Forms in HTML?
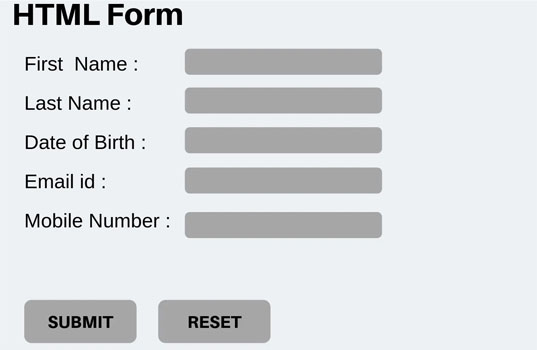
In this session we will try to understand how to create html forms and how to use it.
What is Form?
Form is tool by which we collect information form the user. You might filled lot of forms like admission form, enquiry form etc.
What is HTML Form?
An HTML form is used to collect user input. The user input can then be sent to a server for processing. To create the form in html we use <form></form> tag as follows –
<form name="nameofform" action="pathofserverprogram" method="post">
form elements
</form>
Action Attribute : allow you to specify the path of back end script /server program ready to process your passed data.
Method attribute: Method to be used to upload data. The most frequently used are GET and POST methods. When you use get the data send will be visible on address bar where as if you submit data using post it will not be visible on address bar.
HTML Form Controls /Elements
Form controls/ elements are the tags provided by html which will help you to accept individual value or data from the user. There are different types of form controls that you can use to collect data using HTML form, they are as follows –
- Text Input Controls
- Checkboxes Controls
- Radio Box Controls
- Select Box Controls
- File Select boxes
- Hidden Controls
- Clickable Buttons
- Submit and Reset Button
The Element
<label> tag is used element is used to display label or title for some form filed
<form>
<label> this is label </label>
</form>
Input Controls/ HTML Input Controls
<input> tag is used to accept the input from the user, it has following syntax –
<input type="type" size="" value="" name="" id="" />
Type attribute:- used to specify what type of input control you need, where type can be – text, password, radio, checkbox, button, reset ,submit
Size Attribute: -The input size attribute specifies the visible width, in characters, of an input field.The default value for size is 20.
Value Attribute: – allow you to specify the value that you want to be in that control.
Name Attribute: – allow you to specify the name for the input that can be used to submit the data to server.
Id Attribute: – allow you to specify the id ,used to identify the control and can be accessed using client side scripts.
Input Type Text
<input type=”text” /> defines a single-line text input field,used to accept a single line input from the user.
<form>
<label for="fname">First name:</label><br>
<input type="text" id="fname" name="fname"><br>
<label for="lname">Last name:</label><br>
<input type="text" id="lname" name="lname">
</form>
Input Type Password
<input type=”password” /> defines a password field, used to accept a password, pin or any code.
<form>
<label for="username">Username:</label><br>
<input type="text" id="username" name="username"><br>
<label for="pwd">Password:</label><br>
<input type="password" id="pwd" name="pwd">
</form>
Input Type Submit
<input type=”submit” /> defines a button for submitting form data to a form-handler. When you click on the submit button it will send the form data to the server script or program specified in action attribute.
<form action="/action_page.php">
<label for="fname">First name:</label><br>
<input type="text" id="fname" name="fname" value="John"><br>
<label for="lname">Last name:</label><br>
<input type="text" id="lname" name="lname" value="Doe"><br><br>
<input type="submit" value="Submit">
</form>
Input Type Reset
<input type=”reset” /> defines a reset button that will reset all form values to their default values when clicked.
<form action="/action_page.php">
<label for="fname">First name:</label><br>
<input type="text" id="fname" name="fname" value="John"><br>
<label for="lname">Last name:</label><br>
<input type="text" id="lname" name="lname" value="Doe"><br><br>
<input type="submit" value="Submit">
<input type="reset">
</form>
Input Type Radio
<input type=”radio” /> defines a radio button. Radio buttons let a user select ONLY ONE of a limited number of choices:
<form>
<input type="radio" id="male" name="gender" value="male">
<label for="male">Male</label><br>
<input type="radio" id="female" name="gender" value="female">
<label for="female">Female</label><br>
<input type="radio" id="other" name="gender" value="other">
<label for="other">Other</label>
</form>
Input Type Checkbox
<input type=”checkbox” />
defines a checkbox.
Checkboxes let a user select ZERO or MORE options of a limited number of choices.
<form>
<label for="Technology"> Client Side Tech </label><br>
<input type="checkbox" value="Angular" /> Angular
<input type="checkbox" value="Knockout" /> Knockout
<input type="checkbox" value="ReactJS" /> React JS
<input type="checkbox" value="Vue" /> Vue
</form>
Input Type Button
<input type=”button” /> will create a button, where from you can fire click event, you can click on button to execute some code.
<form>
<input type="button" onclick="alert('Hello World!')" value="Click Me!">
</form>
Input Type File
The <input type=”file” /> defines a file-select field and a “Browse” button for file uploads.
<form>
<label for="myfile">Select a file:</label>
<input type="file" id="myfile" name="myfile">
</form>
Input Type Hidden.
<input type=”hidden” /> is used to store some data in form that will not be visible to the user.
<form>
<input type="hidden" name="color" value="red">
</form>
Select Tag /Element in Form
<select> tag is used to create the select element/ tag. Select tag is used to provide the options to the user where from user can select only one option by default. To create the options in a select tag we use the option tag.
<select name=”selectname”> </select>
Options tag is used to provide the options to the user –
<option value=”‘ selected> text </option>
Value property: – value is to specify the value to the server when user select that option. If value is not specified, then it will submit the text. Selected Property:- it is boolean property, when true it will automatically select that option when false it will not select that element.
<select name="cars" id="cars">
<option value="volvo">Volvo</option>
<option value="saab">Saab</option>
<option value="mercedes">Mercedes</option>
<option value="audi">Audi</option>
</select>
Multiple Attribute: – this attribute is used if you want to select the multiple options. When multiple is applied on select it will convert the dropdown box to listbox.
<select name="cars" id="cars">
<option value="volvo">Volvo</option>
<option value="saab">Saab</option>
<option value="mercedes">Mercedes</option>
<option value="audi">Audi</option>
</select>
Text Area Tag
The HTML <textarea></textarea> tag element represents a multi-line plain-text editing control, useful when you want to allow users to enter a sizable amount of free-form text.
<form>
<textarea id="story" name="story" rows="5" cols="33">
It was a dark and stormy night...
</textarea>
</form>
HTML Button
The HTML <button> element represents a clickable button, used to submit forms or anywhere in a document for accessible, standard button functionality.
<form>
<button class="favorite styled"
type="button">
Add to favorites
</button>
</form>