What is TagHelper? How to use it in ASP.NET Core (MVC)?
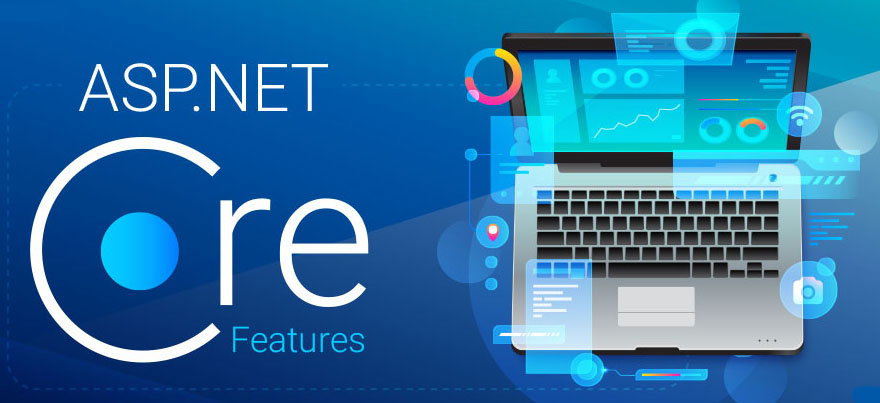
Example Code : https://drive.google.com/file/d/1w6b5R_9liDJLVKLEnCqGLUR3D8Y_-2q5/view?usp=sharing
In this article we will talk about tag helpers and how to use the tag helpers in ASP.NET MVC Core.
What is Tag Helper?
Tag Helpers enable server-side code to participate in creating and rendering HTML elements in Razor files. Tag helpers can be tags or set of attributes those can be applied on html tags can be used in razor view.
Tag helpers are a new feature and similar to HTML helpers, which help us render HTML Tags.A Tag Helpers allows you to conditionally modify or add HTML elements from server-side code. Tag Helpers are authored in C#, and they target HTML elements based on the element name, the attribute name, or the parent tag.
An HTML-friendly development experience since the tag helpers just add few attributes to existing html tag to generate or add some thing in html tag using server code. If you are familiar with HTML Helpers, Tag Helpers reduce the explicit transitions between HTML and C# in Razor views.
ASP.NET Core provides you many built in tag helpers like –Anchor Tag Helper, Form Tag Helpers etc.
You can also create your own tag helper or custom tag helper.
Why Tag Helpers?
A rich IntelliSense environment for creating HTML and Razor markup
A way to make you more productive and able to produce more robust, reliable, and maintainable code using information only available on the server
Installing Tag Helpers
Tag Helpers are by default not available in empty ASP .NET Core project you need to install it using NuGet package manager. We can install it using NuGet graphical interface or using following command –
Install Microsoft.AspNetCore.Mvc.TagHelpers
Managing Tag Helper scope
Tag Helpers scope is controlled by a combination of @addTagHelper, @removeTagHelper directives.
@addTagHelper makes Tag Helpers available, to make the availiable globally to all the views we can add it to _ViewImports.cshtml file as follows –
@addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers
If you want to add a tag helper to specific view only you can also add this common in begining of the view then that tag helper will be availiable to that view only.
@removeTagHelper removes Tag Helpers
The @removeTagHelper has the same two parameters as @addTagHelper, and it removes a Tag Helper that was previously added. For example, @removeTagHelper applied to a specific view removes the specified Tag Helper from the view.
Few built in tag helpers
ASP.NET Core Razor provides you many built in tag helpers few of them are –
- Anchor Tag Helper
- Form Tag Helper
- Input Tag Helper
- Label Tag Helper
- Link Tag Helper
- TextArea Tag Helper
- Select Tag Helper
- Validation Message Tag Helper
- Validation Summary Tag Helper
Anchor Tag Helper:
The Anchor Tag Helper enhances the standard HTML anchor () tag by adding new attributes. By convention, the attribute names are prefixed with asp-. The rendered anchor element’s href attribute value is determined by the values of the asp- attributes.
asp-controller & asp-action
The asp-controller & ap-action attribute assigns the controller and action used for generating the URL. The following markup lists all speakers:
<a asp-controller="Speaker"
asp-action="Index">All Speakers</a>
if no controller and action specified it will use default controller and default action.
asp-route-{value}
The asp-route-{value} attribute enables a wildcard route prefix. Any value occupying the {value} placeholder is interpreted as a potential route parameter. If a default route isn’t found, this route prefix is appended to the generated href attribute as a query string parameter and value.
<a asp-controller="Speaker"
asp-action="Detail"
asp-route-id="@Model.SpeakerId">SpeakerId: @Model.SpeakerId</a>
Form Tag Helper
Generates the HTML action attribute value for a MVC controller action or named route
Generates a hidden Request Verification Token to prevent cross-site request forgery (when used with the [ValidateAntiForgeryToken] attribute in the HTTP Post action method)
asp-controller and asp-action
allow you to specify the name of controller and action where you want to submit the form.
<form asp-controller="Demo" asp-action="Register" method="post">
<!-- Input and Submit elements -->
</form>
The Form Tag Helper above generates the following HTML:
<form method="post" action="/Demo/Register">
<!-- Input and Submit elements -->
<input name="__RequestVerificationToken" type="hidden" value="<removed for brevity>">
</form>
Label Tag Helper
Generates the label caption and for attribute on a element for an expression name. asp-for attribute when applied on a label will generate the label for the model property passed as parameter.
@model SimpleViewModel
<form asp-controller="Demo" asp-action="RegisterLabel" method="post">
<label asp-for="Email"></label>
<input asp-for="Email" /> <br />
</form>
The following HTML is generated for the element:
<label for="Email">Email Address</label>
Input Tag Helper
The Input Tag Helper binds an HTML element to a model expression in your razor view.
<input asp-for="<Expression Name>">
Generates the id and name HTML attributes for the expression name specified in the asp-for attribute. asp-for=”Property1.Property2″ is equivalent to m => m.Property1.Property2. The name of the expression is what is used for the asp-for attribute value.
Sets the HTML type attribute value based on the model type and data annotation attributes applied to the model property
Won’t overwrite the HTML type attribute value when one is specified
Generates HTML5 validation attributes from data annotation attributes applied to model properties
The Input
Tag Helper sets the HTML type
attribute based on the .NET type. The following table lists some common .NET types and generated HTML type (not every .NET type is listed).
.NET type | Input Type |
---|---|
Bool | type=”checkbox” |
String | type=”text” |
DateTime | type=“datetime-local” |
Byte | type=”number” |
Int | type=”number” |
Single, Double | type=”number” |
The following table shows some common data annotations attributes that the input tag helper will map to specific input types (not every validation attribute is listed):
Attribute | Input Type |
---|---|
[EmailAddress] | type=”email” |
[Url] | type=”url” |
[HiddenInput] | type=”hidden” |
[Phone] | type=”tel” |
[DataType(DataType.Password)] | type=”password” |
[DataType(DataType.Date)] | type=”date” |
[DataType(DataType.Time)] | type=”time” |
e.g. Model class
using System.ComponentModel.DataAnnotations;
namespace FormsTagHelper.ViewModels
{
public class RegisterViewModel
{
[Required]
[EmailAddress]
[Display(Name = "Email Address")]
public string Email { get; set; }
[Required]
[DataType(DataType.Password)]
public string Password { get; set; }
}
}
View Code:
@model RegisterViewModel
<form asp-controller="Demo" asp-action="RegisterInput" method="post">
Email: <input asp-for="Email" /> <br />
Password: <input asp-for="Password" /><br />
<button type="submit">Register</button>
</form>
HTML Generated
<form method="post" action="/Demo/RegisterInput">
Email:
<input type="email" data-val="true"
data-val-email="The Email Address field is not a valid email address."
data-val-required="The Email Address field is required."
id="Email" name="Email" value=""><br>
Password:
<input type="password" data-val="true"
data-val-required="The Password field is required."
id="Password" name="Password"><br>
<button type="submit">Register</button>
<input name="__RequestVerificationToken" type="hidden" value="<removed for brevity>">
</form>
Textarea Tag Helper
The Textarea Tag Helper tag helper is similar to the Input Tag Helper.
Generates the id and name attributes, and the data validation attributes from the model for a element. Provides strong typing.<br /> HTML Helper alternative: Html.TextAreaFor</p>
<form asp-controller="Demo" asp-action="RegisterTextArea" method="post">
<textarea asp-for="Description"></textarea>
<button type="submit">Test</button>
</form>
HTML Generated will be –
<form method="post" action="/Demo/RegisterTextArea">
<textarea id="Description" name="Description">
</textarea>
<button type="submit">Test</button>
<input name="__RequestVerificationToken" type="hidden" value="<removed for brevity>">
</form>
Select Tag Helper
The role of the select tag helper is to render an HTML select element populated with options generated from a collection of SelectListItem objects, enumeration and/or options set via the option tag helper.
asp-for: The property on the PageModel that represents the selected element(s)
asp-items: A collection of SelectListItem objects, a SelectList object or an enumeration that provide the options for the select list.
This helper is alternative Html.DropDownListFor and Html.ListBoxFor
Where item can be list of SelectListItem in Model or ViewBag and ViewData. You can also load the items from Enum into select tag.
e.g. Loading from Model property
<select asp-for="Country" asp-items="Model.Countries"> </select>
e.g. Loading from ViewBag
<select asp-for="Country" asp-items="ViewBag.Countries"> </select>
Load Options from Enum
It’s often convenient to use with an enum property and generate the SelectListItem elements from the enum values.
public enum CountryEnum {
India,
USA,
Canada,
France,
Germany,
Spain
}
public class CountryEnumViewModel {
public CountryEnum EnumCountry { get; set; }
}
<select asp-for="EnumCountry" asp-items="Html.GetEnumSelectList<CountryEnum>()">
</select>