How to Create Partial View in ASP.NET MVC 5?
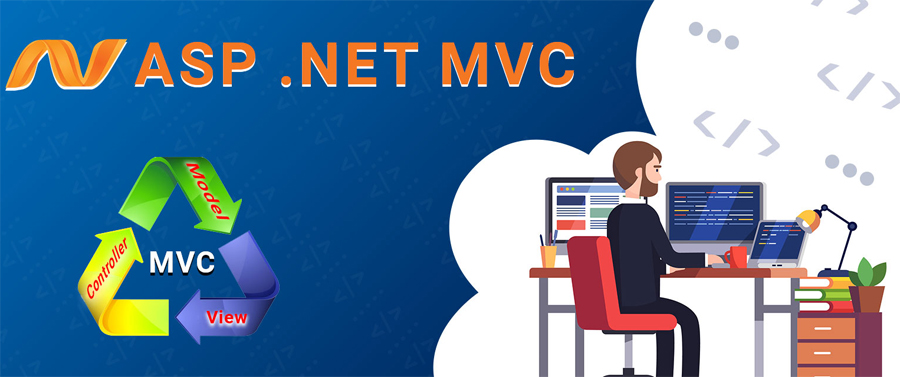
Example Source Code: https://drive.google.com/file/d/1dkgkEq9WR1b1hlDks-pEy8Fx6YBdEEgX/view?usp=sharing
Partial view is view that can be reused or shared in another view. A partial view is a reusable portion of a web page. It is .cshtml or .vbhtml file that contains HTML/CSS/JAvaScript or server side code just like any other view. It can be used in one or more Views or Layout Views. You can use the same partial view at multiple places and eliminates the redundant code.
You can create partial view just like any normal view by selecting checkbox Create as a partial view which can have all the things that view do have.
To render the partial view in any view we can use the following helpr methods –
Partial(“viewname”,[model]): – This method will help you to render the partial view directly inside a view whoes name is passed as a parameter. If the partial view is strongly typed then you can also pass the model object as the parameter.
RenderPartial(“viewname”,[Model]):- This method will help you to render the partial view in a view whoes name is passed as parameter, same way you can pass a model object as a parameter to strongly typed view.
Difference between Partial and RenderPartial Method is that Partial method return MvcHtmlString so it can be called directly in a view and can be stored in another variable but that is not possible with RenderPartial since it returns void so we can not store it any variable and we need to call it in block.
Let us implement simple partial view step by step –
Step 1: Create a ASP.NET MVC Application.
Step 2: To create a partial view, right-click on view -> shared folder and select Add -> View option. In this way we can add a partial view with name _Common with following code.
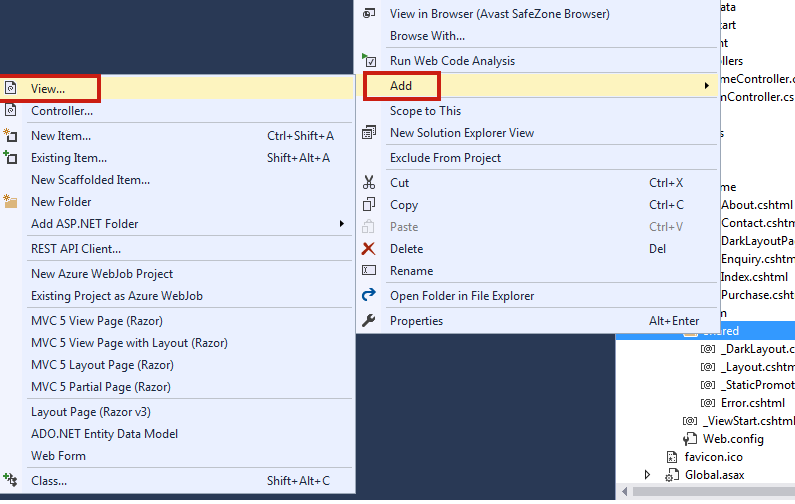
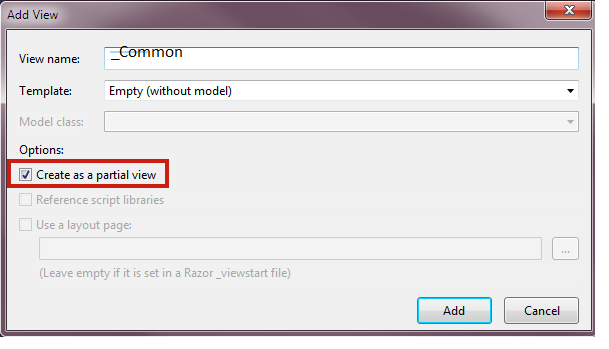
It is not mandatory to create a partial view in a shared folder but a partial view is mostly used as a reusable component, it is a good practice to put it in the “shared” folder.
<div style="background-color:yellow;color:red;width:300px;">
<h4> Partial View </h4>
<p> this is partial view used to demo</p>
</div>
Now Create a test controller and Index Action with Index View and Call this partial view in it using Partial or RenderPartial Method.
public class TestController : Controller
{
// GET: Test
public ActionResult Index()
{
return View();
}
}
Index.cshtml will have the following code.
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<div>
<h1> Test Index </h1>
@Html.Partial("_Common")
<h3>Call the common </h3>
@{ Html.RenderPartial("_Common"); }
</div>
</body>
</html>
Create a strongly typed partial view step by step as follows –
In the same application we can create the customer model to display single record of customer using partial view as follows –
public class Customer
{
public Int64 CustomerID { get; set; }
public string CustomerName { get; set; }
public string Address { get; set; }
public string EmailID { get; set; }
public decimal CreditLimit { get; set; }
}
Now create a partial view with a name _Customer by right click on shared folder and add view option by checking the create as a partial view as follows –
@model PartialViewExampleChildActionCalls.Models.Customer
<table border="1">
<tr>
<td>@Html.LabelFor(p=>p.CustomerID)</td>
<td>@Html.DisplayFor(p=>p.CustomerID)</td>
</tr>
<tr>
<td>@Html.LabelFor(p=>p.CustomerName)</td>
<td>@Html.DisplayFor(p=>p.CustomerName)</td>
</tr>
<tr>
<td>@Html.LabelFor(p=>p.Address)</td>
<td>@Html.DisplayFor(p => p.Address)</td>
</tr>
<tr>
<td>@Html.LabelFor(p=>p.EmailID)</td>
<td>@Html.DisplayFor(p=>p.EmailID)</td>
</tr>
<tr>
<td>@Html.LabelFor(p=>p.CreditLimit)</td>
<td>@Html.DisplayFor(p=>p.CreditLimit)</td>
</tr>
</table>
Create a test controller and index action with index.cshtml file which is connected to single object of the customer as follows –
public class TestController : Controller
{
// GET: Test
public ActionResult Index()
{
Customer c = new Customer() { CustomerID = 1, CustomerName = "Ganesh", Address = "Nigdi", CreditLimit = 45000, EmailID = "Ganesh@gmail.com" };
return View(c);
}
}
Index.cshtml View Code as follows –
@model PartialViewExampleChildActionCalls.Models.Customer
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<div>
<h3>Call Customer</h3>
@Html.Partial("_Customer",Model)
<h3>Call Customer</h3>
@{ Html.RenderPartial("_Customer",Model); }
</div>
</body>
</html>