What is .NET Core?
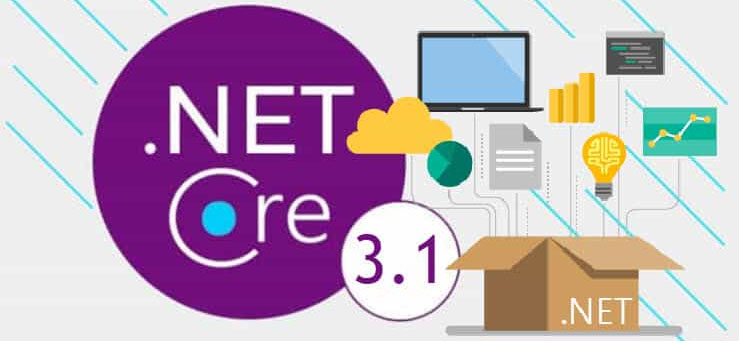
What is .NET Core?
.NET Core is an open-source, general-purpose development platform. You can create .NET Core apps for Windows, macOS, and Linux for x64, x86, ARM32, and ARM64 processors using multiple programming languages. .NET Core and APIs are provided for cloud, IoT, client UI, and machine learning.
The project is primarily developed by Microsoft and released under the MIT License.
Why .NET Core?
Following are the popular features due to which .NET Core is getting popular –
- Cross Platform
- Open Source
- Modern
- Performance
- Consistent Across Environments
- Command Line Interface (CLI)
- Flexible Deployment
.NET Core is Cross Platform
.NET Core is designed from the scratch by Microsoft to support the multiple platforms. Currently .NEt Core supports / Runs on Windows, macOS & maximum implementation of Linux.
.NET Core is Open Source
Yes, .NET Core is open source, using MIT and Apache 2 licenses. .NET Core is a .NET Foundation project. What is means? it mean greater ecosystem, more libraries , more contributors to core framework, but do not worry Microsoft fully support it.
.NET Core is Modern
Over a time field of software / application development got very new stack of technologies and need to variety of types of application for variety of devices and integration. Keeping in fact in mind the Microsoft had lot of modern API’s in .net Core few of them are asynchronous programming, resource governance for containers like docker. It provides you API’s to work with Could, IOT & Mobile Apps.
.NET Core is High Performing
.NET Core developed from scratch to deliver high performance with features like hardware intrinsic, tiered compilation.
.NET Core is Consistent Across Platforms
.NET Core is desingned to runs your code with the same behavior on multiple operating systems & processor architectures e.g. x64, x86, & ARM.
.NET Core – Command Line Interface (CLI)
.NET Core has it’s own command line interface. It is easy-to-use command-line tools can be used for local development & continuous integration.
.NET Core Support Flexible Deployment.
.NET Core support flexible deployment.You can include .NET Core in your app or install it side-by-side (user-wide or system-wide installations). Can be used with Docker containers, we can say containers are the virtual OS running on top of the hardware.
.NET Core Languages & Editors
The C#, Visual Basic, and F# languages can be used to write applications and libraries for .NET Core. These languages can be used in your favorite text editor or Integrated Development Environment (IDE), including:
- Visual Studio
- Visual Studio Code
API’s Supported by .NET Core
.NET Core is designed to support following major API’s.
- Cloud apps with ASP.NET Core
- Mobile apps with Xamarin
- IoT apps with System.Device.GPIO
- Windows client apps with WPF and Windows Forms
- Machine learning ML.NET
Install .Net Core
You can install .NET Core by two ways:
- By installing Visual Studio 2017/2019 :- to download the visual studio you can use the link. https://visualstudio.microsoft.com/downloads/
- Installing .NET Core Runtime or SDK:- to download the .net core SDK you can use link. https://dotnet.microsoft.com/download
.NET Core installer already contains ASP.NET Core libraries, so there is no separate installer for ASP.NET Core. .NET Core 2.1 and .NET Core 3.1 is having long term support. Visual Studio 2017 supports .NET Core 2.1, whereas Visual Studio 2019 supports both the versions.
.NET Core CLI Commands
.NET Core comes with Command Line Interface which allow you to create , build, debug and run the .net applications from the command prompt.
Few CLI commands
All CLI commands starts with dotnet e.g. dotnet new/build/restore etc.
new:- is used to create new application
restore:- is used to restore the packages.
build:- will compile and built the .net core application
publish:- will publish the app on web server.
run:- run the .net core application
clean:- is used to clean the .net core project.
help:- get help about the cli command
You can create a new application using dotnet command as follows –
D:\> dotnet new template -n applicationname
where template can be –
Templates | Short name | Language | Tags | Introduced |
---|---|---|---|---|
Console Application | console | [C#], F#, VB | Common/Console | 1.0 |
Class library | classlib | [C#], F#, VB | Common/Library | 1.0 |
WPF Application | wpf | [C#] | Common/WPF | 3.0 |
WPF Class library | wpflib | [C#] | Common/WPF | 3.0 |
WPF Custom Control Library | wpfcustomcontrollib | [C#] | Common/WPF | 3.0 |
WPF User Control Library | wpfusercontrollib | [C#] | Common/WPF | 3.0 |
Windows Forms (WinForms) Application | winforms | [C#] | Common/WinForms | 3.0 |
Windows Forms (WinForms) Class library | winformslib | [C#] | Common/WinForms | 3.0 |
Let us create a new console application as follows –
d:\> dotnet new console -n addition
To open the project using VsCode editor go to addition folder and type a command code. , it will open the project in vs code.
d:\>cd addition
d:\addition\>code .
It will create the console application with program.cs file modify it as follows –
using System;
namespace Addition
{
class Program
{
static void Main(string[] args)
{
int a, b, c;
Console.Write("Enter Value for A:");
a = Convert.ToInt32(Console.ReadLine());
Console.Write("Enter Value for B:");
b = Convert.ToInt32(Console.ReadLine());
c = a + b;
Console.WriteLine("Result is:" + c);
}
}
}
To run the application switch to addition folder, since app will be get created in application folder and use the following command –
d:\> dotnet run
To create a web application use following command –
d:\>dotnet new web -n firstweb app
To run this application go to app folder since it will create empty project in app folder, then use following command to run the application.
d:\>dotnet run