Areas in ASP.NET MVC 5
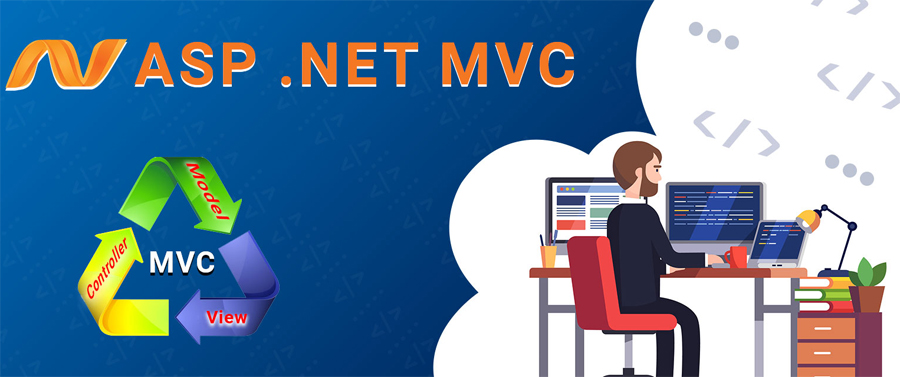
Area Example :https://drive.google.com/file/d/1eZZdAbMT_x2HOVMMpU2yvvfv-V_5EahD/view?usp=sharing
What is Area in ASP.NET MVC?
Area allows us to partition/divide the large application into smaller units where each unit contains a separate MVC folder structure, same as the default MVC folder structure. You can divide the aplication into areas as per use case, or sub module or module or functional unit depending on your requirements.
The large ASP.NET MVC application includes many controllers, views, and model classes. So it can be difficult to maintain it with the default ASP.NET MVC project structure.
Here we can use ASP.NET MVC feature called Area so that we can divide large application into different different areas. For example, a large enterprise application may have different modules like admin, billing, support, marketing, etc. So an Area can contain a separate MVC folder structure for all these modules, as shown below.

As you can see, each area includes the AreaRegistration class. The following is adminAreaRegistration class created with admin area.
public class adminAreaRegistration : AreaRegistration
{
public override string AreaName
{
get
{
return "admin";
}
}
public override void RegisterArea(AreaRegistrationContext context)
{
context.MapRoute(
"admin_default",
"admin/{controller}/{action}/{id}",
new { action = "Index", id = UrlParameter.Optional }
);
}
}
The AreaRegistration class overrides the RegisterArea method to map the routes for the area. In the above example, any URL that starts with the admin will be handled by the controllers included in the admin folder structure under the Area folder. For example, http://localhost/admin/profile will be handled by the profile controller included in the Areas/admin/controller/AdminHomeController folder.
Layouts in Area: –
Every area can have it’s own layout and _Viewstart file so that the views from that area can use that layout.
If any view uses layout from area and if area do not have layout it will automatically copy the default area in shared folder of that area.
Action Links in Areas:
When you use the action links in area you should be care full about the area name, because in previous paragraph we learnt that if you want to invoke any action from the area using action link then we need to use area name as first string in request url so when you use the action link to divert to the controllers from area you need to pass the area name in route values parameter in action link.
Route Values parameter in Action Link: –
Actin link helper has route values parameter and it will help you to add some parameter in request url syntax for the same is as follows –
@Html.ActionLink("text","actioname",routervaluesparameter)
@Html.ActionLink("text","actionname","controllername",routevaluesparameter,htmlattribute)
When you define a parameters in route values parameter it will check weather it is built in parameter, if it is a built in parameter then it will append it’s value at appropriate location in request url , if it is not built in parameter it will append it as query string parameter in request url.
e.g.
@Html.ActionLink("go to index","Index","Home", new {id=10,a="Ganesh"})
This will create a the url http://localhost:89898/home/index/10/?a=Ganesh here id is built in parameter so it get append at it’s position and but a is not built in parameter so it get append as query string parameter.
Similar way we can pass the area name as a route parameter in action link.
@Html.ActionLink("Go to Admin Home","Index","AdminHome",new {id="Sunil",area="Admin",val=10},null}
It will generate the following url –
http://localhost:89898/admin/adminhome/Index/Sunil/?val=10
Since area and id is built in variables so those get appended at their appropriate location and val will be get appended as the query string parameter.