How to Implement Validation in ASP.NET MVC? (Part1)
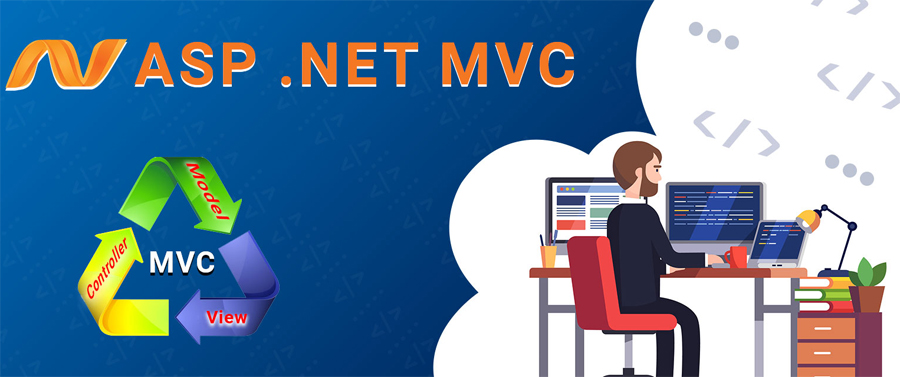
Example Link : https://drive.google.com/file/d/1XRi7oC0jtIPRIFde7gw8XTTxX2iIP4yj/view?usp=sharing
To implement a model validation in ASP.NET Core we need to use Data Annotation API.
What is Data Annotation API in ASP.NET Core ?
Data Annotation API is general purpose API provides you built in attributes those can be used for –
- Validation
- Data Types
- Display Rules
- Meta Data About Model
Data Annotation Attributes for Validation
Data Annotations Provides you following attributes those can be used for validation –
[Required] :- when you apply required attribute on model property, the input for that model property will be compulsory. If do not input the value it will show the error
[StringLength] :- can be used for strings, it allow you to specify the maximum and minimum string length to input i.e. minimum and maximum no of characters that you want allow user to input.
[Range]: – is similar to stringlength but used for numeric value, in range we can specify the minimum and maximum numeric value that is suppose to be inputted.
[EmailAddress] :- when applied allow user to input a valid email id , if user input the invalid email id it will show you error.
[RegularExpression] :- will help you to compare the value of model property /input to the predefined string patterns like email id, url, phone no, mobile no etc. To create the pattern called regular expression we can use various characters and commands few of them are listed as follows – ^ :- specify the start of the regular expression
$ :- specify the end of regular expression.
\d :- represents a digit.
\w :- represents a alphanumeric character.
{} :- specify the repetition and repetition with range.
[] :- single value from range of values with start and end character
Few Examples are –
^\d{4}$ :- to input 4 digits only
^\d{4,10}$:- to input 4,5,6,7,8,9,10 no of digits
^\w{5}$ :- to input five alpha
^\d{3}\w{3}$:- to input three digit and three alphanumeric characters.
^\d{5}[a-f]$:- five digits and one alphanumeric character between a to f.
[Compare] :- This validation attribute will help you to compare value of one model property to another.
All above validation attribute has a common property called ErrorMessage, this is named property allow you to specify the error message that you want to display to the uesr.
Beside these attributes you can also create a Custom Validation Attribute.
To implement validation we need to follow the following steps –
Step 1: Apply the any of the above validation attribute on model properties are per requirement.
Step 2: You can implement a server side validation using a ModelState Object.
Step 3: You can do the client side validation using jQuery Validate plugin of jQuery
Step 4: Make arrangement in a view to display the validation error messages in the view.
Let us implement a step by step in asp.net core project as follow –
Step 1: Create a customer model in Models folder and apply the data validation attributes as follows-
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Threading.Tasks;
namespace ModelValidationusingDataAnnotation.Models
{
public class Customer
{
public Int64 CustomerID { get; set; }
[Required(ErrorMessage ="Customer Name Required")]
public string CutomerName { get; set; }
[Required(ErrorMessage ="Address Required")]
[StringLength(10,MinimumLength =5,ErrorMessage ="Invalid Address Length!")]
public string Address { get; set; }
[Required(ErrorMessage ="Email ID Rquired")]
[EmailAddress(ErrorMessage ="Invalid Email ID")]
public string EmailID { get; set; }
[Required(ErrorMessage ="MobileNo Required")]
[RegularExpression(@"^\d{10}$",ErrorMessage ="10 digits required!")]
public string MobileNo { get; set; }
[Required(ErrorMessage ="Credit Limit REquired")]
[Range(1000,10000,ErrorMessage ="Invalid Credit Limit")]
public decimal CreditLimit { get; set; }
[Required(ErrorMessage ="Password Required")]
public string Password { get; set; }
[Required(ErrorMessage ="Password Required")]
[Compare("Password",ErrorMessage ="Password not confirm password are not same!")]
public string ConfirmPassword { get; set; }
}
}
Step4: Create a input form for the Customer with a helper ValidationMessageFor helper as follows –
[HttpGet]
public ActionResult Create()
{
return View();
}
@model ModelValidationusingDataAnnotation.Models.Customer
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Create</title>
</head>
<body>
<h2> New Customer </h2>
@using (Html.BeginForm())
{
<div>
@Html.LabelFor(p => p.CutomerName)
<div>
@Html.EditorFor(p => p.CutomerName)
@Html.ValidationMessageFor(p=>p.CutomerName)
</div>
</div>
<div>
@Html.LabelFor(p => p.Address)
<div>
@Html.EditorFor(p => p.Address)
@Html.ValidationMessageFor(p => p.Address)
</div>
</div>
<div>
@Html.LabelFor(p => p.EmailID)
<div>
@Html.EditorFor(p => p.EmailID)
@Html.ValidationMessageFor(p => p.EmailID)
</div>
</div>
<div>
@Html.LabelFor(p => p.MobileNo)
<div>
@Html.EditorFor(p => p.MobileNo)
@Html.ValidationMessageFor(p => p.MobileNo)
</div>
</div>
<div>
@Html.LabelFor(p => p.CreditLimit)
<div>
@Html.EditorFor(p => p.CreditLimit)
@Html.ValidationMessageFor(p => p.CreditLimit)
</div>
</div>
<div>
@Html.LabelFor(p => p.Password)
<div>
@Html.EditorFor(p => p.Password)
@Html.ValidationMessageFor(p => p.Password)
</div>
</div>
<div>
@Html.LabelFor(p => p.ConfirmPassword)
<div>
@Html.EditorFor(p => p.ConfirmPassword)
@Html.ValidationMessageFor(p => p.ConfirmPassword)
</div>
</div>
<div>
<input type="submit" value="Save" />
</div>
}
</body>
</html>
What is ValidationMessageFor & ValidationSummary()?
This is built in helper which will help you to display the validation error message for the individual property / single property have following syntax –
VadlidationMessageFor(Model property lamda)
Where as validationsummary (bool) is helper which will help you to display the common validation errors, errors those do not belong to any model property. It has true/false value, when true it will display only common errors, when false will display all the validation errors.
Step 2: Server Side Validation
To implement server side validation Data Annotation API Provides you a built in object called ModelState, it has a property called IsValid. IsValid is boolean property, it will true if their is no validation error , otherwise it will be false based on that you can make the decision weather their is validation error or not.
Beside IsValid property model state also provides you a method AddModelError(key/property,”Error”) to add the custom error in model state object.
You can do the server side validation in post version of create action as follows –
[HttpPost]
public ActionResult Create(Customer rec)
{
//server side validation
if (ModelState.IsValid)
{
//no validation error
//save code.
return RedirectToAction("Index");
}
return View(rec);
}
Step 3: Client Side Validation
For client side validation you do not need to worry here since data annotation api is integrated with JQuery Validate plugin of jquery so you need to add the reference of following js files in View , it will do the client side validation.
<script src="~/Scripts/jquery-3.4.1.js"></script>
<script src="~/Scripts/jquery.validate.js"></script>
<script src="~/Scripts/jquery.validate.unobtrusive.js"></script>