Introduction to Java Script

What is client side scripting/ programming?
client side scripting or programming enables you to write a small code blocks those allow to add interactivity or dynamicness to static html documents. e.g. user inputs, animations, effects, validation etc. Few common client side scripting languages are – vbscript, javascript etc
What is Java Script?
Java Script is worlds most popular, client side programming scripting technology.It is used to add some dynamic ness or interactivity with html document.
JavaScript is a dynamic Scripting / client side programming language. It is lightweight and most commonly used as a part of web pages, whose implementations allow client-side script to interact with the user and make dynamic pages.
JavaScript is a high level, interpreted, programming language used to make web pages more interactive.
What we can do with JavaScript?
- JavaScript is a high level, interpreted, programming language used to make web pages more interactive.
- JavaScript is the Programming Language for the Web.
- JavaScript can update and change both HTML and CSS.
- JavaScript can calculate, manipulate and validate data.
- JavaScript can be used to add animations/ effects.
- JavaScript can be used to manipulate the DOM.
What we can do with JavaScript?
- JavaScript is a high level, interpreted, programming language used to make web pages more interactive.
- JavaScript is the Programming Language for the Web.
- JavaScript can update and change both HTML and CSS.
- JavaScript can calculate, manipulate and validate data.
- JavaScript can be used to add animations/ effects.
- JavaScript can be used to manipulate the DOM.
Feature of JavaScript
Dynamic Typed Language: – java script do not have static types, JavaScript supports dynamic types, it means data type of variable will be decided at runtime not at compile time.
Java Script is Interpreted: – java script uses interpreter and translated and executed line by line by the web browser.
Java Script is Case Sensitive: – just like C JavaScript differentiate between the small case and capital case words.
Object Based: – JavaScript provides you built in objects around which you can write code. It also provides you facility using which you can create the object and use them. But it does not support full concepts of Object Oriented Programming Language.
It’s easy to learn and simple to implement :- JavaScript syntax is just like c, so any new programmer can easily learn and understand the JavaScript.
JavaScript is platform independent :- works on all browser and all platforms.
What is difference between HTML, CSS & Java Script?
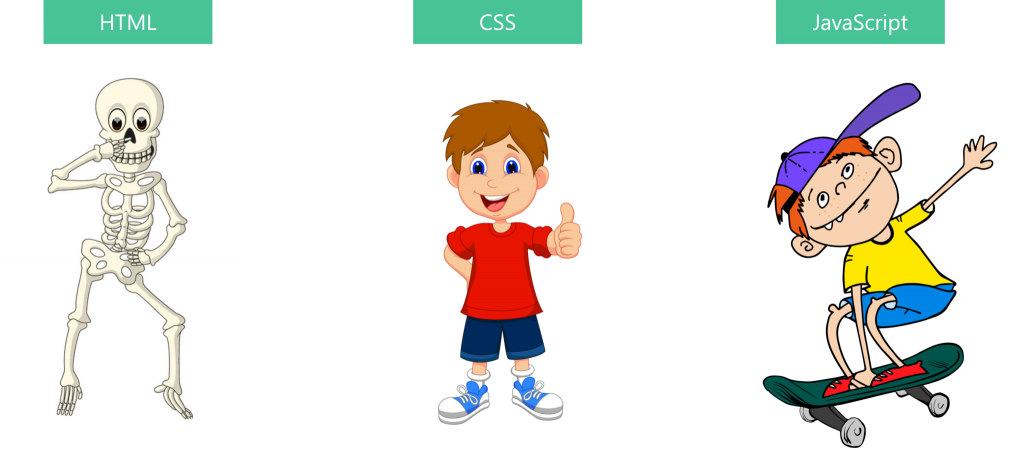
Think of HTML (HyperText Markup Language) as the skeleton of the web. It is used for displaying the web.
On the other hand, CSS is like our clothes. We put on fashionable clothes to look better. Similarly, the web is quite stylish as well. It uses CSS which stands for Cascading Style Sheets for styling purpose.
Then there is JavaScript which puts life into a web page. Just like how kids move around using the skateboard, the web also motions with the help of JavaScript.
How to use JavaScript?
Embed inside Page: – you can embed JavaScript in page using <script> tag as follows –
<script type="text/JavaScript">
code
</script>
What ever code you write in script blog will be executed as soon as you open the document in browser.
You can create separate js file which contains JavaScript code that can be referenced in html document using script tag –
<script type="text/JavaScript" src="path of JavaScript file"></script>
How to create variables in Java Script?
Since JavaScript is dynamic typed language,to create variable in JavaScript we use the var keyword and variable name with initial value as follows –
var variablename[=initial value];
e.g. var v=10;
var s="Rakesh"
Fundamentals of JavaScript
in JavaScript fundamentals we are going to study the following functions –
- Variables
- Conversion functions
- Dialog Boxes
- Conditions
How to create variables in Java Script?
Since JavaScript is dynamic typed language,to create variable in JavaScript we use the var keyword and variable name with initial value as follows –
var variablename[=initial value];
e.g. var v=10;
var s="Rakesh"
Data Type Conversion Functions in JavaScript
we are going to use following three functions for data type conversion –
parseInt(value) :- will convert the given value to integer.
parseFloat(value):-will convert the given value to float.
parseDate(value):- will convert the given value to date.
Java Script Dialog Boxes
JavaScript supports three important types of dialog boxes. These dialog boxes can be used to raise and alert, or to get confirmation on any input or to have a kind of input from the users.
alert :- alert box is used to display some message/information to the user with OK button.
prompt:-prompt box is used to accept a input from the user, it provides you a input box with OK and cancel button. When you click on OK it will accept the input, when you press cancel it will not accept the input.
confirm :- confirm box used to ask a question and get confirmation from the user, confirm box will have button OK and cancel. when user click on OK it returns true, when cancel it returns false.
e.g. Input two no from the user and display their addition.
<html>
<head>
<title>Addition of two numbers</title>
<script type="text/javascript">
var n1 =parseInt(prompt("Enter First No:"));
var n2=parseInt(prompt("Enter Second No:"));
var r =n1 + n2;
alert("Addition is:"+ r);
</script>
</head>
<body>
</body>
</html>
Conditional statements
Any expression or statement when evaluated will either generate true or false is called condition. To implement conditions in JavaScript , we can use two statements if and if else as follows –
If Statement
in if we can handle only true part of condition, if condition is true, then we execute some code, if condition is not true then it will not do any thing.
if(condition)
{
statements/ instructiosn;
}

If-else statement
If else statement is used to handle the condition, here if condition is true it will execute the true part of the condition , if condition is not true, it will execute the false part of the condition.
if(condition)
{
statemetns/instructions
}
else
{
statemetns/instructions
}

e.g. Input a integer number from the user and display weather it is even or odd number.
<html>
<head>
<title> even odd </title>
<script type="text/javascript">
var v =prompt("Enter No:");
if(v%2==0)
alert("Even Number!");
else
alert("Odd Number!");
</script>
</head>
<body>
</body>
</html>
Nested Conditions
Condition inside another condition is called nested condition. you can nest if inside if or if inside else or if else inside if as per your requirements.
if(condition)
{
statements/instruction;
if()
{
if()
{}
else
{}
}
}
else
{
if()
{}
else
{}
}
e.g. write a program in JavaScript to input three integer numbers from the user and display greater number from it.
<html>
<head>
<title> even odd </title>
<script type="text/JavaScript">
var a =parseInt(prompt("Input value for A:"));
var b=parseInt(prompt("Input value for B:"));
var c=parseInt(prompt("Input value for C:"));
if(a>b)
{
if(a>c)
{
alert("A is greater!");
}
else
{
alert("C is greater");
}
}
else
{
if(b>c)
{
alert("B is Greater");
}
else
{
alert("C is Greater");
}
}
</script>
</head>
<body>
</body>
</html>
Logical Operators
JavaScript provides you && (and), || (or) & ! (not) operators to combine the condition, in case && resulting condition will be true only when all the conditions joined using && are true otherwise it will be false, where as in || operator resulting condition will be true if any of the condition used to join if true, if all are false then it will be false, where as ! will revert the condition.
e.g. write a program in JavaScript to input a integer number and display weather it is two digit number or not a two digit number.
<html>
<head>
<title> even odd </title>
<script type="text/javascript">
var a =parseInt(prompt("Input value for A:"));
if(a> 9 && a<100)
alert("Two digit number!");
else
alert("Not Two digit number!");
</script>
</head>
<body>
</body>
</html>
Loops or looping statement
Loops or looping statements are used to repeat a specific block until some end condition is met. There are three categories of loops in JavaScript :
- for loop
- while loop
- do while loop
For loop in JavaScript
for loop is used when you know in advance how many times you want to execute the loop. The for loop repeatedly executes the loop code while a given condition is TRUE.
for(begin; condition; step) {
loop code;
}
In the above syntax:
begin statement is executed one time before the execution of the loop code
condition defines the condition for executing the loop code
step statement is executed every time after the code block has been executed.
break: – used to break or terminate the loop in between.
continue:- used to continue the execution of loop by skipping some part of loop.
e.g. input 10 integer numbers from the user and display count of even and odd number.
<html>
<head>
<title> even odd </title>
<script type="text/javascript">
var ecnt=0,ocnt=0;
for(var i=0;i<10;i++)
{
var n=parseInt(prompt("Enter No:"));
if(n % 2 ==0)
ecnt++;
else
ocnt++;
}
alert("Even Count is:"+ ecnt);
alert("Odd Count is:"+ ocnt);
</script>
</head>
<body>
</body>
</html>
While Loop in Java Script
used when you do not in advance how many times you want to execute a loop, in while loop will be executed till condition is true, their is no beg and end conditions here.
while(condition) {
loop code;
}
e.g. input a integer number from the user and display adidition of all digits of that number.
<html>
<head>
<title> even odd </title>
<script type="text/javascript">
var sum=0,dig,num;
num =parseInt(prompt("Enter No:"));
while(num!=0)
{
dig =num % 10;
sum = sum +dig;
num=parseInt(num/10); //floating point division
}
alert("Sum is:"+ sum);
</script>
</head>
<body>
</body>
</html>
do-while loop in JavaScript.
do while is called post check, it will execute the loop at least for one time and further execution depends on condition. This loop will first execute the code, then check the condition and while the condition holds true, execute repeatedly.
do
{
statements/ instructions;
}while(condition);
e.g. input integer numbers from the user and display addition of all inputted numbers until user do not enter number divisible by 7.
<html>
<head>
<title> even odd </title>
<script type="text/javascript">
var sum=0;
do
{
var num = parseInt(prompt("Enter no:"));
sum =sum + num;
}while(num % 7!=0);
alert("Sum is:"+ sum);
</script>
</head>
<body>
</body>
</html>
Nested loops in Java Script
Loop inside another loop is called nested loop. You can nest for inside another for, while inside another while, for inside while as per your requirements.
e.g. display all armstrong numbers from 10 to 500, armstrong number is number whoes cube of digits added togeather will generate a same number is called armstrong number.
153 = cube(1) + cube(5) + cube(3)
= 1 + 125 + 27
=153
<html>
<head>
<title> even odd </title>
<script type="text/javascript">
var num,dig,sum;
for(var i=10;i<=500;i++)
{
num=i;
sum =0;
while(num!=0)
{
dig = num % 10;
sum += dig *dig *dig;
num=parseInt(num/10);
}
if(sum ==i)
alert(i);
}
</script>
</head>
<body>
</body>
</html>