVC Application:
You can configure your custom route using the MapRoute method of route collection. You need to provide at least two parameters in MapRoute function i.e. route name and URL pattern. The Default parameter is optional.
You can register multiple custom routes with different names. Consider the following example where we register the “Employee” route.
namespace FirstMVCDemo
{
public class RouteConfig
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Employee",
url: " Employee/{id}",
defaults: new { controller = "Employee", action = "Index" }
);
routes.MapRoute(
name: "Default", //Route Name
url: "{controller}/{action}/{id}", //Route Pattern
defaults: new
{
controller = "Home", //Controller Name
action = "Index", //Action method Name
id = UrlParameter.Optional //Defaut value for above defined parameter
}
);
}
}
}
So, in this way you can configure as many as routes you want with your own URL pattern in ASP.NET MVC Application. Let’s add Employee Controller to our application
namespace FirstMVCDemo.Controllers
{
public class EmployeeController : Controller
{
public ActionResult Index()
{
return View();
}
}
}
Note: More specific routes should be registered before the genera route, otherwise route engine will map all request to general route and specific route will not be executed.