What is ASP.NET Core?

ASP.NET is a popular web-development framework for building web apps on the .NET platform. ASP.NET Core is the open-source version of ASP.NET, that runs on macOS, Linux, and Windows. ASP.NET Core was first released in 2016 and is a re-design of earlier Windows-only versions of ASP.NET.
Using ASP.NET Core we can develop –
- Cloud Web Apps.
- Services.
- Internet of Things Apps.
- Mobile Backend.
Why ASP.NET Core?
Cross Platform: ASP.NET Core applications can run on Windows, Linux, and Mac. You don not need to develop different apps for different platforms using different frameworks.
Performance: ASP.NET Core no longer depends on System.Web.dll Requied for IIS for browser-server communication. ASP.NET Core allows us to include packages that we need for our application. This reduces the request pipeline and improves performance and scalability.
Open Source:- ASP.NET Core is open souce project and community project and lot of contributors.
Built in Dependency Injections: It includes the built-in IoC container for automatic dependency injection which makes it maintainable and testable.
Integration with Client Side Frameworks(SPA):It allows you to use and manage modern UI frameworks such as Angular, ReactJS, Knockout, Bootstrap, etc.
Hosting: ASP.NET Core web application supportsmultiple hosting platforms,It is not depend on only IIS. Currently it .Net Core ability to host on the following:
- Kestrel
- IIS
- HTTP.sys
- Nginx
- Apache
- Docker
Code Sharing:- It allows you to build a class library that can be used with other .NET frameworks such as .NET Framework 4.x or Mono. Thus a single code base can be shared across frameworks.
Side-by-Side App Versioning:– ASP.NET Core runs on .NET Core, which supports the simultaneous running of multiple versions of applications.
Smaller Deployments:– ASP.NET Core application runs on .NET Core, which is smaller than the full .NET Framework. Smaller deployment sizes will reduce the deployment overhead and improve the performance of the application.
A unified story for building web UI and web APIs:- ASP.NET Core has merged the MVC controller & Web API Controller , so you do not need to write the code twice one for webapi and another for mvc.
Architect ed for test-ability :- ASP.NET Core designed from scratch to support the Test Driven Development. It is built in DI and IOC which increase the test ability of ASP.NET Core Application.
Razor Pages:- Razor Pages is new path in ASP.NET core which makes coding page-focused scenarios easier and more productive just like web form.
Blazor:- A new Framework comes with ASP.NET Core lets you use C# in the browser alongside JavaScript. Share server-side and client-side app logic all written with .NET.
ASP.NET Core is Modular: ASP.NET Core is modular and it supports concept of middle ware those can be injected in the request pipe line, to configure the request pipe line.
ASP.NET Core Release Dates
Version | Original Release Date |
.NET Core 3.1 | December 3, 2019 |
.NET Core 3.0 | September 23, 2019 |
.NET Core 2.2 | December 4, 2018 |
.NET Core 2.1 | May 30, 2018 |
.NET Core 2.0 | August 14, 2017 |
.NET Core 1.1 | November 16, 2016 |
.NET Core 1.0 | June 27, 2016 |
Installation
- You can install .Net Core with visual studio 2019 installation process.
- Download https://visualstudio.microsoft.com/free-developer-offers/
- Select option (checkbox) .NET Core cross platform Development.

- You do not need to install the ASP.NET Core separately it is part of .NET Core bundle.
- By default visual studio 2019 will install .net core 2.0, you can check it by a command – doetnet –version from command prompt.
- To install .net core 3.x first download https://dotnet.microsoft.com/download
Create A Application using Visual Studio.
- Open Visual Studio.
- On the start window, choose Create a new project.
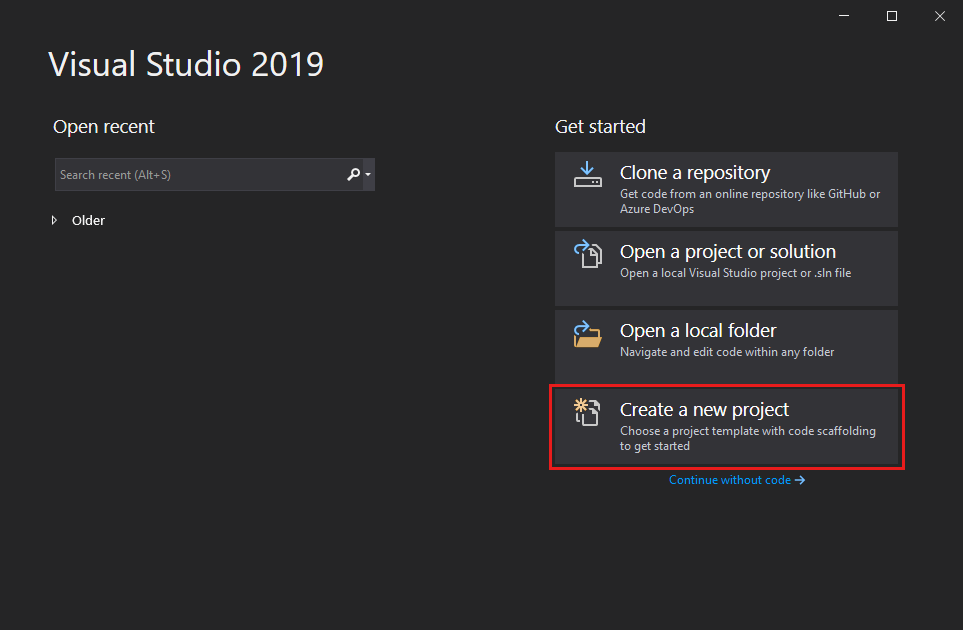
3. On the Create a new project window, enter or type ASP.NET in the search box. Next, choose C# from the Language list, and then choose Windows from the Platform list.
After you apply the language and platform filters, choose the ASP.NET Core Web Application template, and then choose Next.
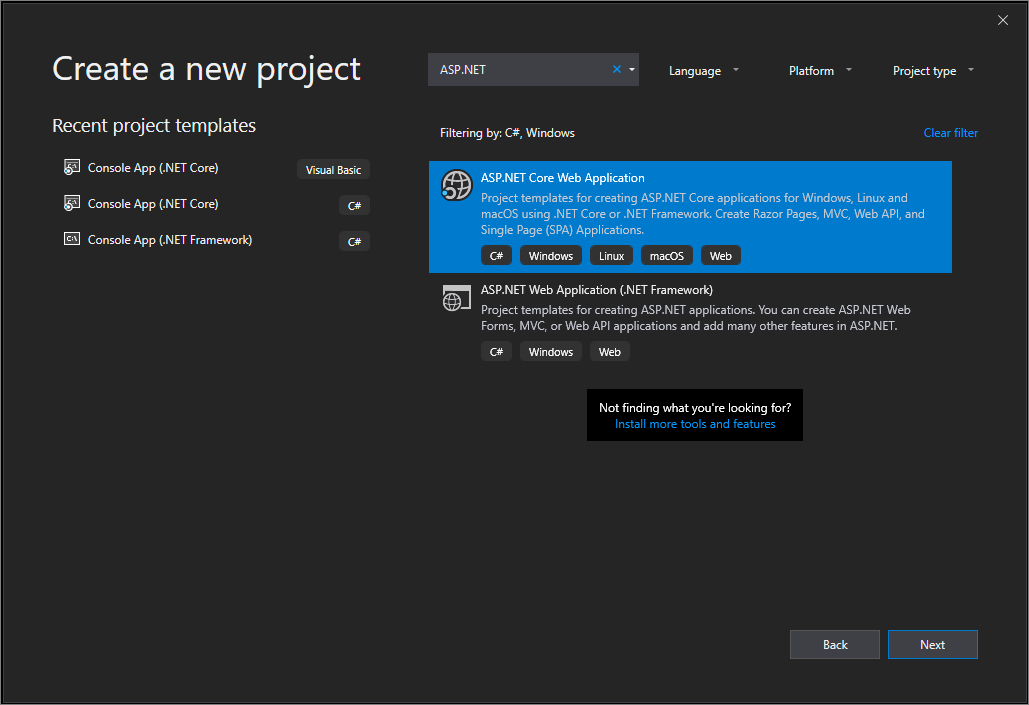
4. In the Configure your new project window, type or enter HelloWorld in the Project name box. Then, choose Create.

Following are the projects –
- Empty
- API
- Web Application (Razor Pages)
- Web Application (Model View Controller)
- Razor Class Library
- Angular
- React
- React & Redux
5. In the Create a new ASP.NET Core Web Application window, verify that ASP.NET Core 3.1 appears in the top drop-down menu. Then, choose Empty Web Application. Next, choose Create.
Project Files in ASP.NET core
- ASP.NET Core 1.0 does not create .csproj file, instead, it uses .xproj and project.json files to manage the project.
- This has changed in ASP.NET Core 2.0. Visual Studio now uses .csproj file to manage projects.
- We can edit the .csproj settings by right clicking on the project and selecting Edit
- The csproj file includes settings related to targeted .NET Frameworks, project folders, NuGet package references etc.
- We do not need to unload project for editing the project file. (as old version).
- File & Folder References not included in a file. (Files in Root Folder are the part of project)
References are Dependencies in ASP.NET Core
- .csproj file has package reference tag to define the package references.
- In new asp.net core packages are referenced as dependencies and categories as per the package manager.
- Asp.Net Core also have a concept of meta package it maintains list of packages those can be reference with single entry.
- You can install dependencies using nuget package manager
Properties section in ASP.NET Core
The Properties node includes launchSettings.json file which includes Visual Studio profiles of debug settings.
Launch settings contains the configuration settings for debug profile and development.
Which can contain multiple profiles and environment variables and as per the we can write conditional code –
E.g. IsDevelopment in Startups class.
wwwroot folder in ASP.NET Core
By default, the wwwroot folder in the ASP.NET Core project is treated as a web root folder.
Static files can be stored in any folder under the web root and accessed with a relative path to that root.
You can create separate folders for the different types of static files such as JavaScript, CSS, Images, library scripts etc.
Main Method in ASP.NET Core
Initially ASP.NET Core application stars as console application.
Main method is entry point for application.
Where we can configure ,host and run asp.net core application
CreateWebHostBuilder calls createDefaultBuilder method of WebHost it creates default web host with certain preconfigured default settings.
It Uses a Startup class, which contains methods to configure services and Configure.
Create Default Builder in ASP.NET Core
- Setting up the web server.
- Loading the host and application configuration from various configuration sources.
- Configuration logging.
- It Uses a Startup class, which contains methods to configure services and Configure
In / Out Process Hosting in ASP.NET Core
By default when you run the Application in visual studio It will use the in process hosting model that means it will use the IIS Express Worker Process.
When we run the application from the command prompt it runs in Out Of Process hosting it Kestrel
Kestrel Cross platform web server for asp.net core and it is default server for out process hosting model .