Action Filters In ASP.NET MVC 5? Few Built in Filters.
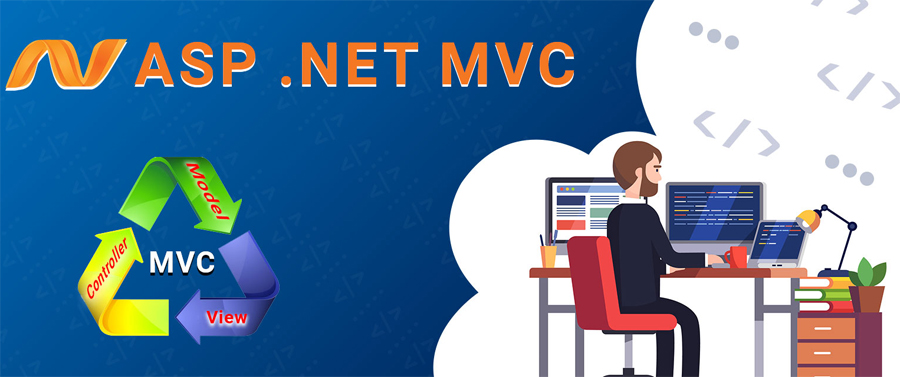
Example Code: https://drive.google.com/file/d/15OGfgD_p6pqZDOV8obhF4ykSYEAwhYTj/view?usp=sharing
Filters/ Action Filters are the built in or user defined attributes those can be applied on action or controller which help you to execute some logic/code before execution of action or after execution of the action. Filters allow you to add pre and post logic to action which will be executed before execution of action and after execution of action.
ASP.NET MVC Filters are classified or divided into following types of categories –
Authorization Filters:
Prforms authentication and authorizes before executing an action method. Used to validate the user credentials, tokens to check weather user is valid user or logged in user.
e.g. [Authorize], [RequireHttps], [AllowAnanymous]
Action filters:
Are built in or user define attributes those can be applied on action or controller performs some operation/execute some code before and after an action method executes.
e.g. [ValidateAntiForgeryToken]
Result filters/Response Filters:
Result filters are used to modify the result of controller action after action execution. It is very usefull if you want to return the modified result or view content of action.
e.g. [OutputCache]
Exception / Error Filters:
Exception filters help you to execute some code when error and exception occurred in any action from the controller of ASP.NET MVC 5.
e.g. [HandleError]
Let us try to use the built in Attributes –
[OutputCache] Response/Result Filter
This is built in filter in ASP.NET MVC 5 this filter when applied on action or controller it will cache the output of the action or all the actions from the controller for specified duration.
[OutputCache(Duration =10)]
When you apply the output cache on the controller action it will cache the output for the duration of 10 seconds. During this if any user makes the request it will not get new output it will get only the old output after 10 seconds if user makes the request will get a new output.
Create a new ASP.NET MVC application with TestController and GetDate method as follows –
namespace BuiltInFiltersEX.Controllers
{
public class TestController : Controller
{
// GET: Test
public ActionResult Index()
{
return View();
}
[OutputCache(Duration =10)]
public string GetDate()
{
return DateTime.Now.ToString();
}
}
When you run the TestController and GetDate action method it will display the cached result for 10 seconds and new result will be displayed after 10 seconds.
[HandleError] Exception Filter
This is Exception Filter will be used when you want to display the custom error view instead of built in error view in ASP.NET MVC 5 application. This attribute will work only when you enable the CustomError handling in ASP.NET MVC application.
Let us try to understand we will do it without Handle Error attribute –
Create ASP.NET MVC Application using regular steps.
Add Test controller and doDivision Action as follows and run it.
namespace BuiltInFiltersEX.Controllers
{
public class TestController : Controller
{
// GET: Test
public ActionResult Index()
{
return View();
}
public int doDivision(int a, int b)
{
return a / b;
}
}
}
when you call it http://localhost:56917/test/dodivision/?a=200&b=20 it will generate output 10
but if you make the request http://localhost:56917/test/dodivision/?a=200&b=0 will throw you exception divide by zero and will show you the following error screen
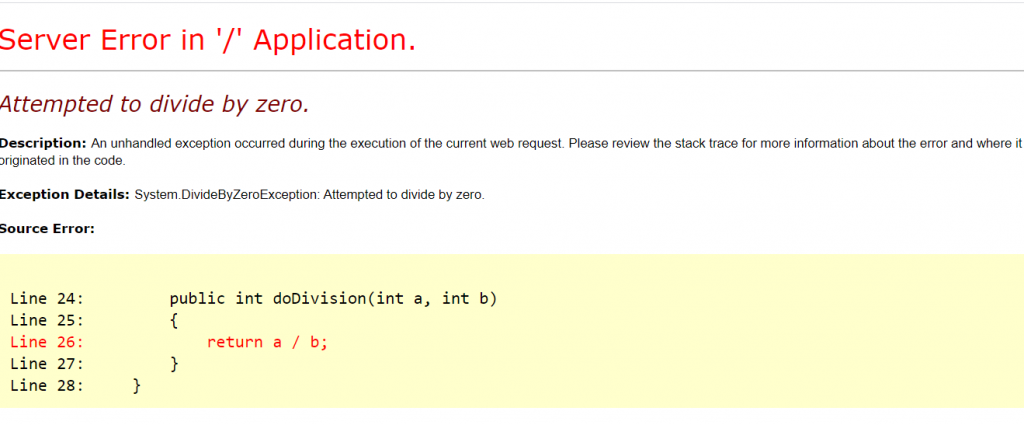
Now to display a custom error view first enable the custom error using web.config using CustomErrors tag as follows –
<customErrors mode="On/Off/RemoteOnly"></customErrors>
Where mode can be On, Off, RemoteOnly –
On – display custom error page
Off – you want to display built in error page (default)
RemoteOnly – you want to display built in error page in development and custom error page in remote machine.
You can go to web.config file of the project and go to the tag System.Web as follows –
<system.web>
<compilation debug="true" targetFramework="4.7.2" />
<httpRuntime targetFramework="4.7.2" />
<customErrors mode="On"></customErrors>
</system.web>
After this we can create the MyError.cshtml view as follows –
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Error</title>
</head>
<body>
<hgroup>
<h1>Error.</h1>
<h2>An error occurred while processing your request.</h2>
</hgroup>
</body>
</html>
Now apply [HandleError] attribute on doDivision action as follows –
namespace BuiltInFiltersEX.Controllers
{
public class TestController : Controller
{
[HandleError(View ="MyError")]
public int doDivision(int a, int b)
{
return a / b;
}
}
}
when you call it http://localhost:56917/test/dodivision/?a=200&b=20 it will generate output 10
but if you make the request http://localhost:56917/test/dodivision/?a=200&b=0 will throw you exception divide by zero and will show you the following error screen
Now it shows the following –
