How to Implement Validation in ASP.NET MVC? (Part 2)
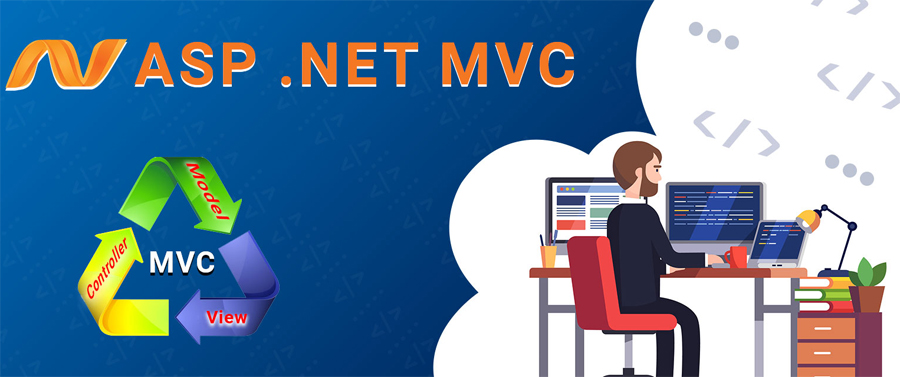
Example Source Code: https://drive.google.com/file/d/18IPwYq1aujZ3waQHR0QTeeJvPp7By9H2/view?usp=sharing
Other Attributes
Data Type: this attribute will help you to define the data type of any model property which will be followed by helper while generating HTML in a view or ui, few data types are MultiLineText, Password, Credit Card, Url etc.
[Required(ErrorMessage ="Password Required")]
[Compare("ConfirmPassword",ErrorMessage ="Password and confirm password are not same!")]
[DataType(DataType.Password)]
public string Password { get; set; }
[Required(ErrorMessage ="Address Required")]
[StringLength(10,MinimumLength =5,ErrorMessage ="Invalid Address Length")]
[DataType(DataType.MultilineText)]
public string Address { get; set; }
Display: will help you to change the name of the column or field for diplay name for or label for helper, can be used as follows –
[Required(ErrorMessage ="Customer Name Required")]
[Display(Name ="Enter Customer Name")]
public string CustomerName { get; set; }
How to implement Custom Validation?
To implement a custom validation we have two options –
- Write a custom code in action to check input and as per that you can add a custom model error in model state object using AddModelError method of modelstate object.
public ActionResult Create(Customer rec)
{
if (ModelState.IsValid) {
if (rec.CreditLimit % 2 != 0)
{
ModelState.AddModelError("CreditLimit", "Please Input Even Credit Limit");
ModelState.AddModelError("", "Please Input Even Credit Limit!");
return View(rec);
}
return RedirectToAction("Index");
}
return View(rec);
}
2. Create a custom validation attribute.
How to create custom validation attribute in ASP.NET MVC?
Create custom validation attribute define a class which inherit from built in class called ValidationAttribute.
Override IsValid Method and write a logic to validate the input and returns and true if their is no validation problem otherwise return false.
then apply the newly created attribute to the model property as per requirements.
You can do it as follows –
public class EvenVal:ValidationAttribute
{
public override bool IsValid(object value) // will get you value of property
{
double val = Convert.ToDouble(value.ToString());
if (val % 2 == 0)
{
return true;
}
else
{
return false;
}
}
}
Validating Model class generated using Entity Framework Database First.
If you remember in entity db first the model classes will be generated automatically based on table select while creating edmx file, so it is not recommended to apply validation into those generated classes, since those classes many need to be generated again if their is changes in database, but the classes generated by entity framework are partial so we can provide it’s additional definition to another class.
Step 1: create a class similar to model class with those properties where you want to apply the validation and apply data annotation attributes on those model properties, as follows –
public class CustomerVal
{
[Required(ErrorMessage ="Customer Name Required")]
public string CustomerName { get; set; }
[Required(ErrorMessage ="Address Required")]
[StringLength(10,MinimumLength =5,ErrorMessage ="Invalid String Length!")]
public string Address { get; set; }
[Required(ErrorMessage ="Email Address Required")]
[EmailAddress(ErrorMessage ="Invalid Email ID")]
public string EmailID { get; set; }
[Required(ErrorMessage ="Mobile No Required")]
[RegularExpression(@"^\d{10}$",ErrorMessage ="10 Digits Required")]
public string MobileNo { get; set; }
[Required(ErrorMessage ="Credit Limit Required")]
[Range(1000,10000,ErrorMessage ="Invalid Credit Limit")]
public Nullable<decimal> CreditLimit { get; set; }
}
Step 2: Define a partial class and apply metadatatype attribute on it to tell above class contains the meta data about the actual model class.
[MetadataType(typeof(CustomerVal))]
public partial class CustomerTbl
{
}