ASP.NET MVC Scaffold Templates
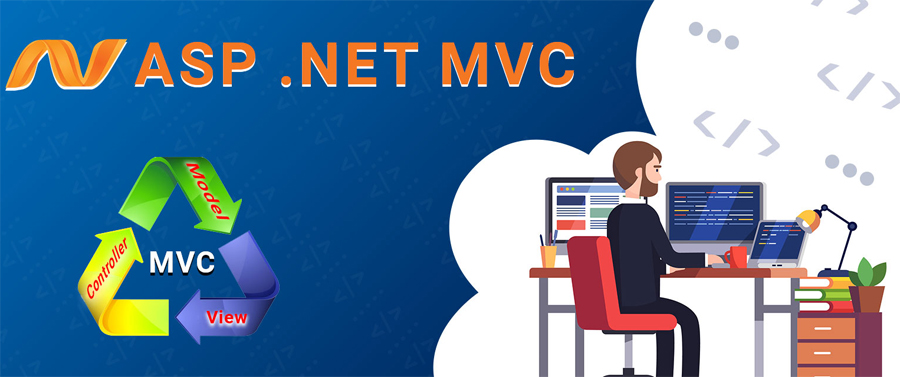
Example Code : https://drive.google.com/file/d/1XQTimW39SuNI7kxrhvPmUQ_6MQlp34YC/view?usp=sharing
Scaffolding is a technique used by many MVC frameworks like ASP.NET MVC to generate code for basic CRUD (create, read, update, and delete) operations against your model classes /database. For a view ASP.NET MVC provides you following templates –
- Empty
- Empty with Model
- List
- Create
- Edit
- Delete
- Details
Empty template:- will create the empty view, for all previous demo we had used it.
Empty with Model: – this template allow you to select the model class and generate the empty strongly typed view which is connected to selected model.
List: – when you select list template it will force you to select the model class and it will create a strongly typed view which is connected to IEnumerable collection of that model and will generate code to display data in table format /tabular format using foreach and table, tr , th tags.
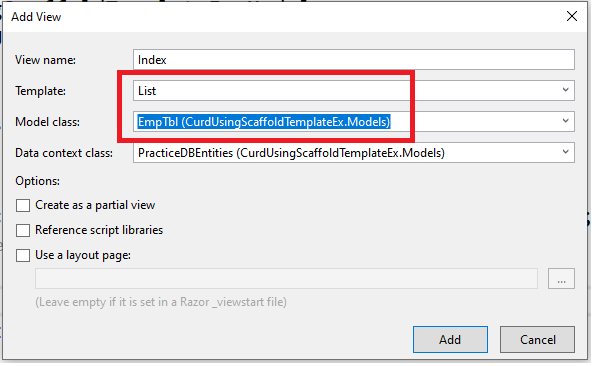
Generated View Code: –
@model IEnumerable<CurdUsingScaffoldTemplateEx.Models.EmpTbl>
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<p>
@Html.ActionLink("Create New", "Create")
</p>
<table class="table">
<tr>
<th>
@Html.DisplayNameFor(model => model.EmpName)
</th>
<th>
@Html.DisplayNameFor(model => model.MobileNo)
</th>
<th>
@Html.DisplayNameFor(model => model.Salary)
</th>
<th>
@Html.DisplayNameFor(model => model.DeptTbl.DeptName)
</th>
<th></th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.EmpName)
</td>
<td>
@Html.DisplayFor(modelItem => item.MobileNo)
</td>
<td>
@Html.DisplayFor(modelItem => item.Salary)
</td>
<td>
@Html.DisplayFor(modelItem => item.DeptTbl.DeptName)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id=item.EmpID }) |
@Html.ActionLink("Details", "Details", new { id=item.EmpID }) |
@Html.ActionLink("Delete", "Delete", new { id=item.EmpID })
</td>
</tr>
}
</table>
</body>
</html>
Create Template : – when you select create tamplete it will create the UI code to create the model object. It will create a stronlgy typed view which is conected with single instnace of the model class. It contains the code which will generate the input form for that model class. Using that form you can input the data and create the object of that class when you submit that form. We can do it while adding the view.
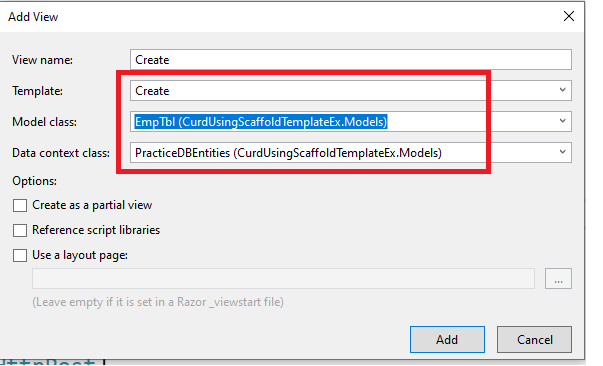
View Code Generated:
@model CurdUsingScaffoldTemplateEx.Models.EmpTbl
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Create</title>
</head>
<body>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<h4>EmpTbl</h4>
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
<div class="form-group">
@Html.LabelFor(model => model.EmpName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.EmpName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.EmpName, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.MobileNo, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.MobileNo, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.MobileNo, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.DeptID, "DeptID", htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.DropDownList("DeptID", null, htmlAttributes: new { @class = "form-control" })
@Html.ValidationMessageFor(model => model.DeptID, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Salary, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Salary, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Salary, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Create" class="btn btn-default" />
</div>
</div>
</div>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
</body>
</html>
Edit Template: –
When you select edit template it will force you to select the model class and it will generate the form for that model class( it will generate form code automatically) using which you can edit the object of the model class when you submit that form.
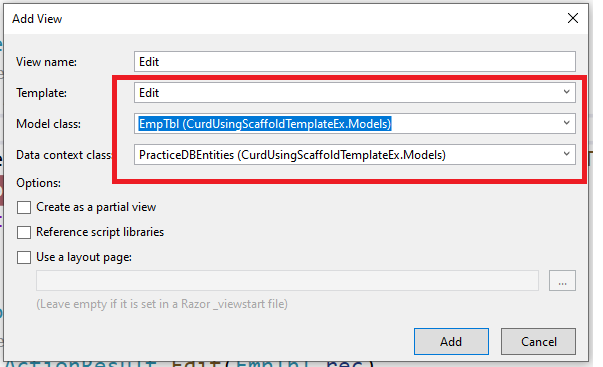
Edit Template View Code:-
@model CurdUsingScaffoldTemplateEx.Models.EmpTbl
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Edit</title>
</head>
<body>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<h4>EmpTbl</h4>
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
@Html.HiddenFor(model => model.EmpID)
<div class="form-group">
@Html.LabelFor(model => model.EmpName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.EmpName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.EmpName, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.MobileNo, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.MobileNo, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.MobileNo, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.DeptID, "DeptID", htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.DropDownList("DeptID", null, htmlAttributes: new { @class = "form-control" })
@Html.ValidationMessageFor(model => model.DeptID, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Salary, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Salary, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Salary, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Save" class="btn btn-default" />
</div>
</div>
</div>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
</body>
</html>
Details Template:
Details template is used to display record in details. It also forces you to select the model and it will generate the details view for the selected model. It contains UI code to display the record using dd,dl and dt tags.
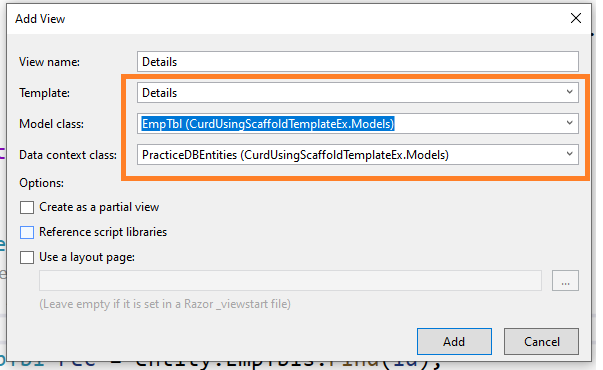
Details Template View Code:-
@model CurdUsingScaffoldTemplateEx.Models.EmpTbl
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Details</title>
</head>
<body>
<div>
<h4>EmpTbl</h4>
<hr />
<dl class="dl-horizontal">
<dt>
@Html.DisplayNameFor(model => model.EmpName)
</dt>
<dd>
@Html.DisplayFor(model => model.EmpName)
</dd>
<dt>
@Html.DisplayNameFor(model => model.MobileNo)
</dt>
<dd>
@Html.DisplayFor(model => model.MobileNo)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Salary)
</dt>
<dd>
@Html.DisplayFor(model => model.Salary)
</dd>
<dt>
@Html.DisplayNameFor(model => model.DeptTbl.DeptName)
</dt>
<dd>
@Html.DisplayFor(model => model.DeptTbl.DeptName)
</dd>
</dl>
</div>
<p>
@Html.ActionLink("Edit", "Edit", new { id = Model.EmpID }) |
@Html.ActionLink("Back to List", "Index")
</p>
</body>
</html>
Delete Template: – when you select this template it will force you to select the model class when you select the model class it will create a strongly typed view connected with single instance of the model class. It will auto generate the code to display the record with delete button and when you click on the delete button it will delete that record.
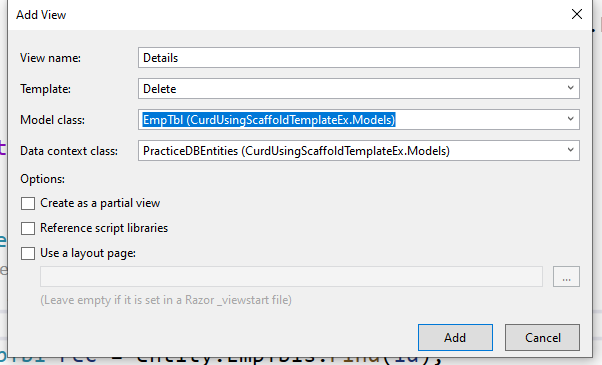
View Code:
@model CurdUsingScaffoldTemplateEx.Models.EmpTbl
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Delete</title>
</head>
<body>
<h3>Are you sure you want to delete this?</h3>
<div>
<h4>EmpTbl</h4>
<hr />
<dl class="dl-horizontal">
<dt>
@Html.DisplayNameFor(model => model.EmpName)
</dt>
<dd>
@Html.DisplayFor(model => model.EmpName)
</dd>
<dt>
@Html.DisplayNameFor(model => model.MobileNo)
</dt>
<dd>
@Html.DisplayFor(model => model.MobileNo)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Salary)
</dt>
<dd>
@Html.DisplayFor(model => model.Salary)
</dd>
<dt>
@Html.DisplayNameFor(model => model.DeptTbl.DeptName)
</dt>
<dd>
@Html.DisplayFor(model => model.DeptTbl.DeptName)
</dd>
</dl>
@using (Html.BeginForm()) {
@Html.AntiForgeryToken()
@Html.HiddenFor(p=>p.EmpID)
<div class="form-actions no-color">
<input type="submit" value="Delete" class="btn btn-default" /> |
@Html.ActionLink("Back to List", "Index")
</div>
}
</div>
</body>
</html>