What is Database First Approach in Entity Framework?
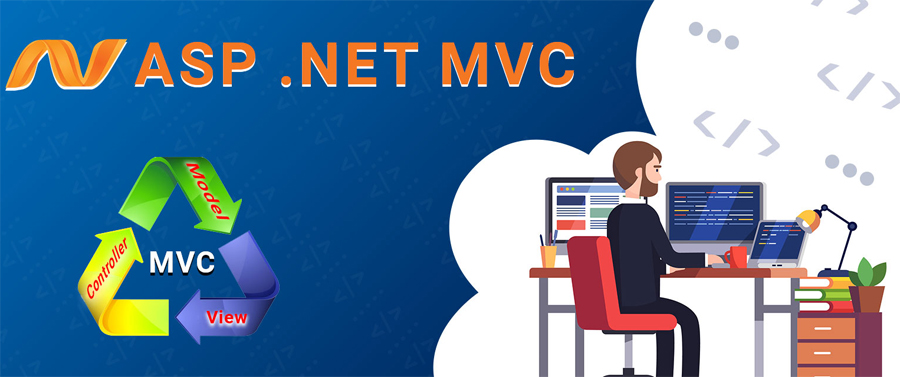
Example Code: https://drive.google.com/file/d/1laZVt6zyzVNx8KmR0xL2TnbZj3wNlNJQ/view?usp=sharing
In the Database First approach, we need to have an existing database and create a a business model from which we can use the Database first approach. Before starting directly with developing in MVC, let’s start with Exploring Database first.
- Database first approach is used when a database is ready; then Entity Framework will complete its duty and create Plain C# Objects entities for you.
- If you already had a designed database and you don’t want to do extra efforts, then you can go with this approach.
- You can modify the database manually and update the model from a database.
- So, we can say, entity framework can create your model classes based on tables and columns from the relational database.

Step by Step Process: –
Create a new ASP.NET Web Application as previously we create.
We’re going to make use of Entity Framework Designer, which is included as part of Visual Studio, to create our model.
Project -> Add New Item…
Select Data from the left menu and then ADO.NET Entity Data Model
Enter BloggingModel as the name and click OK
This launches the Entity Data Model Wizard
Select Generate from Database and click Next

Select Data from the left menu and then ADO.NET Entity Data Model.

Enter BookStore as the name and click Add. This launches the Entity Data Model Wizard. Select EF Designer from Database and click Next.


Click the checkbox next to Tables you want to import and click Finish.
Now let’s look at the new DbContext class.
//------------------------------------------------------------------------------
// <auto-generated>
// This code was generated from a template.
//
// Manual changes to this file may cause unexpected behavior in your application.
// Manual changes to this file will be overwritten if the code is regenerated.
// </auto-generated>
//------------------------------------------------------------------------------
namespace DatabaseFirst
{
using System;
using System.Data.Entity;
using System.Data.Entity.Infrastructure;
public partial class BookContext : DbContext
{
public BookContext()
: base("name=BookContext")
{
}
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
throw new UnintentionalCodeFirstException();
}
public virtual DbSet<Book> Books { get; set; }
}
}
Update Model From Database
To update model from the database, right-click the .edmx file and select Update Model from Database.

Expand the Tables, Views, and Stored Procedures nodes, and check the objects you want to add to the .edmx file.

Click Finish to update the .edmx file with the database changes.

The context class is a most important class while working with EF 6 or EF Core. It represent a session with the underlying database using which you can perform CRUD (Create, Read, Update, Delete) operations.
The context class in Entity Framework is a class which derives from System.Data.Entity.DbContextDbContext in EF 6 and EF Core both. An instance of the context class represents Unit Of Work and Repository patterns wherein it can combine multiple changes under a single database transaction.
The context class is used to query or save data to the database. It is also used to configure domain classes, database related mappings, change tracking settings, caching, transaction etc.